How to Change Iframe Source in JavaScript
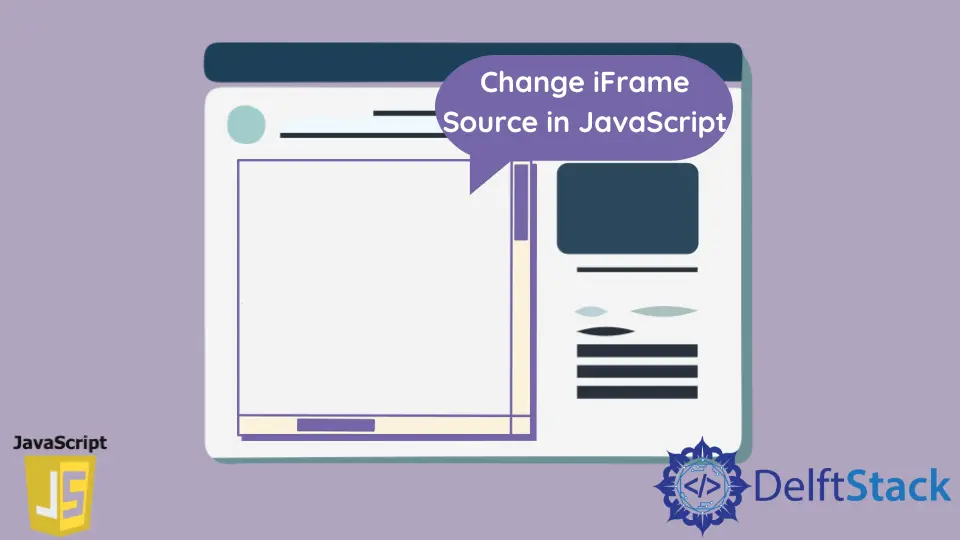
iframe
is an HTML element used to display a website within a web page with the help of a page URL or Path. Within the current HTML document, we can embed another document.
To specify the size of iframe
, we can use the "height"
and "width"
attributes separately or style attributes for CSS.
Syntax:
<iframe src="dummy_iframe.htm" height="200" width="300" title="Dummy Iframe"></iframe>
Change iframe
Source in JavaScript
The idea behind changing the iframe
source is to switch the web page in the display of iframe
. In JavaScript we can change the source or redefine it with the JavaScript default method getElementById()
.
We can re-assign new path or URL of webpage to getElementById("idOfElement").src
.
Syntax:
getElementById('idOfElement').src = 'newPath.html'
Example Code:
<!DOCTYPE html>
<html>
<body>
<h2>Change iframe source in JavaScript</h2>
<p>Click the chosen button to view the chosen page on the website in iframe:</p>
<button onclick="myFunction('https://www.delftstack.com/')">Click to display Home</button>
<button onclick="myFunction('https://www.delftstack.com/about-us/')">Click to display About</button>
<br></br>
<script type="text/javascript">
function myFunction(path) {
document.getElementById('myIframe').src = path;
}
</script>
<iframe id="myIframe" src="https://www.delftstack.com/" width="1000" height="450" frameborder="0" scrolling="no"></iframe>
</body>
</html>
In above source code we have created HTML iframe
block using <iframe></iframe>
tags with specified width and height. We have assigned an ID to the iframe
element, and the default src
attribute is assigned with the"https://www.delftstack.com/"
path.
We have declared myFunction()
, which will receive the path as an argument. In that function we have used document.getElementById()
which will receive html element id, and assign that passed path to the src
.
We have created multiple buttons for switching the iframe
source and called myFunction()
with the path on the click event of those buttons.
Alternative Way in JQuery
There is a default method attr()
in JQuery. We can use that method to set the attribute and value as well. As shown below, we can also use JQuery to reassign the iframe
source.
Syntax:
$('#idOfElement').attr('src', 'https://www.delftstack.com/');
Using the element id called default attr()
method and passed the attribute and value.