How to Marquee Element in JavaScript
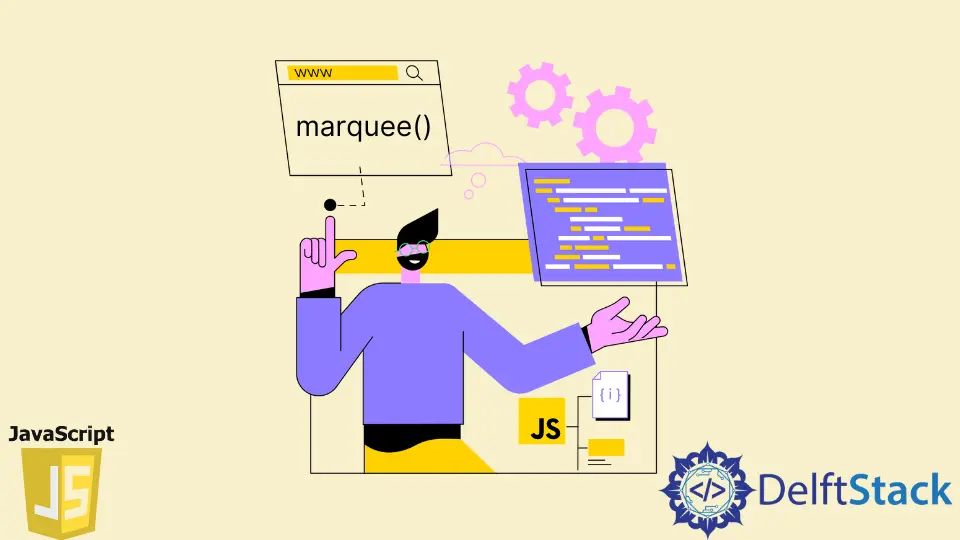
We mostly require decorating our content text on a website or web page. Marquee is one of that features to beautify the representation of content.
If we need to scroll the content automatically from Top to Bottom, Bottom to Top, Left to Right and Right to left, we normally use the marquee in our source code. In this article, we will learn the HTML default tag and JavaScript custom code to implement marquee.
Use Marquee Tag in HTML
In HTML, there is a marquee tag to auto-scroll the web content. By default, the content will scroll right to the left, but we can customize the scroll direction (up, down, right, left) like direction="up"
, but this tag has been deprecated in HTML 5.
Example Code:
<html>
<head>
<title>Example for HTML Marquee Tag</title>
</head>
<h1>
Delft stack marquee tag example
</h1>
<marquee width="40%" direction="up" height="30%">
Delft stack is a best website to learn programming skills!
</marquee>
</html>
In the above html source code, we used the default HTML marquee tag on text content. We customized the direction to up, defined height and width with inline CSS.
Use JavaScript to Add Marquee Feature
We can add the marquee feature to web content using JavaScript and J-Query with the help of custom functions. We can scroll the content to the desired direction using height and width positions.
We define the ID to the content we want to animate and scroll and pass those ids to the custom function of a marquee.
Example Code:
<html>
<head>
<title>Example for JavaScript Marquee</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
</head>
<style>
#text{
cursor:pointer;
overflow:hidden;
position:absolute;
left:10px;
margin-right:10px;
top:10px;
}
</style>
<script>
function marquee(a, b) {
var width = b.width();
var start_pos = a.width();
var end_pos = -width;
function scroll() {
if (b.position().left <= -width) {
b.css('left', start_pos);
scroll();
}
else {
time = (parseInt(b.position().left, 10) - end_pos) *
(10000 / (start_pos - end_pos)); //we can increase or decrease speed by changing value 10000
b.animate({
'left': -width
}, time, 'linear', function() {
scroll();
});
}
}
b.css({
'width': width,
'left': start_pos
});
scroll(a, b);
}
$(document).ready(function() {
marquee($('#display'), $('#text')); //we need to add name of container element & marquee element
});
</script>
<h1 id="text" >
Delft stack is a best website to learn programming skills!
</h1>
</html>
We used J-Query Google CDN in the above source code to use J-Query functions. Include the J-Query Google CDN link inside the head of the HTML page source.
We have declared a custom marquee function. It helps to animate and scroll the content using height width positions and CSS.
We used the H1 tag to display the text content and define the ID to that HTML element. Inside the (document).ready()
, we passed a marquee function that will take element ID as an argument and animate that specific HTML element.