How to Sanitize String in JavaScript
-
Use the
sanitize()
Method to Sanitize String in JavaScript -
Use the
sanitize()
Method of DomPurify to Sanitize String in JavaScript
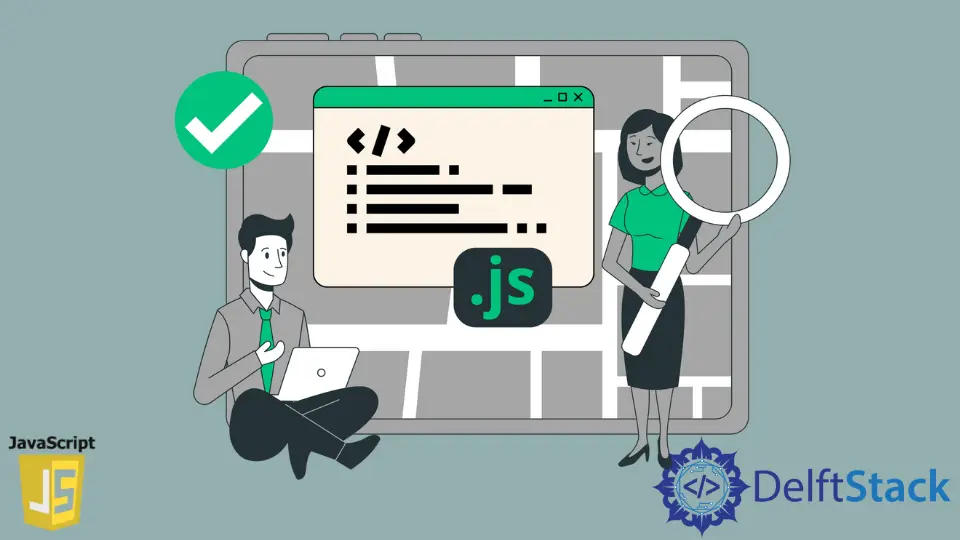
In this article, sanitization will be discussed in detail. Packages used to sanitize data in HTML or prevent XSS attacks are also explained in detail.
Use the sanitize()
Method to Sanitize String in JavaScript
Web applications frequently require HTML input. However, presenting them safely on a web page is difficult because they are subject to cross-site scripting (XSS) assaults.
The sanitize()
method of the Sanitizer interface sanitizes a tree of DOM nodes, removing unwanted elements or attributes. It should be used when the data is already available as DOM nodes, such as when sanitizing a Document
instance in a frame.
The default sanitizer()
configuration automatically strips out XSS-relevant input, including script tags, custom elements, and comments. This configuration may be customized using the sanitizer()
constructor options.
Syntax:
sanitize(input)
Parameter:
input
: It can be aDocumentFragment
or aDocument
to be sanitized.
Return value:
- The return value is a sanitized
DocumentFragment
.
We will now sanitize data from an iframe
with id username
.
Example:
const sanitizer = new Sanitizer(); // Default sanitizer;
// Get the frame and its Document object
const frame_element = document.getElementById('username')
const unsanitized_frame_tree = frame_element.contentWindow.document;
// Sanitize the document tree and update the frame.
const sanitized_frame_tree = sanitizer.sanitize(unsanitized_frame_tree);
frame_element.replaceChildren(sanitized_frame_tree);
Use the sanitize()
Method of DomPurify to Sanitize String in JavaScript
DOMPurify is a DOM-only XSS sanitizer for HTML, MathML, and SVG that is super-fast and over-tolerant. It is also effortless to use and get started.
DOMPurify is built in JavaScript and is compatible with all contemporary browsers (Safari (10+), Opera (15+), Internet Explorer (10+), Edge, Firefox, Chrome, etc., that use Blink or WebKit). It is not affected by MSIE6 or other older browsers, and it either utilizes a fallback or does nothing at all.
Automated tests now cover 19 distinct browsers, with more to follow. In addition, Node.js v14.15.1, v15.4.0, v16.13.0, v17.0.0, and older versions are also covered when executing DOMPurify on JSDOM.
Security experts created DOMPurify with extensive experience in online assaults and XSS.
What DOMPurify Does
DOMPurify cleans up HTML and protects against XSS attacks. You can feed DOMPurify a string containing filthy HTML, and it will return a string containing clean HTML (unless otherwise set).
DOMPurify will remove anything that includes harmful HTML. It’s also relatively quick.
It takes the browser’s technology and converts them into an XSS filter, so DOMPurify will be faster if your browser is faster. Just include DOMPurify on your website to start using it.
Use the Un-Minified Development Version
<script type="text/javascript" src="src/pure.js"></script>
Use the Minified and Tested Production Version
<script type="text/javascript" src="dist/pure.min.js"></script>
Following that, you may sanitize strings by running the following code:
let clean = DOMPurify.sanitize(dirty);
The generated HTML may be written into a DOM element using innerHTML
or directly into the DOM with document.write().
That is all up to you.
It’s worth noting that it allows HTML, SVG, and MathML by default. If all you need is HTML, which is a frequent use-case, you can easily put it up as well:
let clean = DOMPurify.sanitize(dirty, {USE_PROFILES: {html: true}});
Please keep in mind that the sanitization benefits may be lost if you sanitize HTML first and then edit it. If you send the sanitized markup to another library after sanitization, make sure the library doesn’t interfere with the HTML on its own.
After sanitizing your markup, you can look at the property DOMPurify.removed
to see which elements and attributes were deleted.
You can load this script asynchronously if you’re using an AMD module loader like Require.js:
import DOMPurify from 'dompurify';
var clean = DOMPurify.sanitize(dirty);
DOMPurify may be used both server-side with Node.js and client-side with Browserify or equivalent translators. It is necessary to have Node.js 4.x or later.
DOMPurify is intended to support any version that has been marked as active. Simultaneously, it phases away support for any version marked as maintenance.
DOMPurify may not instantly break with all versions under maintenance, but it does cease running tests against specific earlier versions.
npm install dompurify
For JSDOM v10 or newer:
const createDOMPurify = require('dompurify');
const {JSDOM} = require('jsdom');
const window = new JSDOM('').window;
const DOMPurify = createDOMPurify(window);
const clean = DOMPurify.sanitize(dirty);
For JSDOM versions older than v10:
const createDOMPurify = require('dompurify');
const jsdom = require('jsdom').jsdom;
const window = jsdom('').defaultView;
const DOMPurify = createDOMPurify(window);
const clean = DOMPurify.sanitize(dirty);
What Is Supported by DOMPurify
DOMPurify, by default, supports CSS and HTML custom data attributes. It additionally supports the Shadow DOM and recursively sanitizes DOM templates.
It also sanitizes HTML for usage with the JQuery $()
and elm.html()
APIs without causing any issues.