How to Get the Last Item in an Array in JavaScript
- Using Array Length Property
- Using the Array Slice Method
- Using the Array Pop Method
- Using the Array at Method (ES2022)
- Conclusion
- FAQ
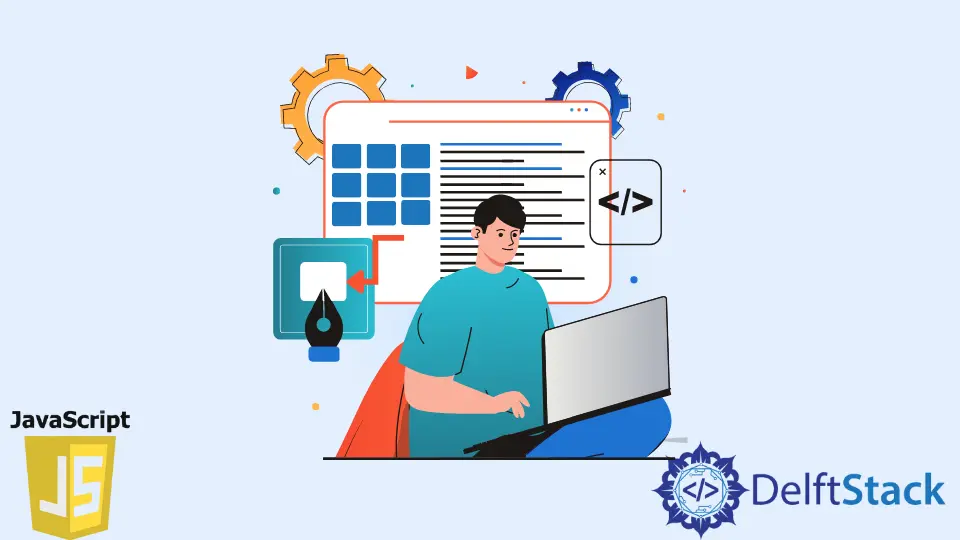
When working with arrays in JavaScript, there are often times when you need to access the last item. Whether you’re building a dynamic web application, manipulating data, or simply learning the ropes of JavaScript, knowing how to retrieve that last element efficiently can save you time and effort.
In this article, we’ll explore various methods to get the last item in an array, each with its own advantages. By the end, you’ll have a solid understanding of how to handle this common task, along with practical code examples to illustrate each approach. Let’s dive in!
Using Array Length Property
One of the simplest and most straightforward methods to get the last item in an array is by leveraging the length
property. This property returns the number of elements in an array, allowing us to access the last element using its index. Remember, array indices start from zero, so the last element will always be at the index of length - 1
.
Here’s how you can do it:
const array = [1, 2, 3, 4, 5];
const lastItem = array[array.length - 1];
console.log(lastItem);
Output:
5
In this code snippet, we first define an array containing five numbers. By accessing array.length
, we get the total number of elements, which is 5. We then subtract 1 to get the index of the last item, which is 4. Finally, we log the last item to the console, which displays the number 5. This method is efficient and easy to understand, making it a popular choice among developers.
Using the Array Slice Method
Another effective way to retrieve the last item in an array is by using the slice()
method. This method is typically used for creating a shallow copy of a portion of an array, but it can also be employed to get the last element by specifying a negative index. Negative indices count backward from the end of the array, making this approach quite intuitive.
Here’s how you can implement it:
const array = [1, 2, 3, 4, 5];
const lastItem = array.slice(-1)[0];
console.log(lastItem);
Output:
5
In this example, we again define an array of numbers. By calling array.slice(-1)
, we create a new array containing only the last element. Since slice()
returns an array, we need to access the first element of this new array using [0]
. The result is the same as before, and we log it to the console. This method is particularly useful if you want to retrieve more than one element from the end of the array, as you can adjust the number passed to slice()
.
Using the Array Pop Method
If you want to not only access but also remove the last item from an array, the pop()
method is your go-to solution. This method modifies the original array by removing the last element and returning it. It’s a great option when you need to work with both the last item and the modified array.
Here’s an example:
const array = [1, 2, 3, 4, 5];
const lastItem = array.pop();
console.log(lastItem);
console.log(array);
Output:
5
Output:
[1, 2, 3, 4]
In this snippet, we define an array and then call array.pop()
. This removes the last element from the array and assigns it to the variable lastItem
. We then log the last item, which is 5, followed by logging the modified array, which now contains only the first four elements. This method is particularly useful when you want to reduce the size of your array while simultaneously retrieving its last element.
Using the Array at Method (ES2022)
With the introduction of ES2022, JavaScript now supports the at()
method, which allows you to access elements in an array using both positive and negative indices. This method is particularly handy for accessing the last item without needing to calculate the length.
Here’s how it works:
const array = [1, 2, 3, 4, 5];
const lastItem = array.at(-1);
console.log(lastItem);
Output:
5
In this code, we define our array and then use array.at(-1)
to directly access the last element. This approach is clean and concise, making it an excellent choice for modern JavaScript development. The at()
method enhances readability and reduces the chances of errors that can occur when manually calculating indices.
Conclusion
Retrieving the last item in an array is a fundamental skill for any JavaScript developer. Whether you choose to use the length property, slice method, pop method, or the new at method, each approach has its unique advantages. By understanding these different methods, you can select the one that best fits your specific use case. As you continue to work with arrays in JavaScript, these techniques will become second nature, enhancing your coding efficiency and effectiveness.
FAQ
-
What is the fastest way to get the last item in an array?
Using the length property is often considered the fastest method, as it directly accesses the last element without creating new arrays. -
Can I use negative indices in JavaScript arrays?
Yes, negative indices can be used with theslice()
andat()
methods to access elements from the end of the array. -
Does the
pop()
method modify the original array?
Yes, thepop()
method removes the last item from the original array, altering its length. -
What is the difference between slice() and at() methods?
Theslice()
method returns a new array, while theat()
method returns the element itself without modifying the original array. -
Is there a method to get multiple last items from an array?
Yes, you can use theslice()
method with a negative index to retrieve multiple last items by specifying how many you want to return.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript