How to Replace All Instances of a String in JavaScript
-
Use the
String.prototype.replaceAll()
Built-In JavaScript Function to Replace All Occurences of a String -
Use a Regular Expression With the
g
Flag to Replace All Occurences of a String in JavaScript -
Use
split()
andjoin()
for Older Browsers or for Compatibility Reasons
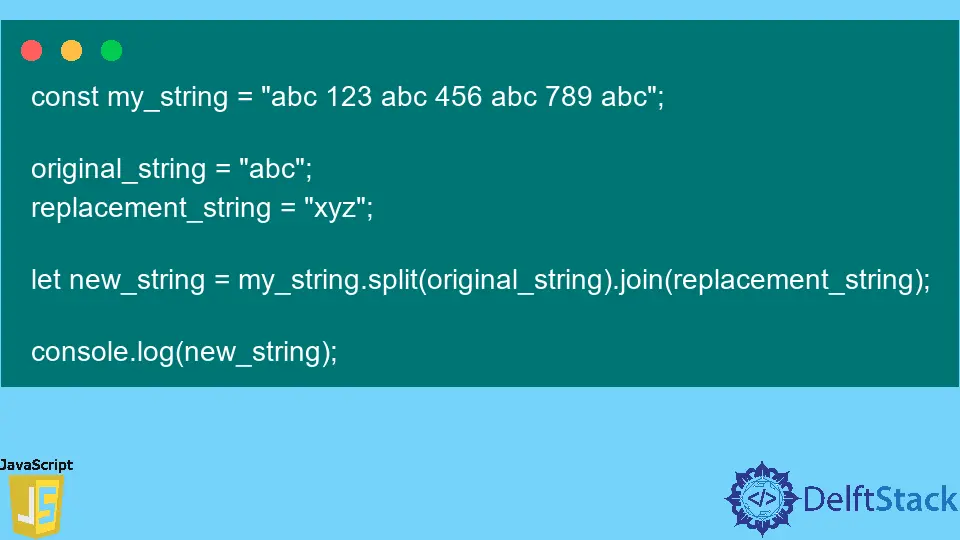
JavaScript allows you to replace all occurrences of a character or a substring in a string using a couple of methods.
Not all methods are equal in terms of speed and resource utilization, so it’s important to clearly define your use case before deciding on the best method. Additionally, the best solution depends on the browsers you are targeting - or, more exactly, the browser versions.
Hence, older browsers may not be able to understand newly-introduced JavaScript functionality. For example, the replaceAll
method is the easiest and most recommended option, but it won’t work with any Internet Explorer version. Thankfully, there are other ways you can achieve the same result in older browsers, as detailed below.
Use the String.prototype.replaceAll()
Built-In JavaScript Function to Replace All Occurences of a String
It is by far the most straightforward solution available in JavaScript, especially since it is part of the standard library. You do not need to create your own function from scratch, and this method is also considerably faster than most other implementations.
const my_string = 'abc 123 abc 456 abc 789 abc';
console.log(my_string.replaceAll('abc', 'xyz'));
Output:
"xyz 123 xyz 456 xyz 789 xyz"
Pass the Replacement String as a Variable With the String.prototype.replaceAll()
Method
The above example requires you to manually enter both the original string and the replacement as function arguments. In case you want to pass the replacement string as a variable, you can use the following method instead:
const my_string = 'abc 123 abc 456 abc 789 abc';
let rep_string = 'xyz';
console.log(my_string.replaceAll('abc', rep_string));
Output:
"xyz 123 xyz 456 xyz 789 xyz"
In fact, you can pass both arguments as variables as well. As you might expect, all you need to do is create another variable to store the substring you want to replace and then pass it as the first argument of the replaceAll
method.
const my_string = 'abc 123 abc 456 abc 789 abc';
let substring = 'abc';
let rep_string = 'xyz';
console.log(my_string.replaceAll(substring, rep_string));
Output:
"xyz 123 xyz 456 xyz 789 xyz"
Use a Regular Expression With the g
Flag to Replace All Occurences of a String in JavaScript
Another way you can achieve the same result is by using a regular expression with the g
flag and the replace()
method. The downside of using this method is that it can be slower, so try to avoid this if execution speed is a priority in your application.
The g
flag stands for global
, and without it the code will throw a TypeError
if you try to execute it. It is a requirement when working with regular expression objects and the replace()
method.
const my_string = 'abc 123 abc 456 abc 789 abc';
let new_string = my_string.replace(/abc/g, 'xyz');
console.log(new_string)
Output:
"xyz 123 xyz 456 xyz 789 xyz"
Use split()
and join()
for Older Browsers or for Compatibility Reasons
As mentioned above, older browsers may not understand new JavaScript functionality, as is the case with the replaceAll()
method. You can achieve the same results in those cases by splitting and joining your string instead.
Remember that this is a pretty bad solution in terms of optimization, so avoid using this method if your code is not meant to be compatible with older software.
const my_string = 'abc 123 abc 456 abc 789 abc';
let new_string = my_string.split('abc').join('xyz');
console.log(new_string);
Output:
"xyz 123 xyz 456 xyz 789 xyz"
It should be fairly obvious that the general solution involves using split()
for the string you want to search for and join()
for the string you want to replace it with. To make things even clearer, here is how you can pass the original and the replacement strings as variables instead of hard-coding them:
const my_string = 'abc 123 abc 456 abc 789 abc';
original_string = 'abc';
replacement_string = 'xyz';
let new_string = my_string.split(original_string).join(replacement_string);
console.log(new_string);
Output:
"xyz 123 xyz 456 xyz 789 xyz"