How to Generate a Random Number Between Two Numbers in JavaScript
- Generate a Random Number Between 1 and User-Defined Value in JavaScript
- Generate a Random Number Between Two Numbers in JavaScript
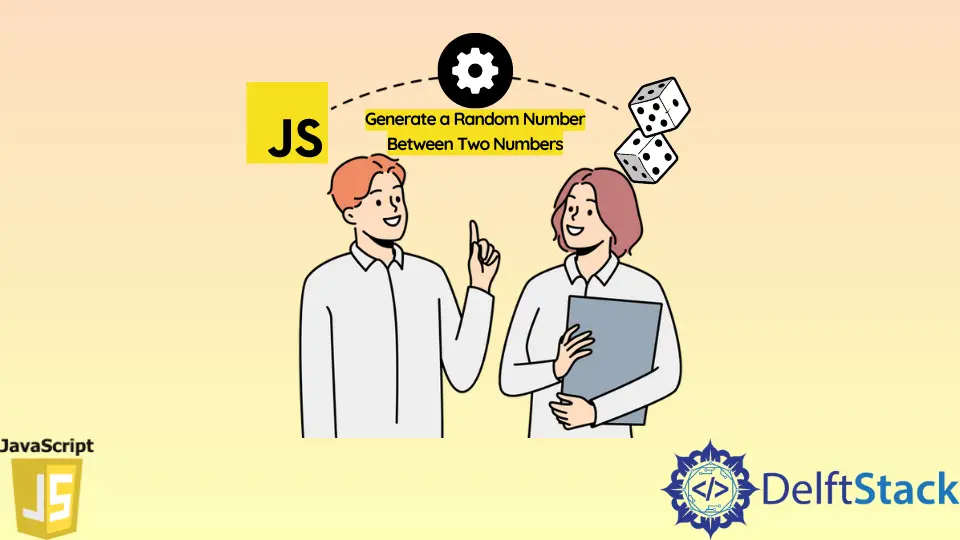
This tutorial will learn how to generate a random number between two numbers in JavaScript. We will use the method Math.random()
that generates a random floating number between 0.0
and 0.999
.
Generate a Random Number Between 1 and User-Defined Value in JavaScript
We can generate a random number in JavaScript using a random number generator - Math.random()
, by multiplying the generated float number with the maximum number we want to generate. Math.random()* max
generates a random number between 0 and max
.
function generateRandom(max) {
return Math.random() * max;
}
console.log('1st try: ' + generateRandom(5));
console.log('2nd try: ' + generateRandom(5));
console.log('3rd try: ' + generateRandom(5));
console.log('4th try: ' + generateRandom(5));
Output:
1st try: 3.3202340813091347
2nd try: 1.9796467067025836
3rd try: 3.297605748406279
4th try: 1.1006700756032417
If we want to get only the result in integer format, we can use Math.floor()
, this method returns the largest integer less than or equal to the input number.
console.log(Math.floor(1.02));
console.log(Math.floor(1.5));
console.log(Math.floor(1.9));
console.log(Math.floor(1));
Output:
1
1
1
1
By using the Math.floor()
method we can get our randomly generated integers,
function generateRandomInt(max) {
return Math.floor(Math.random() * max);
}
console.log('1st Integer try: ' + generateRandomInt(9));
console.log('2nd Integer try: ' + generateRandomInt(9));
console.log('3rd Integer try: ' + generateRandomInt(9));
console.log('4th Integer try: ' + generateRandomInt(9));
Output:
1st Integer try: 8
2nd Integer try: 5
3rd Integer try: 7
4th Integer try: 0
Generate a Random Number Between Two Numbers in JavaScript
If we also want to have a user-defined minimum value, we need to change the equation of Math.random() * max
to Math.random() * (max-min)) +min
. Using this equation the returned value is a random number between min
and max
.
function generateRandomInt(min, max) {
return Math.floor((Math.random() * (max - min)) + min);
}
console.log('1st min and max try: ' + generateRandomInt(2, 9));
console.log('2nd min and max try: ' + generateRandomInt(2, 9));
console.log('3rd min and max try: ' + generateRandomInt(2, 9));
console.log('4th min and max try: ' + generateRandomInt(2, 9));
Output:
1st min and max try: 6
2nd min and max try: 2
3rd min and max try: 8
4th min and max try: 3
In case we are interested in having the max
value to be included in our random generator scope, we need to change the equation to (Math.random() * (max+1 - min)) +min
. Adding 1 to max-min
will include the max in range and Math.floor()
will always guarantee that this value is not more than the max
.
function generateRandomInt(min, max) {
return Math.floor((Math.random() * (max + 1 - min)) + min);
}
console.log('1st min and max included try: ' + generateRandomInt(2, 9));
console.log('2nd min and max included try: ' + generateRandomInt(2, 9));
console.log('3rd min and max included try: ' + generateRandomInt(2, 9));
console.log('4th min and max included try: ' + generateRandomInt(2, 9));
Output:
1st min and max included try: 3
2nd min and max included try: 7
3rd min and max included try: 9
4th min and max included try: 6