How to Prepend Elements in JavaScript
-
Use the
prepend()
Method to Prepend Elements in JavaScript -
Use the
insertBefore()
Method to Prepend Elements in JavaScript -
Use the
insertAdjacentElement()
Method to Prepend Elements in JavaScript -
Use the
before()
Method to Prepend Elements in JavaScript
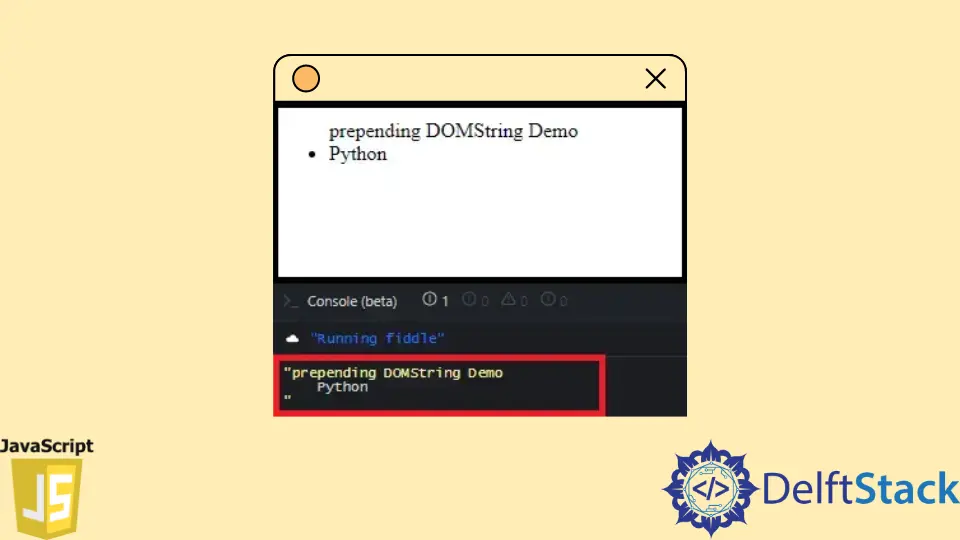
This article educates how to prepend elements in JavaScript. Prepend means inserting at the beginning of the specified element.
We can use prepend()
, insertBefore()
, before()
, and insertAdjacentElement()
methods to do that. Let’s learn them one by one.
Use the prepend()
Method to Prepend Elements in JavaScript
We can insert a DOMString
or a collection of Node
at the beginning of the specified element by using the prepend()
method, which means we can insert before an immediate first child of a parent element. See the code snippets given below.
HTML Code (This HTML would be the same throughout this tutorial):
<ul id="languages">
<li>Python</li>
</ul>
JavaScript Code:
let parentNode = document.querySelector('#languages');
let li = document.createElement('li');
li.textContent = 'Java';
parentNode.prepend(li);
Output:
Initially, we have one list item on our page: Python
. We use querySelector()
to select the first element that matches the particular selector and save it in parentNode
to prepend another list item.
Then, create a <li>
element using createElement()
and set the text content as Java
using .textContent
property.
Finally, we use the prepend()
method to insert the Java
before Python
(see the output above). Can we add multiple <li>
elements before the first child of an element?
Yes, of course. See the following code and observe the output.
JavaScript Code:
let parentNode = document.querySelector('#languages');
let languages = ['Java', 'JavaScript', 'TypeScript'];
let nodes = languages.map(lang => {
let li = document.createElement('li');
li.textContent = lang;
return li;
});
parentNode.prepend(...nodes);
Output:
This example also selects the first element that matches the given selector.
Further, we use the map()
method to call an arrow function for every element of the languages
array that creates the <li>
elements and use the .textContent
property to set the text content of <li>
with the current element of the array.
Remember, the map()
method neither calls a function for an empty element nor changes the actual array, which is languages
here.
Lastly, we pass nodes
having all list items using spread operator (...
) to the prepend()
method. The spread operator iterates over the array and splits it into individual elements.
Remember, the prepend()
method inserts the set of nodes at once before the parent’s first child. Let’s proceed with the following code to prepend the DOMString
using JavaScript.
JavaScript Code:
let parent = document.querySelector('#languages');
parent.prepend('prepending DOMString Demo');
console.log(parent.textContent);
Output:
You see, the DOMString
is inserted just before the first child (list item Python
) of the element.
By printing on the console, we see the list item Python
and DOMString
within double quotes because we use the .textContent
property here that returns the text content of the particular node and all its children.
Use the insertBefore()
Method to Prepend Elements in JavaScript
JavaScript Code:
let parentNode = document.querySelector('#languages');
let li = document.createElement('li');
li.textContent = 'Java';
parentNode.insertBefore(li, parentNode.firstChild);
Output:
Here, the inserBefore()
method takes the current element you want to insert and the first child of the parent element as parameters. Then, it inserts the current element before the first child of the parent element (see the above output).
Use the insertAdjacentElement()
Method to Prepend Elements in JavaScript
JavaScript Code:
let parentNode = document.querySelector('#languages');
let li = document.createElement('li');
li.textContent = 'Java';
parentNode.insertAdjacentElement('afterbegin', li);
Output:
This example uses the insertAdjacentElement()
method that inserts the specified element at the given position.
It has four properties: beforebegin
, afterbegin
, beforeend
, and afterend
, but we are using afterbegin
to insert after the parent element but before the first child.
See the following to visualize how these properties add the element. Remember, we take the element as a parent whose id is languages
.
<!-- beforebegin -->
<ul id="languages">
<!-- afterbegin -->
<li>Python</li>
<!-- beforeend -->
</ul>
<!-- afterend -->
We’ve seen how we can select the parent element and insert a new element before the first child of the parent element. Let’s explore how to select the child element itself and insert another element before itself.
Use the before()
Method to Prepend Elements in JavaScript
JavaScript Code:
let parentNode = document.querySelector('#languages');
let li = document.createElement('li');
li.textContent = 'Java';
parentNode.firstChild.before(li);
Output:
Here, we use the before()
method to prepend the elements. We can insert a DOMString
or a collection of node
just before the specified element of the parent.
Here, we can select any child, but we focus on inserting before the firstChild
. You can find more about before()
here.