Textbox Events in JavaScript
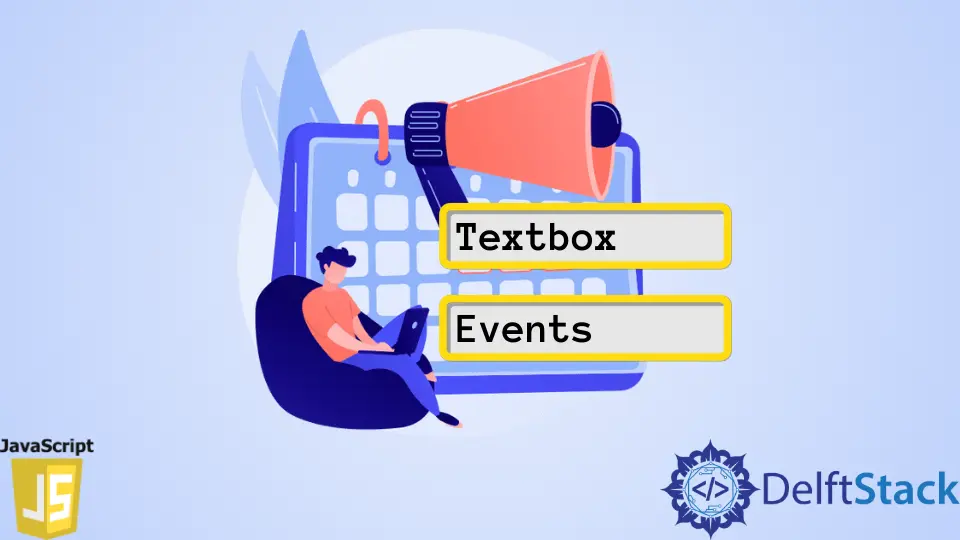
In JavaScript applications, the user interface is the part of the webpage where the user interacts. The texts we type in and the buttons we click combine to form a user interface.
In JavaScript, we have textbox events which are actions that happen in the browser. We trigger these events by entering the inputs and submitting the forms in the textbox.
We assign an event handler to respond to and handle these events in JavaScript. A user action on any form element is considered an event in JavaScript, including a mouse movement, mouse clicks, typing on the keyboard, etc.
An event handler is a JavaScript text that we associate with the event of the user interface element category. The most common way to create an event handler is to specify a function to execute first when an event occurs.
This article will discuss using an editable input’s oninput
and oninvalid
properties.
Textbox oninput
Event in JavaScript
The oninput
event happens when an element gets the user input. This event only occurs when the value of an <input>
or <textarea>
element is changed.
The following example code demonstrates the oninput
event to get input from the user.
<!DOCTYPE html>
<html>
<body>
<p>Using oninput event to get input.</p>
<p>Enter input to trigger the function.</p>
Enter the name: <input type="text" id="myinput" value="John">
<script>
document.getElementById("myinput").oninput = function() {myFunc()};
function myFunc() {
alert("The value of the input was changed.");
}
</script>
</body>
</html>
See demonstration and output of the code above in this link.
As we can see in the code, we assigned input value to Jon and created a function named myFunction()
. This function is called when changing the input or text area element.
So, when we run the code and change the input element, we will get a message: The value of the input field was changed
. By changing the input element, the oninput
event occurs.
Textbox oninvalid
Event in JavaScript
We have another textbox event in JavaScript called oninvalid
. This event occurs when a submittable input element entered by a user is invalid.
For example, when the user clicks on the submit button without filling it, the required attribute specifies that the input must be filled out before submitting the form. In this case, the oninvalid
event occurs.
Let’s understand this event using the below code example.
<!DOCTYPE html>
<html>
<body>
<form action="/action_page.php" method="get">
Name: <input type="text" oninvalid="alert('You must fill out the form!');" name="fname" required>
<input type="submit" value="Submit">
</form>
<p>If you click submit, without filling out the text field,
an alert message will occur.</p>
<p><strong>Note:</strong> The oninvalid event is not supported in Internet Explorer 9 and earlier.</p>
</body>
</html>
Click this link to see the working code above.
We specified a condition that says You must fill out the form
in the code, so the user must enter some input before clicking the submit button.
When we run the code, and the user clicks on the submit button without filling the input section, the oninvalid
event will be called, and an alert message will be displayed to the user. This is how we use oninvalid
textbox events using JavaScript.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn