How to Remove Property From Object in JavaScript
-
Use the
delete
Operator to Remove a Property From an Object in JavaScript -
Use
underscore
Library to Remove a Property From an Object in JavaScript -
Use the
spread
Syntax to Remove a Property From an Object in JavaScript ECMAScript 6
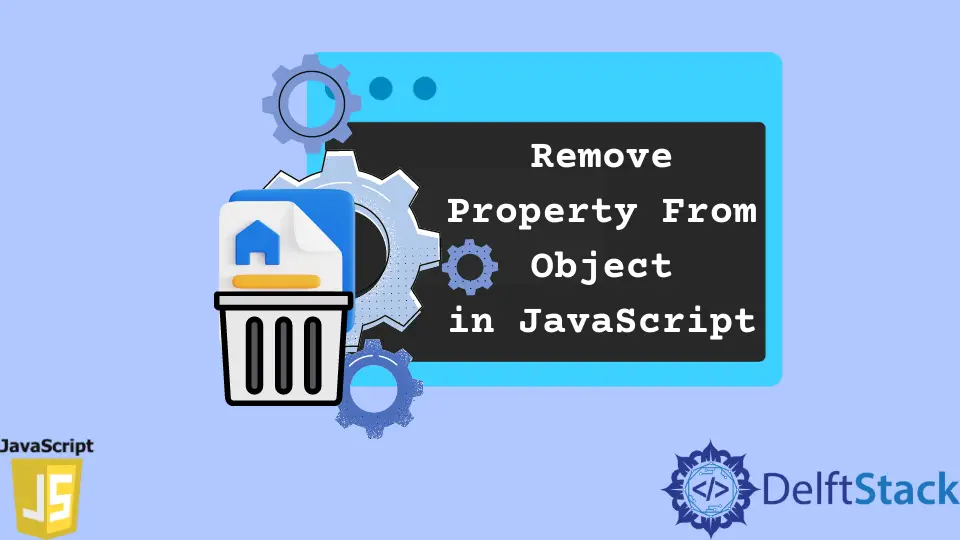
This tutorial will explain how we can remove a property from an object in JavaScript with multiple methods. We will demonstrate using the delete
operator, the reassignment
mechanism, the underscore
library, and the spread
syntax in ECMAScript 6.
Use the delete
Operator to Remove a Property From an Object in JavaScript
One method to remove any of the properties of any object is the delete
operator. This operator removes the property from the object. For example, we have an object myObject
with the properties as id
, subject
, and grade
, and we need to remove the grade
property.
var myObject = {'id': '12345', 'subject': 'programming', 'grade': 'A'};
console.log('Original object:', myObject);
// delete the grade property
delete myObject.grade
console.log('Updated object: ', myObject)
Output:
Original object: {id: "12345", subject: "programming", grade: "A"}
Updated object: {id: "12345", subject: "programming"}
Use underscore
Library to Remove a Property From an Object in JavaScript
One of the libraries that can help in removing a property from an object in JavaScript but without updating the original object is the underscore
library. That library provides two important functions _.pick()
and _.omit()
.
The _.omit()
function could remove the given property from the object and return an updated copy.
var myObject = {'id': '12345', 'subject': 'programming', 'grade': 'A'};
console.log('Original object:', myObject);
var updatedObject = _.omit(myObject, 'grade')
console.log('Updated object with omit() : ', updatedObject)
console.log('Original object after update:', myObject);
Output:
Original object: {id: "12345", subject: "programming", grade: "A"}
Updated object with omit() : {id: "12345", subject: "programming"}
Original object after update: {id: "12345", subject: "programming", grade: "A"}
The _.pick()
function includes only the object’s given properties and neglects all other properties, and returns an updated copy from that object.
var myObject = {'id': '12345', 'subject': 'programming', 'grade': 'A'};
console.log('Original object:', myObject);
var updatedObject = _.pick(myObject, 'id', 'subject')
console.log('Updated object with pick() : ', updatedObject)
console.log('Original object after update:', myObject);
Output:
Original object: {id: "12345", subject: "programming", grade: "A"}
Updated object with pick() : {id: "12345", subject: "programming"}
Original object after update: {id: "12345", subject: "programming", grade: "A"}
We can import the library from here.
Use the spread
Syntax to Remove a Property From an Object in JavaScript ECMAScript 6
The JavaScript spread
syntax in ECMAScript 6 provides a very elegant way to remove the given properties from JavaScript Object without changing the original object but return the newly updated object.
When we use the spread
syntax, it will look as if we were separating the original object into two variables. One of them will be the removed property. The other variable will hold a copy of the original object without that removed object.
var myObject = {'id': '12345', 'subject': 'programming', 'grade': 'A'};
console.log('Original object:', myObject);
var {grade, ...myUpdatedObject} = myObject;
console.log('The removed \'grade\' property : ', grade)
console.log('Updated object : ', myUpdatedObject)
console.log('Original object after update:', myObject);
Output:
Original object: {id: "12345", subject: "programming", grade: "A"}
The removed 'grade' property : A
Updated object : {id: "12345", subject: "programming"}
Original object after update: {id: "12345", subject: "programming", grade: "A"}