How to Convert String to Lower Case in JavaScript
-
Convert String to Lower Case in JavaScript Using
toLowerCase()
-
Convert String to Lower Case in JavaScript Using
toLocaleLowerCase()
-
Convert String to Lower Case With Our Custom Named Method
lower()
-
Convert Non-
String
Objects to Lower Case
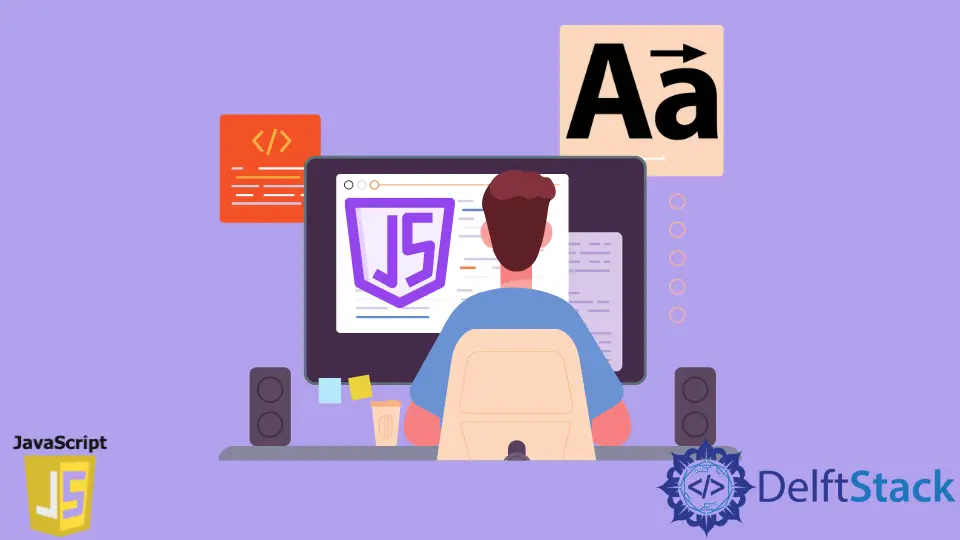
In this tutorial, we will learn how to convert a string to lower case in JavaScript.
JavaScript enables us to convert strings in many ways. In native JavaScript we can lower case the letters using the toLowerCase()
prototype method, or the toLocaleLowerCase()
prototype that is used to convert lower case considering the user/host locale.
Convert String to Lower Case in JavaScript Using toLowerCase()
Assume we have a string - My Awesome String
, and we want to convert it to lower case - my awesome string
. We can use the prototype method toLowerCase()
to lower case it. It creates a new string from the input string but with all lower case characters
Let’s see an example converting to lower case and printing to console using the console.log()
method.
var tempText = 'My Awesome String';
console.log(tempText.toLowerCase());
You can also add the prototype just after the single quote like the following example:
var TextConvertedToLowerCase = 'My Awesome String'.toLowerCase();
console.log(TextConvertedToLowerCase);
Convert String to Lower Case in JavaScript Using toLocaleLowerCase()
If the string contains locale-specific mappings like Turkish or German, we can use the toLocaleLowerCase()
method to convert the string to the lower case.
We can use toLocaleLowerCase()
without sending any arguments, similar to text.toLocaleLowerCase()
, or we can send the locale mapping as a parameter to that method, we can even send an array of locales and JavaScript will choose the best fit locale-mapping.
In the following example, we will convert an English string to lower case and convert a Turkish Latin Capital I
letter to lower case to show you how to send an array of locales to the function.
var demoText = 'My Awesome Second Demo Text';
console.log(demoText.toLocaleLowerCase());
console.log(demoText.toLocaleLowerCase('en-US'));
var localeArray = ['tr', 'TR'];
var LatinCapitalLetterIWithDotAboveInTurkish = '\u0130';
console.log(
LatinCapitalLetterIWithDotAboveInTurkish.toLocaleLowerCase(localeArray));
Output:
my awesome second demo text
my awesome second demo text
i
Convert String to Lower Case With Our Custom Named Method lower()
If we are not so familiar with JavaScript and want to change the syntax for toLowerCase()
to be similar to the one we use in our favorite languages like Python or PHP. In the following example, we will explain how to do so:
function lower(inputString) {
return String(inputString).toLowerCase();
}
var demoText = 'Our Awesome String To Lower Converter';
console.log(lower(demoText));
Convert Non- String
Objects to Lower Case
In case we want to convert the Date
to lower case, where the Date
is a non- String
object by nature, we can do it using the toLowerCase()
or toLocaleLowerCase()
methods as they both implemented to work generically with any value type.
Let’s see in the following example how to convert Date()
to lower case:
var normalDate = new Date();
var lowerCaseDate = new Date().toString().toLowerCase();
console.log('Normal Date Format > ' + normalDate);
console.log('Lower Case Date Format > ' + lowerCaseDate);
Output:
Normal Date Format > Thu Nov 12 2020 12:07:11 GMT+0000 (Coordinated Universal Time)
Lower Case Date Format > thu nov 12 2020 12:07:11 gmt+0000 (coordinated universal time)