JavaScript Equivalent to Printf or String.Format
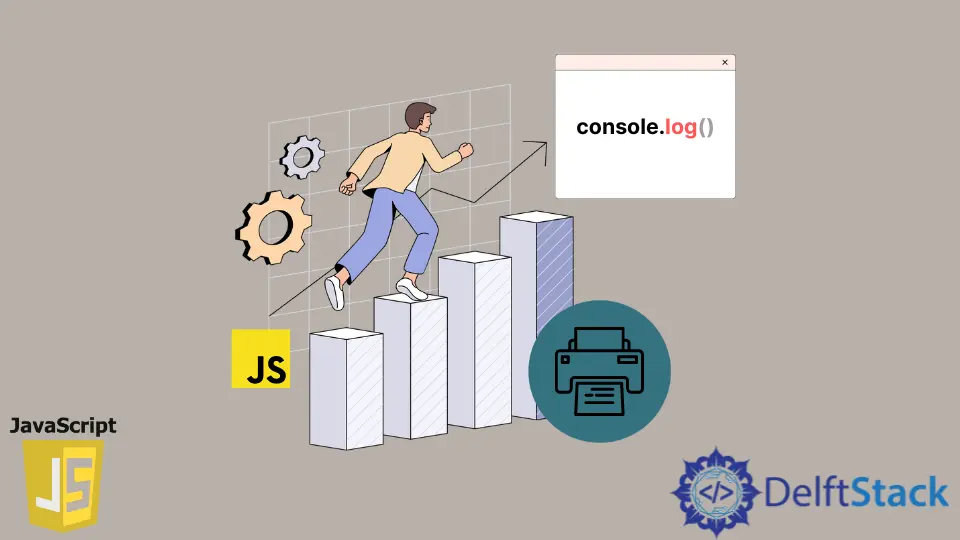
This article will describe the alternatives of printf
or String.Format
in JavaScript.
printf
is a function that we use in most programming languages like C
, PHP
, and Java
. This standard output function allows you to print a string or a statement on the console.
But in JavaScript, we cannot use printf
, so we need its alternatives, which we will be discussing here. Apart from that, we sometimes use the format specifiers to customize the output format.
Using console.log()
in JavaScript
One option here is to use console.log
to print something on the console. This was made available after the ES6 update, and its working is quite familiar with the working of printf
.
console.log
is very easy and is certainly the most common among developers. Let us look at the following code segment to understand the working of console.log
in JavaScript.
function example() {
let i = 5;
console.log(i);
}
example();
We declared a variable using the let
keyword in this code segment and printed it on the console. So, we use console.log(i)
to retrieve the value of variable i
and print it on the console screen.
If we want to print some string or some random text, we can use console.log
in the following way:
function example() {
console.log('Hello');
}
example();
In this code segment, as you can see, we used the console.log
and entered the text we wanted to print within the '
. It is how to print normal text items using console.log
in JavaScript.
This shows that console.log
is the most simple and easy alternative to printf
. But if you want to customize the format of the output, then we can create a custom prototype to create the String.Format
functionality.
We can see the following code segment as an example:
String.prototype.format = function() {
var args = arguments;
return this.replace(/{(\d+)}/g, function(match, number) {
return typeof args[number] != 'undefined' ? args[number] : match;
});
};
console.log(
'{0} was used as placeholder, furthermore {1} is used as the second argument {0} {2}'
.format('C language', 'C sharp'));
We have created a custom prototype function attached to each JavaScript string object in this code segment. What the format function does is that it takes the string and looks for {}
and replaces the number inside of it with the argument provided at that index.
So {0}
gets replaced with C language
and {1}
gets replaced by C sharp
. But {2}
remains as it is as we have provided no argument to take place for the {2}
placeholder.
And if you provide the third argument, the bracket {2}
gets replaced with the third argument. It might have helped you understand the custom String.prototype.format
function.