How to Replace Commas in a String in JavaScript
- Understanding the Replace Method
- Replacing All Commas with an Empty String
- Replacing Commas with Spaces
- Replacing Commas with Another Character
- Conclusion
- FAQ
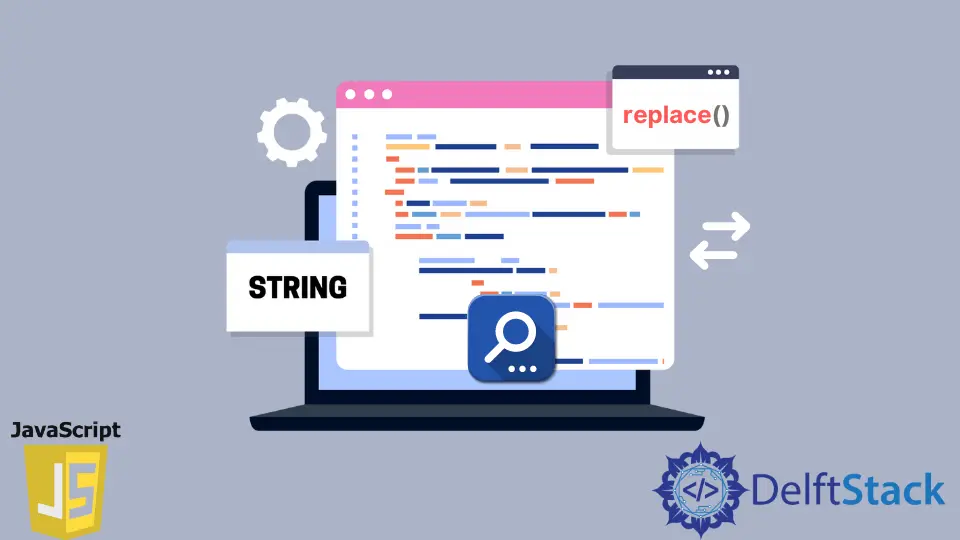
In the world of programming, manipulating strings is a common task. One frequent requirement is replacing specific characters within a string, such as commas. Whether you’re cleaning up user input or formatting data for display, knowing how to replace commas in a string using JavaScript is a valuable skill.
In this article, we will explore the replace
method and demonstrate how to replace all commas from a given string value with various examples. By the end of this guide, you will be equipped with the knowledge to handle string manipulation effectively in your JavaScript projects.
Understanding the Replace Method
The replace
method in JavaScript is a powerful tool for string manipulation. It allows you to search for a specified substring or pattern and replace it with a new substring. When dealing with commas, this method can be particularly useful. You can use it to remove commas, replace them with spaces, or even substitute them with other characters.
The basic syntax of the replace
method is:
string.replace(searchValue, newValue);
searchValue
: This can be a string or a regular expression that identifies the substring to be replaced.newValue
: This is the string that will replace the matched substring.
Let’s dive into some practical examples to see how this works in action.
Replacing All Commas with an Empty String
To remove all commas from a string, you can use the replace
method in combination with a regular expression. The regular expression /,/g
targets all commas in the string.
Here’s a simple example:
let originalString = "Hello, world, this, is, a, test.";
let modifiedString = originalString.replace(/,/g, "");
console.log(modifiedString);
Output:
Hello world this is a test.
In this example, the original string contains several commas. By using the regular expression /,/g
, we tell JavaScript to look for all occurrences of commas and replace them with an empty string. As a result, the modified string has no commas left, creating a cleaner output.
Replacing Commas with Spaces
Sometimes, you may want to replace commas with spaces instead of removing them entirely. This can help in maintaining the readability of the string while still eliminating the punctuation.
Here’s how to do it:
let originalString = "Apples, Oranges, Bananas";
let modifiedString = originalString.replace(/,/g, " ");
console.log(modifiedString);
Output:
Apples Oranges Bananas
In this code, we replace each comma with a space. The result is a string where the items are separated by a space instead of a comma. This method is particularly useful when dealing with lists where you want to improve readability without losing the information.
Replacing Commas with Another Character
You might have a scenario where you want to replace commas with a different character, such as a semicolon or a dash. This can be done easily using the replace
method.
Here’s an example where we replace commas with semicolons:
let originalString = "Red, Green, Blue";
let modifiedString = originalString.replace(/,/g, ";");
console.log(modifiedString);
Output:
Red; Green; Blue
In this case, we replace each comma with a semicolon. This technique can be used to format data for specific output requirements. By adjusting the newValue
in the replace
method, you can customize how your strings are presented.
Conclusion
Replacing commas in a string using JavaScript is a straightforward process, thanks to the replace
method. Whether you want to remove commas, replace them with spaces, or substitute them with other characters, JavaScript provides the flexibility you need. With the examples provided, you should now feel more confident in manipulating strings in your projects. Remember, string manipulation is a fundamental skill in programming, and mastering it can greatly enhance your coding capabilities.
FAQ
-
How do I replace only the first comma in a string?
You can use thereplace
method without the global flag. For example,originalString.replace(",", " ")
will only replace the first comma. -
Can I use the
replace
method on non-string types?
No, thereplace
method is specific to string objects. You must convert non-string types to strings first. -
What happens if there are no commas in the string?
If there are no commas in the string, thereplace
method will return the original string unchanged. -
Can I use
replace
with other characters besides commas?
Yes, you can use thereplace
method to replace any character or substring within a string. -
Is it possible to replace multiple different characters at once?
Yes, you can use a regular expression with the|
(pipe) operator to match multiple characters. For example,string.replace(/[,.]/g, " ")
will replace both commas and periods with a space.