How to Create Comment Box in HTML and JavaScript
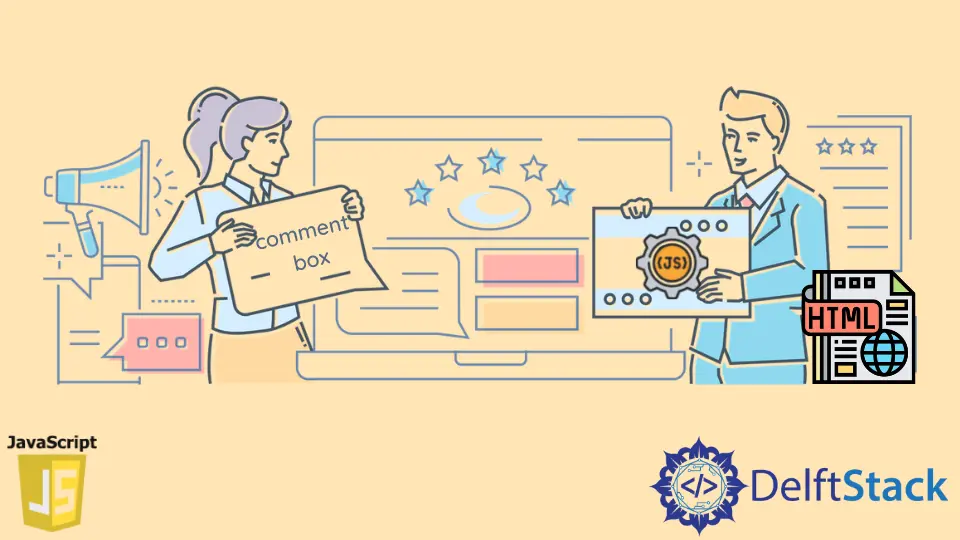
This tutorial is about creating a comment box using a form, getting data from user input in HTML, and displaying it using JavaScript events and functions.
Create Comment Box in HTML and JavaScript
In order to get comment and other user data from input fields we can create input forms as a comment box using default html <form></form>
and <textarea></textarea>
elements. We can define ids to that element and get the data of the value property of that element using ids.
To collect user input values, we used an HTML form element. Using the type attribute, we can display input elements differently.
Syntax:
<form>
<input type="text" id="inputId" name="username">
</form>
If there is a need to insert long text or multiple line texts, the <textarea>
element is perfect for getting comments and reviews from the user. We can specify the size of the text area by providing the maximum rows and columns in it using <rows>
and <cols>
or using CSS.
A fixed-width text area can be used for unlimited characters. We can define id to it as well to get the value.
Syntax:
<textarea id="elementId" name="inputName" rows="3" cols="40">
Default placeholder or any dummy text.
</textarea>
Now, let’s create a simple webpage in which we will use both input field elements to get data like full name, email and comment from the user and display it on-click of the button.
Code Example:
<!DOCTYPE html>
<html>
<body>
<h2>DelftStack learning</h2>
<h3>JavaScript comment box example</h3>
<form id="myForm">
Full name: <input id="userName" type="text" name="fname">
<br><br>
Email : <input id="userEmail" type="text" name="email">
<br><br>
<textarea id ="userComment" rows="4" cols="50" name="comment"> Enter comment here...</textarea>
<br><br>
<input type="button" onclick="myFunction()" value="Submit">
</form>
<h5>Submitted data :</h5>
<p id="data"></p>
<script>
function myFunction(){
let data = ""; let name = document.getElementById("userName").value
let email = document.getElementById("userEmail").value
let comment = document.getElementById("userComment").value
data = "User name : "+name+"<br/>User email : "+email+ "<br/>User comment : "+comment
document.getElementById("data").innerHTML = data // display data to paragraph
}
</script>
</body>
</html>
Output:
In the above html source, we have used the paragraph form tag <form></form>
to create user input fields. In that tag, we have defined multiple input elements type of text to get the user name and email, and we have to define ids to that element.
In myFunction()
we are getting all the user input values using document.getElementById("id")
and storing into variables. We used concatenations (+) to create a single string and displayed that string in a paragraph which is under the submitted data heading.