How to Remove Duplicates From an Array in JavaScript
-
Use
Set
to Remove Duplicates in JavaScript With ECMAScript 6spread
Syntax -
Use
Array.filter()
to Remove the Duplicates From JavaScript Array -
Use
hashtable
to Remove Duplicate From a Primitive Array in JavaScript -
Use
_.uniq()
Function Fromunderscore
andLoDash
Libraries to Remove Duplicate From Arrays in JavaScript
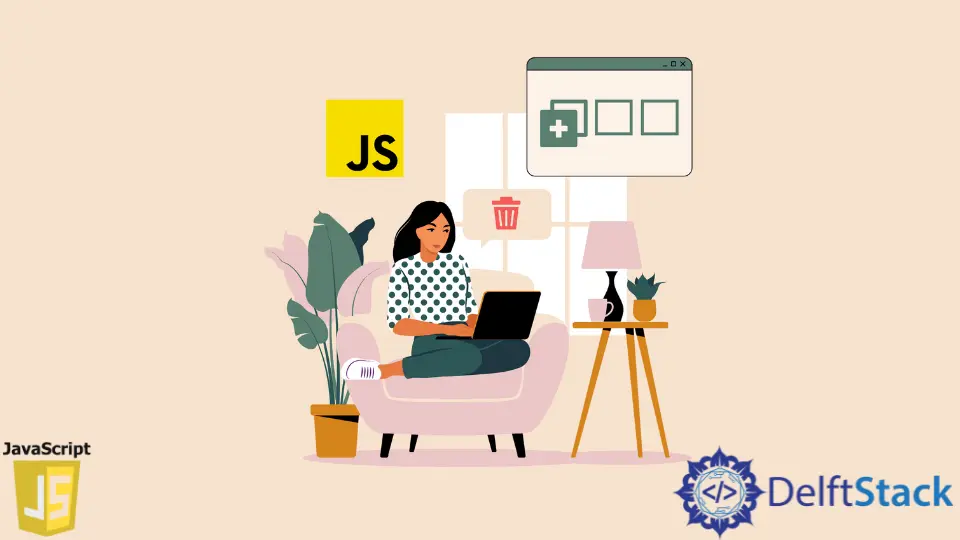
This tutorial will explain how we can remove duplicates from an array in JavaScript using different methods. One of the methods is to pass the target array to the constructor of the class Set
to return an equivalent non-duplicate array. Another way is to use the JavaScript Array’s filter()
method and implement the test condition in its callback function.
Use Set
to Remove Duplicates in JavaScript With ECMAScript 6 spread
Syntax
In Mathematics, the set contains a group of unique, non-duplicate elements. In JavaScript, the Set
class can get all those non-duplicate elements from an array.
With ECMAScript 6, we can use the power of the spread syntax by using the class Set
to get a new array of non-duplicates from that we send to the Set()
constructor.
var arr = [1, 2, 3, 4, 1, 2, 3, 1, 2, 3]
var uniqueArr = [...new Set(arr)]
console.log(uniqueArr)
Output:
[1, 2, 3, 4]
Use Array.filter()
to Remove the Duplicates From JavaScript Array
The JavaScript array introduced one of the higher-order functions called filter()
, which loops on each array element, applies a test condition on it, and only returns that element if it meets the condition. This test condition will be implemented inside the callback function, passed as an argument to the filter()
method.
We can remove the duplicates from the array by setting the test condition to check if the index of the current element in-loop is the first occurrence in that array. The filter()
function will take an extra argument pos
that will represent the array index of the element during execution.
var arrTwo = ['Hello 1 ', ' Hello 2 ', 'Hello 1 ', ' Hello 2 ', 'Hello 1 again']
const filteredArray = arrTwo.filter(function(ele, pos) {
return arrTwo.indexOf(ele) == pos;
})
console.log('The filtered array ', filteredArray);
Output:
The filtered array (3) ["Hello 1 ", " Hello 2 ", "Hello 1 again"]
If we can use JavaScript ECMAScript 6 arrow syntax, that removing duplicate operation be implemented in a better way.
var arrTwo =
['Hello 1 ', ' Hello 2 ', 'Hello 1 ', ' Hello 2 ', 'Hello 1 again'];
const filteredArray = arrTwo.filter((ele, pos) => arrTwo.indexOf(ele) == pos);
console.log('The filtered array', filteredArray);
Output:
The filtered array (3) ["Hello 1 ", " Hello 2 ", "Hello 1 again"]
Use hashtable
to Remove Duplicate From a Primitive Array in JavaScript
If we have an array only composed of only primitive types like numbers [ 1, 2, 3, 4, 1, 2, 3 ]
and we want to remove duplicates from that array [ 1, 2, 3, 4 ]
we can implement our filterArray()
function using hashtables
.
We will build a temporary object called found
that will include all non-duplicate values. Inside the filter
function, our condition will return false
if the element already exists in the found
object; otherwise, we will add that element to the found
object with the value true
;
var arrThree =
[
'Hello 1 ', ' Hello 2 ', ' Hello 2 ', 'Welcome', 'Hello 1 again',
'Welcome', 'Goodbye'
]
function filterArray(inputArr) {
var found = {};
var out = inputArr.filter(function(element) {
return found.hasOwnProperty(element) ? false : (found[element] = true);
});
return out;
}
const outputArray = filterArray(arrThree);
console.log('Original Array', arrThree);
console.log('Filtered Array', outputArray);
Output:
Original Array ["Hello 1 ", " Hello 2 ", " Hello 2 ", "Welcome", "Hello 1 again", "Welcome", "Goodbye"]
Filtered Array ["Hello 1 ", " Hello 2 ", "Welcome", "Hello 1 again", "Goodbye"]
Use _.uniq()
Function From underscore
and LoDash
Libraries to Remove Duplicate From Arrays in JavaScript
If we are already using any of the underscore
library methods, we can use the _.uniq()
method, as it returns only the first occurrence of the element from the input array.
var arrFive = [1, 2, 3, 1, 5, 2];
console.log('LoDash output', _.uniq(arrFive));
LoDash output [1, 2, 3, 5]
we can import the library from here.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript