How to Do String Interpolation in JavaScript
- Method 1: Template Literals
- Method 2: String Concatenation
-
Method 3: Using the
String.format
Method (Custom Implementation) - Method 4: String Interpolation with External Libraries
- Conclusion
- FAQ
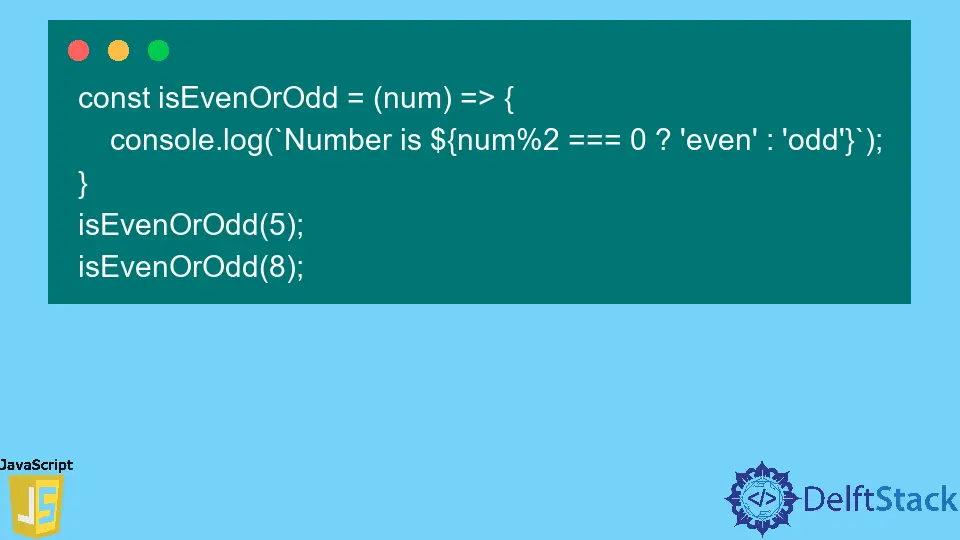
String interpolation in JavaScript is a powerful feature that allows developers to create dynamic strings with ease. Whether you’re working on a web application or a simple script, mastering string interpolation can save you time and make your code more readable.
In this article, we will explore various methods of string interpolation in JavaScript, including template literals, concatenation, and using libraries. Each method has its unique advantages and use cases, so let’s dive in and discover how to harness the power of string interpolation in your JavaScript projects.
Method 1: Template Literals
Template literals are a modern way to perform string interpolation in JavaScript. Introduced in ES6, they allow you to embed expressions directly within string literals using backticks. This method is not only concise but also enhances readability, making your code cleaner.
Here’s how you can use template literals:
const name = "Alice";
const age = 30;
const greeting = `Hello, my name is ${name} and I am ${age} years old.`;
console.log(greeting);
Output:
Hello, my name is Alice and I am 30 years old.
Using template literals, you can include variables and expressions directly in the string. The ${}
syntax lets you insert any JavaScript expression, including calculations or function calls. This flexibility makes template literals a preferred choice for many developers. Additionally, they support multi-line strings, allowing you to create formatted text easily. Overall, template literals are a powerful tool for string interpolation in modern JavaScript development.
Method 2: String Concatenation
Before the introduction of template literals, string concatenation was the primary method for combining strings in JavaScript. This involves using the +
operator to join strings and variables. While it may seem straightforward, concatenation can lead to less readable code, especially with multiple variables.
Here’s an example of string concatenation:
const name = "Alice";
const age = 30;
const greeting = "Hello, my name is " + name + " and I am " + age + " years old.";
console.log(greeting);
Output:
Hello, my name is Alice and I am 30 years old.
In this example, we concatenate the strings and variables using the +
operator. While this method works, it can quickly become cumbersome as the number of variables increases. Additionally, it can be harder to visualize the final output, especially when dealing with long strings. Despite its limitations, string concatenation is still widely used in legacy code and may be suitable for simpler scenarios where readability is not a concern.
Method 3: Using the String.format
Method (Custom Implementation)
Although JavaScript does not have a built-in String.format
method like Python, you can create a custom implementation to achieve similar functionality. This method allows you to define placeholders in a string and replace them with actual values.
Here’s a simple example of a custom format
function:
function formatString(template, ...values) {
return template.replace(/{(\d+)}/g, (match, index) => {
return typeof values[index] !== 'undefined' ? values[index] : match;
});
}
const name = "Alice";
const age = 30;
const greeting = formatString("Hello, my name is {0} and I am {1} years old.", name, age);
console.log(greeting);
Output:
Hello, my name is Alice and I am 30 years old.
In this code, we define a formatString
function that takes a template string and an arbitrary number of values. The function uses a regular expression to find placeholders in the format of {0}
, {1}
, etc., and replaces them with the corresponding values from the values
array. This approach provides flexibility and allows you to create dynamic strings without relying on the more verbose concatenation method. Although it requires a bit more setup, it can be beneficial for complex string formatting scenarios.
Method 4: String Interpolation with External Libraries
For developers looking for more advanced string interpolation features, several external libraries can provide enhanced functionality. Libraries like Handlebars or Mustache offer templating capabilities that can simplify string interpolation, especially in larger applications.
Here’s an example using Handlebars:
const Handlebars = require('handlebars');
const template = Handlebars.compile("Hello, my name is {{name}} and I am {{age}} years old.");
const data = { name: "Alice", age: 30 };
const greeting = template(data);
console.log(greeting);
Output:
Hello, my name is Alice and I am 30 years old.
In this example, we use Handlebars to compile a template string that includes placeholders for name
and age
. By providing a data object, Handlebars replaces the placeholders with the corresponding values. This method is particularly useful when dealing with complex data structures or when you need to generate dynamic HTML content. While using an external library adds an additional dependency to your project, it can significantly enhance your string interpolation capabilities and improve code maintainability.
Conclusion
String interpolation is an essential skill for any JavaScript developer. Whether you choose to use template literals, string concatenation, custom formatting functions, or external libraries, understanding these methods will enable you to write cleaner and more efficient code. As you continue to work on JavaScript projects, experiment with these techniques to find the best fit for your needs. With practice, you’ll become proficient in string interpolation, enhancing both your coding skills and the quality of your applications.
FAQ
-
What is string interpolation in JavaScript?
String interpolation is a method used to create dynamic strings by embedding variables and expressions directly within string literals. -
Why should I use template literals?
Template literals provide a more readable and concise way to perform string interpolation compared to traditional concatenation. -
Can I use multiple variables in string interpolation?
Yes, you can include multiple variables and expressions in a single string using template literals or custom formatting functions. -
Are there any libraries for string interpolation?
Yes, libraries like Handlebars and Mustache offer advanced templating features for string interpolation and can be beneficial in larger applications. -
Is string concatenation still relevant?
While string concatenation is less popular due to the introduction of template literals, it is still used in legacy code and simpler scenarios.