Comment générer un nombre aléatoire entre deux nombres en JavaScript
- Générer un nombre aléatoire entre 1 et une valeur définie par l’utilisateur en JavaScript
- Générer un nombre aléatoire entre deux nombres en JavaScript
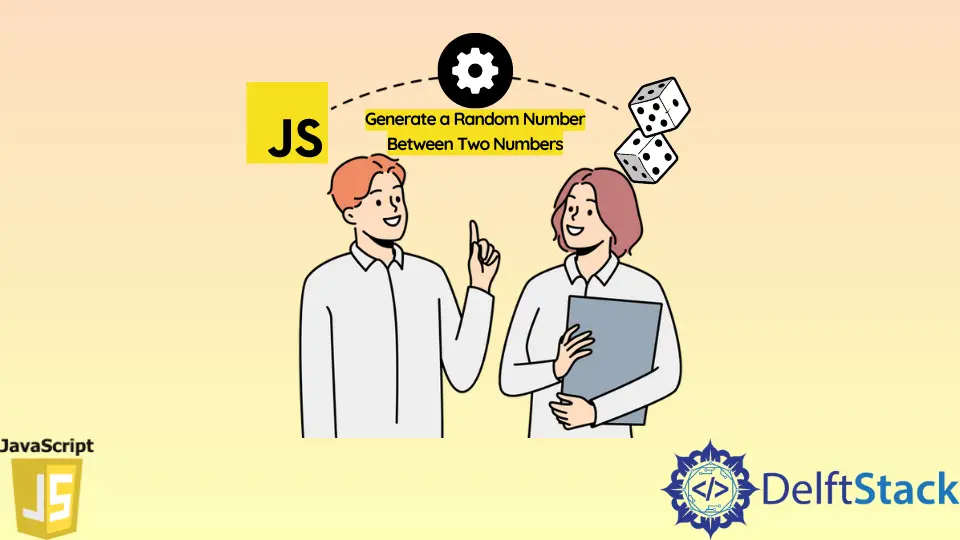
Ce tutoriel vous apprendra à générer un nombre aléatoire entre deux nombres en JavaScript. Nous utiliserons la méthode Math.random()
qui génère un nombre float aléatoire entre 0.0
et 0.999
.
Générer un nombre aléatoire entre 1 et une valeur définie par l’utilisateur en JavaScript
Nous pouvons générer un nombre aléatoire en JavaScript à l’aide d’un générateur de nombres aléatoires - Math.random()
, en multipliant le nombre de flotteurs généré par le nombre maximum que nous voulons générer. Math.random()* max
génère un nombre aléatoire entre 0 et max
.
function generateRandom(max) {
return Math.random() * max;
}
console.log('1st try: ' + generateRandom(5));
console.log('2nd try: ' + generateRandom(5));
console.log('3rd try: ' + generateRandom(5));
console.log('4th try: ' + generateRandom(5));
Production :
1st try: 3.3202340813091347
2nd try: 1.9796467067025836
3rd try: 3.297605748406279
4th try: 1.1006700756032417
Si nous voulons obtenir uniquement le résultat sous forme d’entier, nous pouvons utiliser Math.floor()
, cette méthode retourne le plus grand entier inférieur ou égal au nombre d’entrée.
console.log(Math.floor(1.02));
console.log(Math.floor(1.5));
console.log(Math.floor(1.9));
console.log(Math.floor(1));
Production :
1
1
1
1
En utilisant la méthode Math.floor()
, nous pouvons obtenir nos entiers générés aléatoirement,
function generateRandomInt(max) {
return Math.floor(Math.random() * max);
}
console.log('1st Integer try: ' + generateRandomInt(9));
console.log('2nd Integer try: ' + generateRandomInt(9));
console.log('3rd Integer try: ' + generateRandomInt(9));
console.log('4th Integer try: ' + generateRandomInt(9));
Production :
1st Integer try: 8
2nd Integer try: 5
3rd Integer try: 7
4th Integer try: 0
Générer un nombre aléatoire entre deux nombres en JavaScript
Si nous voulons également avoir une valeur minimale définie par l’utilisateur, nous devons changer l’équation de Math.random() * max
en Math.random() * (max-min)) +min
. En utilisant cette équation, la valeur retournée est un nombre aléatoire entre min
et max
.
function generateRandomInt(min, max) {
return Math.floor((Math.random() * (max - min)) + min);
}
console.log('1st min and max try: ' + generateRandomInt(2, 9));
console.log('2nd min and max try: ' + generateRandomInt(2, 9));
console.log('3rd min and max try: ' + generateRandomInt(2, 9));
console.log('4th min and max try: ' + generateRandomInt(2, 9));
Production :
1st min and max try: 6
2nd min and max try: 2
3rd min and max try: 8
4th min and max try: 3
Si nous souhaitons inclure la valeur max
dans notre générateur aléatoire, nous devons changer l’équation en Math.random() * (max+1 - min) + min
. L’ajout de 1 à max-min
inclura le max dans la plage et Math.floor()
garantira toujours que cette valeur n’est pas supérieure au max
.
function generateRandomInt(min, max) {
return Math.floor((Math.random() * (max + 1 - min)) + min);
}
console.log('1st min and max included try: ' + generateRandomInt(2, 9));
console.log('2nd min and max included try: ' + generateRandomInt(2, 9));
console.log('3rd min and max included try: ' + generateRandomInt(2, 9));
console.log('4th min and max included try: ' + generateRandomInt(2, 9));
Production :
1st min and max included try: 3
2nd min and max included try: 7
3rd min and max included try: 9
4th min and max included try: 6