How to Pretty Print JSON in JavaScript
- Understanding JSON Formatting
- Method 1: Using JSON.stringify()
- Method 2: Using Console.table()
- Method 3: Using a Third-Party Library
- Conclusion
- FAQ
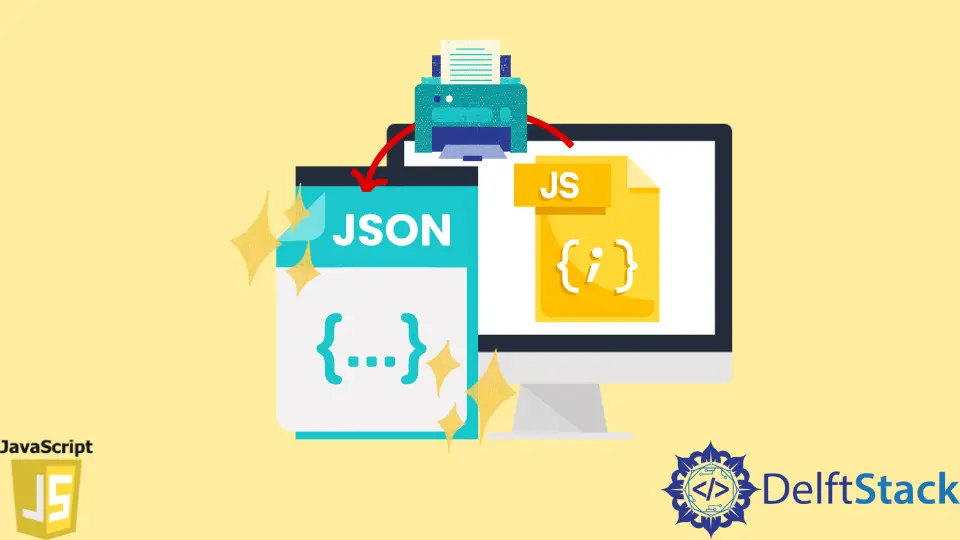
When working with JSON data in JavaScript, readability can often become a challenge. Whether you’re dealing with API responses, configuration files, or data storage, a well-formatted JSON structure is essential for effective debugging and comprehension.
This article will guide you through various methods to pretty print JSON in JavaScript, ensuring that you can easily visualize and understand your data. By the end of this guide, you’ll be equipped with the necessary techniques to format your JSON output neatly and efficiently.
Understanding JSON Formatting
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write. However, when JSON data is generated programmatically, it can often appear as a single line of text, making it difficult to interpret. Pretty printing is the process of formatting this data into a more readable structure, often involving indentation and line breaks.
The good news is that JavaScript provides built-in methods to accomplish this. Let’s dive into the various methods available for pretty printing JSON in JavaScript.
Method 1: Using JSON.stringify()
One of the most straightforward ways to pretty print JSON in JavaScript is by using the JSON.stringify()
method. This method converts a JavaScript object into a JSON string, and it can also take additional parameters to format the output.
Here’s how you can do it:
const jsonData = {
name: "John Doe",
age: 30,
city: "New York",
hobbies: ["reading", "traveling", "coding"]
};
const prettyJson = JSON.stringify(jsonData, null, 4);
console.log(prettyJson);
Output:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"hobbies": [
"reading",
"traveling",
"coding"
]
}
In this example, JSON.stringify()
takes three parameters: the object to convert, a replacer (which we set to null
for now), and the number of spaces to use for indentation (in this case, 4). The result is a neatly formatted JSON string that is easy to read. This method is particularly useful when you’re logging or displaying JSON data in a user interface.
Method 2: Using Console.table()
Another method to visually represent JSON data is by using console.table()
. While this method does not output a traditional JSON format, it presents the data in a tabular format, which can be easier to digest, especially for arrays of objects.
Here’s an example:
const jsonData = [
{ name: "John Doe", age: 30, city: "New York" },
{ name: "Jane Smith", age: 25, city: "Los Angeles" },
{ name: "Mike Johnson", age: 35, city: "Chicago" }
];
console.table(jsonData);
Output:
┌─────────┬─────────────┬─────┬──────────────┐
│ (index) │ name │ age │ city │
├─────────┼─────────────┼─────┼──────────────┤
│ 0 │ John Doe │ 30 │ New York │
│ 1 │ Jane Smith │ 25 │ Los Angeles │
│ 2 │ Mike Johnson │ 35 │ Chicago │
└─────────┴─────────────┴─────┴──────────────┘
Using console.table()
allows you to visualize your JSON data in a structured way. This method is particularly useful for debugging and analyzing data, as it clearly displays the relationships between different objects and their properties.
Method 3: Using a Third-Party Library
For more advanced formatting options, you can utilize third-party libraries like json-beautify
. This library allows you to customize the output beyond what JSON.stringify()
can offer. To use it, you’ll need to install the library first.
Here’s how to do it:
- Install the library using npm:
npm install json-beautify
- Use it in your JavaScript code:
const beautify = require('json-beautify');
const jsonData = {
name: "John Doe",
age: 30,
city: "New York",
hobbies: ["reading", "traveling", "coding"]
};
const prettyJson = beautify(jsonData, null, 4, 100);
console.log(prettyJson);
Output:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"hobbies": [
"reading",
"traveling",
"coding"
]
}
In this example, json-beautify
provides additional options, such as custom indentation levels and maximum line lengths. This flexibility can be particularly helpful when dealing with large JSON objects, allowing you to maintain readability without sacrificing detail.
Conclusion
Pretty printing JSON in JavaScript is a crucial skill that enhances the readability of your data, making it easier to debug and understand. Whether you choose to use the built-in JSON.stringify()
method, leverage the visual benefits of console.table()
, or opt for third-party libraries like json-beautify
, there are several effective ways to format your JSON output. By implementing these techniques, you can ensure that your JSON data is not only functional but also accessible and easy to work with.
FAQ
-
What is pretty printing in JSON?
Pretty printing is the process of formatting JSON data to make it more readable, typically by adding indentation and line breaks. -
Can I pretty print JSON directly in the browser console?
Yes, you can useJSON.stringify()
orconsole.table()
directly in the browser console to pretty print JSON. -
Are there any performance implications when pretty printing JSON?
Pretty printing can slightly affect performance due to additional processing for formatting, but it generally has a negligible impact for most use cases. -
Is there a limit to how much JSON I can pretty print?
The limit depends on the environment and the size of the data. Browsers and JavaScript engines typically handle large JSON objects, but very large datasets may require special handling. -
Can I customize the formatting of pretty printed JSON?
Yes, using libraries likejson-beautify
, you can customize indentation levels and other formatting options.