How to Merge Two Arrays Without Any Duplicates in JavaScript
- JavaScript Merge Arrays Using the Spread Syntax in ECMAScript 6
-
JavaScript Merge Arrays Using
Array.concat
Function in ECMAScript 5 -
Remove Duplicates From an Array Using the
for
Loop -
JavaScript Remove Array Duplicates From an Array Using
Array.prototype
in ECMAScript 6 -
JavaScript Remove Duplicates From Array Using
Object.defineProperty
in ECMAScript 5 -
Merge and Only Keep Unique Values Using
Lo-Dash
orUnderscore.js
-
Merge and Only Keep Unique Values Using
Set
in ECMAScript 6 -
Merge Without Duplicate Values Using
for
Loop andDictionary
With Just O(n) Complexity
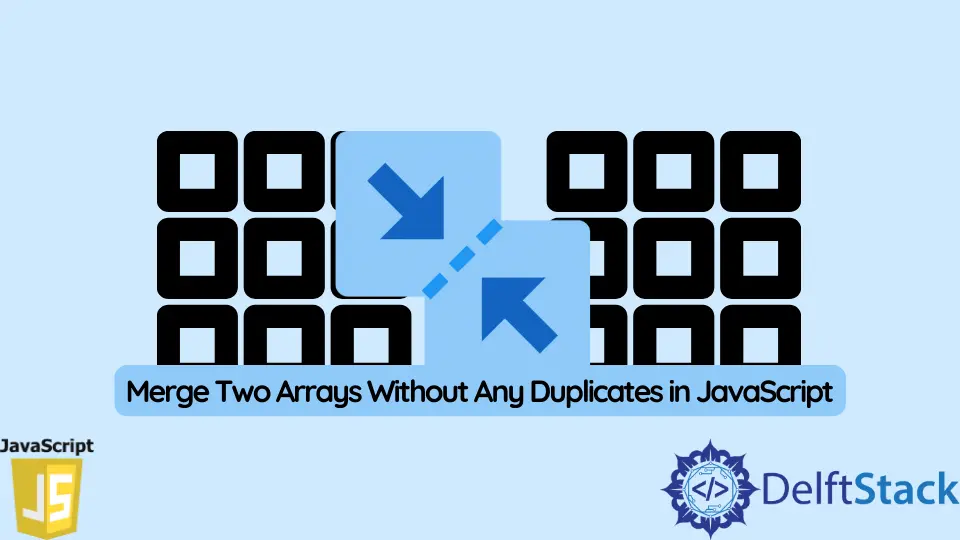
This tutorial will learn how we can merge two arrays in JavaScript without any duplicate values.
First, we will show the different merging methods in both ES5 and ES6 versions.
Then, we will learn how to remove the duplicates using different methods.
Finally, you will learn to do the operation in only one step, either by writing your own functions or using external libraries.
Let’s assume we have two arrays arrayA
and arrayB
and we want to merge them in arrayC
:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
var arrayC;
JavaScript Merge Arrays Using the Spread Syntax in ECMAScript 6
The spread syntax (...
) is used to expand the contents of iterable elements in a place where elements like arrays are expected, or where zero or more argument is expected in a function, for example:
var arr = [2, 4];
function add(x, y) {
return x + y;
}
console.log(add(...arr));
Now lets merge our arrays into arrayC
using the spread syntax:
arrayC = [...arrayA, ...arrayB];
console.log(arrayC);
Example Code:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
var arrayC;
console.log("Array A > "+arrayA);
console.log("Array B > "+arrayB);
//merging using the spread syntax
arrayC = [...arrayA,...arrayB];
console.log("Merged Using Spread Syntax >"+arrayC);
Output:
Array A > Java,JavaScript
Array B > C#,PHP,Java
Merged Using Spread Syntax >Java,JavaScript,C#,PHP,Java
JavaScript Merge Arrays Using Array.concat
Function in ECMAScript 5
Merging using the Array.concat
is used to concatenate the contents the input array ( arrayB
) to the contents of the source array ( arrayA
).
arrayC = arrayA.concat(arrayB);
console.log(arrayC);
If we want to remove the duplicates from the resulted array, we can do it in multiple ways, so let’s see how we can do it.
Example:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
var arrayC;
console.log("Array A > "+arrayA);
console.log("Array B > "+arrayB);
//merging using Array.concat function in ECMAScript 5
arrayC = arrayA.concat(arrayB);
console.log("Merged Using Array.concat function >"+arrayC);
Output:
Array A > Java,JavaScript
Array B > C#,PHP,Java
Merged Using Array.concat function >Java,JavaScript,C#,PHP,Java
Remove Duplicates From an Array Using the for
Loop
The simplest way to remove the duplicates is to create your own nested for loop.
Our Algorithm will be like:
- Create a clone from the input array
inArray
so we will not change the original array. - Create a nested loop to find and remove the duplicate element found using
arr.splice()
. The outer starts fromn
and the inner fromn+1
.
function removeDuplicates(inArray) {
var arr =
inArray
.concat() // create a clone from inArray so not to change input array
// create the first cycle of the loop starting from element 0 or n
for (var i = 0; i < arr.length; ++i) {
// create the second cycle of the loop from element n+1
for (var j = i + 1; j < arr.length; ++j) {
// if the two elements are equal , then they are duplicate
if (arr[i] === arr[j]) {
arr.splice(j, 1); // remove the duplicated element
}
}
}
return arr;
}
Then we can use that removeDuplicates
function like any other function.
var arrayWithoutDuplicates = removeDuplicates(arrayC);
console.log(arrayWithoutDuplicates);
Example:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
var arrayC;
console.log("Array A > "+arrayA);
console.log("Array B > "+arrayB);
//removing duplicates from an array using nested for loop
function removeDuplicates(inArray){
var arr = inArray.concat() // create a clone from inArray so not to change input array
//create the first cycle of the loop starting from element 0 or n
for(var i=0; i<arr.length; ++i) {
//create the second cycle of the loop from element n+1
for(var j=i+1; j<arr.length; ++j) {
//if the two elements are equal , then they are duplicate
if(arr[i] === arr[j]) {
arr.splice(j, 1); //remove the duplicated element
}
}
}
return arr;
}
arrayC = arrayA.concat(arrayB);
console.log("Merged arrayC > "+ arrayC );
console.log("Removing duplicates using removeDuplicates > "+ removeDuplicates(arrayC) );
Output:
Array A > Java,JavaScript
Array B > C#,PHP,Java
Merged arrayC > Java,JavaScript,C#,PHP,Java
Removing duplicates using removeDuplicates > Java,JavaScript,C#,PHP
JavaScript Remove Array Duplicates From an Array Using Array.prototype
in ECMAScript 6
We can also remove the duplicates using Array.prototype
in case we can use ECMAScript 6.
What does even prototype
mean? The prototype
is like the Object associated with all functions and Objects in JavaScript
.
Let’s see how we can use the Array.prototype
.
Our Algorithm will be like:
- Create a clone from the array Object
this.concat()
so we will not change the original array - Create a nested loop to find and remove the duplicate element found using
arr.splice()
. The outer start fromn
and the inner fromn+1
.
Array.prototype.removeDuplicates = function() {
var arr = this.concat(); // create a clone from the input so not to change
// the source
// create the first cycle of the loop starting from element 0 or n
for (var i = 0; i < arr.length; ++i) {
// create the second cycle of the loop from element n+1
for (var j = i + 1; j < arr.length; ++j) {
// if the two elements are equal , then they are duplicate
if (arr[i] === arr[j]) {
arr.splice(j, 1); // remove the duplicated element
}
}
}
return arr;
}
Then calling that prototype could be like:
var arrayWithoutDuplicates = arrayC.removeDuplicates();
console.log(arrayWithoutDuplicates);
Example:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
var arrayC;
console.log("Array A > "+arrayA);
console.log("Array B > "+arrayB);
arrayC = arrayA.concat(arrayB);
//removing duplicates from an array using Array.prototype in ECMAScript 6
Array.prototype.removeDuplicatesPrototype = function() {
var arr = this.concat(); // get the input array
//create the first cycle of the loop starting from element 0 or n
for(var i=0; i<arr.length; ++i) {
//create the second cycle of the loop from element n+1
for(var j=i+1; j<arr.length; ++j) {
//if the two elements are equal , then they are duplicate
if(arr[i] === arr[j]) {
arr.splice(j, 1); //remove the duplicated element
}
}
}
return arr;
}
console.log("Merged arrayC > "+arrayC);
console.log("Removing duplicates using removeDuplicatesPrototype > "+arrayC.removeDuplicatesPrototype());
JavaScript Remove Duplicates From Array Using Object.defineProperty
in ECMAScript 5
If we can only use ECMAScript 5, we can create our own property using Object.defineProperty
with that way we will be able to remove duplicates from all Array
type elements.
Here we define the property type as an array prototype by writing by typing Array.prototype
. The name of the property should be inserted as the next parameter as removeDuplicates
.
Let’s see how it is written correctly:
Object.defineProperty(Array.prototype, 'removeDuplicates', {
// defining the type and name of the property
enumerable: false,
configurable: false,
writable: false,
value: function() {
var arr = this.concat(); // get the input array
// create the first cycle of the loop starting from element 0 or n
for (var i = 0; i < arr.length; ++i) {
// create the second cycle of the loop from element n+1
for (var j = i + 1; j < arr.length; ++j) {
// if the two elements are equal , then they are duplicate
if (arr[i] === arr[j]) {
arr.splice(j, 1); // remove the duplicated element
}
}
}
return arr;
}
});
Then we can call that property directly like that:
var arrayWithoutDuplicates = arrayC.removeDuplicates();
console.log(arrayWithoutDuplicates);
Example
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
var arrayC;
console.log("Array A > "+arrayA);
console.log("Array B > "+arrayB);
arrayC = arrayA.concat(arrayB);
//removing duplicates from an array using defineProperty in ECMAScript 5
Object.defineProperty(Array.prototype, 'removeDuplicatesProperty', { //defining the type and name of the property
enumerable: false,
configurable: false,
writable: false,
value: function() {
var arr = this.concat(); // get the input array
//create the first cycle of the loop starting from element 0 or n
for(var i=0; i<arr.length; ++i) {
//create the second cycle of the loop from element n+1
for(var j=i+1; j<arr.length; ++j) {
//if the two elements are equal , then they are duplicate
if(arr[i] === arr[j]) {
arr.splice(j, 1); //remove the duplicated element
}
}
}
return arr;
}
});
console.log("Merged arrayC > "+arrayC);
console.log("Removing duplicates using removeDuplicatesProperty > "+arrayC.removeDuplicatesProperty() );
Merge and Only Keep Unique Values Using Lo-Dash
or Underscore.js
If the target is to get a new array of unique values out of two or more arrays and use external files, we can use the Lo-Dash
Library to do so.
First, We need to import the library using Cloudflare CDN
inside the HTML Template ,
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.15/lodash.min.js"></script>
Then we use the library like:
arrayC = _.union(arrayA, arrayB);
Example:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
console.log('Array A > ' + arrayA);
console.log('Array B > ' + arrayB);
// merging arrayA and arrayB keeping only unique values using Lo-Dash library
// don't forget to import the script using <script
// src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.15/lodash.min.js"></script>
console.log(
'Merging arrayA and arrayB keeping only unique values using Lo-Dash Lib. > ' +
_.union(arrayA, arrayB));
Merge and Only Keep Unique Values Using Set
in ECMAScript 6
If we can use ECMAScript 6 and our target is only the unique values of multiple arrays, then Set
can be a very good option.
we can use it like the example below using the spread
syntax:
arrayC = [...new Set([...arrayA, ...arrayB])];
Or we can use it like that
arrayC = Array.from(new Set(arrayA.concat(arrayB)));
Example:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
console.log('Array A > ' + arrayA);
console.log('Array B > ' + arrayB);
// merging arrayA and arrayB keeping unique values using Set in ECMAScript 6
console.log(
'Merging arrayA and arrayB keeping only unique values using Set > ' +
Array.from(new Set(arrayA.concat(arrayB))));
console.log(
'Merging arrayA and arrayB keeping only unique values using Set with spread syntax > ' +
[...new Set([...arrayA, ...arrayB])]);
Merge Without Duplicate Values Using for
Loop and Dictionary
With Just O(n) Complexity
Another way to merge two arrays and get the results without having any duplicate values is by using the idea of the Dictionary
in JavaScript
where we can’t have two duplicate values.
function mergeAndGetUnique(arrayA, arrayB) {
var hash = {};
var x;
for (x = 0; i < arrayA.length; i++) {
hash[arrayA[i]] = true;
}
for (x = 0; i < arrayB.length; i++) {
hash[arrayB[i]] = true;
}
return Object.keys(hash);
}
Then we can use our function like that:
arrayC = mergeAndGetUnique(arrayA, arrayB);
Example:
var arrayA = ['Java', 'JavaScript'];
var arrayB = ['C#', 'PHP', 'Java'];
var arrayC;
console.log("Array A > "+arrayA);
console.log("Array B > "+arrayB);
arrayC = arrayA.concat(arrayB);
//merging arrayA and arrayB keeping unique values using dictionary with O(n) complexity
function mergeAndGetUnique(arrayA, arrayB) {
var hash = {};
var x;
for (x = 0; x < arrayA.length; x++) {
hash[arrayA[x]] = true;
}
for (x = 0; x < arrayB.length; x++) {
hash[arrayB[x]] = true;
}
return Object.keys(hash);
}
console.log("Merging arrayA and arrayB keeping only unique values using Set with spread syntax > "+ mergeAndGetUnique(arrayA, arrayB) );