How to Subtract Dates in JavaScript
-
Use the
getTime()
Function to Subtract Datetime in JavaScript -
Use the
Math.abs()
Function to Subtract Datetime in JavaScript -
Use
Date.UTC()
Function to Convert Dates to UTC
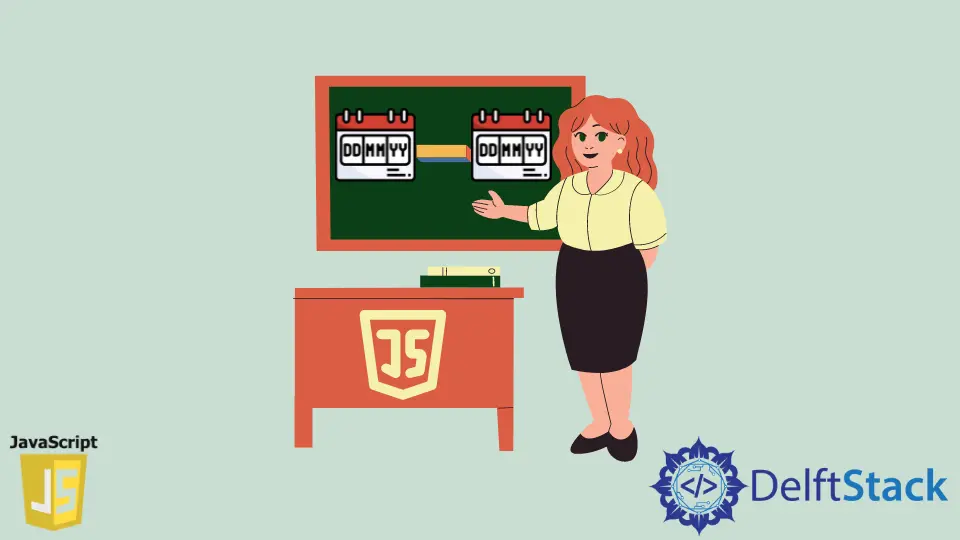
This article explains how to subtract two dates in JavaScript.
Use the getTime()
Function to Subtract Datetime in JavaScript
The first step would be to define your two dates using the in-built new Date()
function. To get the difference between them in days, subtract the two using the getTime()
function, which converts them to numeric values. You can print the result in days or convert it to hours, minutes, seconds, or milliseconds as required. Below is the example code:
var day1 = new Date('08/25/2020');
var day2 = new Date('12/25/2021');
var difference = day2.getTime() - day1.getTime();
document.write(difference);
Output:
42080400000
Use the Math.abs()
Function to Subtract Datetime in JavaScript
This procedure is similar to the first one except that it returns the absolute value. You will need to define the two dates then subtract the two variables using the Math.abs()
function as follows:
var day1 = new Date('08/25/2020');
var day2 = new Date('08/25/2021');
var difference = Math.abs(day2 - day1);
days = difference / (1000 * 3600 * 24)
console.log(days)
Output:
365
Note that the Math.abs()
function is case sensitive and will not work if written differently.
Use Date.UTC()
Function to Convert Dates to UTC
Where the dates in question span a daylight saving time change, the solutions above may prove a little problematic. The best way to get around this would be to convert the dates to UTC to get rid of the DST first then get the difference between them. We will need to create a function with two objects for the two dates i.e:
function difference(date1, date2) {
const date1utc =
Date.UTC(date1.getFullYear(), date1.getMonth(), date1.getDate());
const date2utc =
Date.UTC(date2.getFullYear(), date2.getMonth(), date2.getDate());
day = 1000 * 60 * 60 * 24;
return (date2utc - date1utc) / day
}
const date1 = new Date('2020-12-10'), date2 = new Date('2021-10-31'),
time_difference = difference(date1, date2)
console.log(time_difference)
Output:
325