Image Onload Event in JavaScript
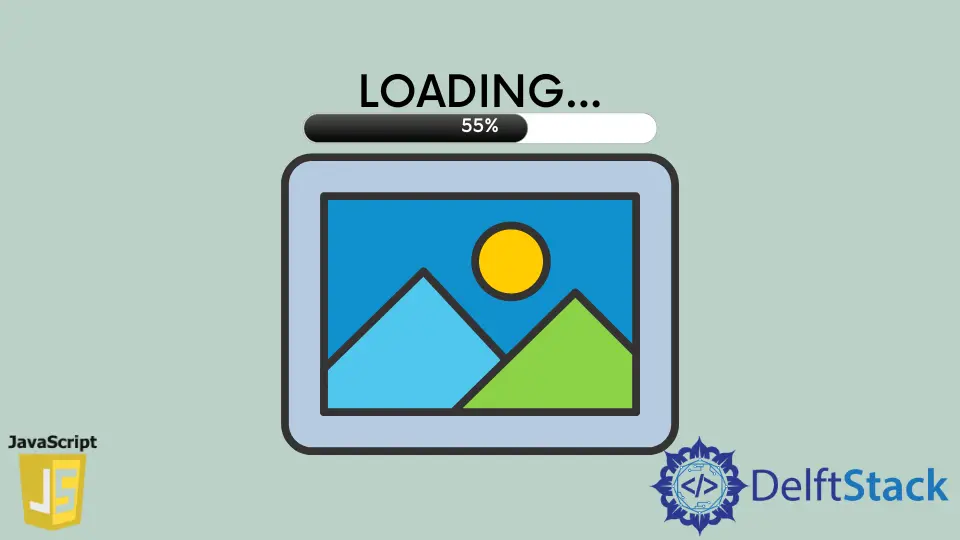
This article will discuss how we can handle the .onload
event in JavaScript. We will learn how to create an alert box using JavaScript when an image has been uploaded.
We will also find out how we can check whether an image is loaded or not using JavaScript by creating an alert box.
the .onload
Event in JavaScript
The .onload
event occurs only when an object has been loaded. The .onload
event is mainly used within the element body to execute a script once a web page has loaded all contents, including images, script, CSS files, etc.
The browsers used will allow us to track the loading of external resources such as images, scripts, iframes, etc. When we upload an image, and if it is saved in the browser cache, the onload
event will be fired.
How can we create an alert box that displays when an image is loaded regardless of whether the image is cached?
We need to create an alert box that will display a message that says The image is loaded successfully
when the onload
event fires on an image. We can tackle this problem using JavaScript and HTML.
the image.onload
Event in JavaScript
Using JavaScript, we can check whether an image has been completely loaded or not. We will use the HTMLimageElement
interface’s complete attribute.
This attribute returns true
when the image has been fully uploaded and false
otherwise. We have the naturalWidth
and naturalHeight
properties in JavaScript, and we can use either.
We used .naturalWidth
in the example code, which will return true
if the image loads successfully and returns 0
if not.
HTML code:
<!DOCTYPE html>
<html>
<head>
<title>Onload event using Javascript</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<h1 class="title">Onload event using Javascript</h1>
<script src="script.js"></script>
<img src="https://secure.gravatar.com/avatar?d=wavatar">
</body>
</html>
JavaScript code:
window.addEventListener('load', event => {
var image = document.querySelector('img');
var isLoadedSuccessfully = image.complete && image.naturalWidth !== 0;
alert(isLoadedSuccessfully);
});
If we run this code, it will return true
, which means the image is loaded successfully.
You can see the live demo of the example code above by clicking here.
the image.onload
Event in HTML
In case we don’t want to use JavaScript, we can use HTML attributes to check whether an image has loaded or not. In HTML, we can use onload
and onerror
attributes.
In HTML, the onload
attribute fires when the image is loaded successfully, while the onerror
attribute fires if an error happens while loading an image.
We used onload
and onerror
attributes in the following code. So, with the help of these attributes, we can create an alert box that will display a message that says The image is loaded successfully
when the image is loaded.
If an error occurs while loading the image, it will display a message that says the image is not loaded
.
HTML code:
<!DOCTYPE html>
<html>
<head>
<title>Onload event using HTML</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<h1 class="title">Onload event using HTML </h1>
<p id="currentTime"></p>
<script src="script.js"></script>
<img src="https://secure.gravatar.com/avatar?d=wavatar"
onload="javascript: alert('The image is loaded successfully')"
onerror="javascript: alert('Image is not loaded')" />
</body>
</html>
If we run the code, it will display an alert message that The image is loaded successfully
.
Click here to see the live demo of the given example code.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn