How to Filter Array of Objects in JavaScript
- Understanding the JavaScript filter() Method
- Filtering an Array of Objects
- Filtering with Multiple Criteria
-
Using the
filter()
Method with Complex Objects - Conclusion
- FAQ
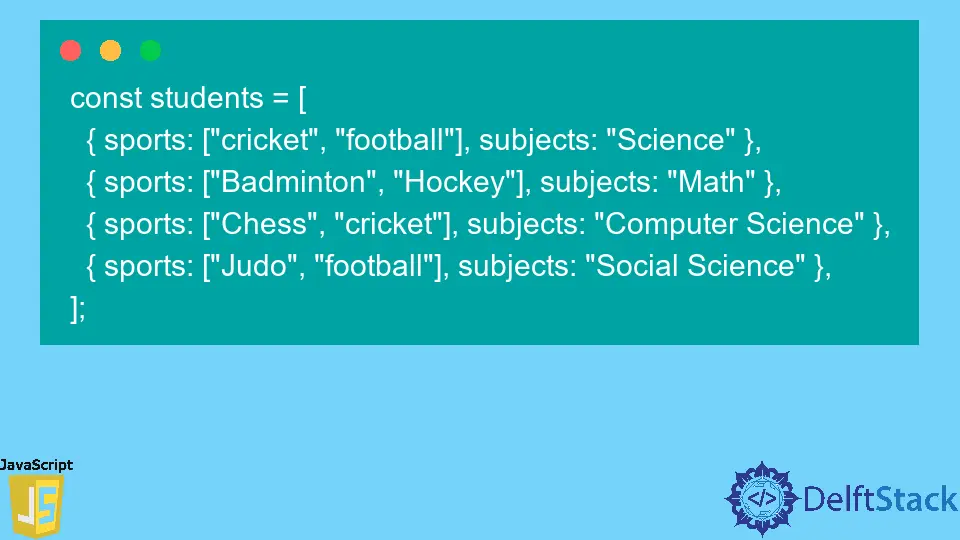
Filtering an array of objects in JavaScript can seem daunting at first, especially if you’re new to the language. However, with the right tools and methods, it becomes a straightforward task. One of the most powerful functions at your disposal is the filter()
method. This method allows you to create a new array that contains only the elements that meet certain criteria, making it an essential tool for any JavaScript developer.
In this article, we will explore how to effectively use the filter()
method to sift through arrays of objects, providing you with practical examples and detailed explanations to enhance your understanding. Whether you’re working with data from an API or manipulating local data structures, mastering this technique will significantly improve your coding skills.
Understanding the JavaScript filter() Method
The filter()
method in JavaScript creates a new array populated with all elements that pass the test implemented by the provided function. It does not change the original array and can be used on arrays of any data type, including objects. The method takes a callback function as an argument, which is executed for each element in the array. If the callback function returns true
, the element is included in the new array; if it returns false
, the element is excluded.
Here’s a basic example of how the filter()
method works:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers);
Output:
[2, 4]
In this example, the filter()
method is used to create a new array containing only the even numbers from the original numbers
array. The callback function checks if each number is even by using the modulus operator. If it is, that number gets included in the evenNumbers
array.
Filtering an Array of Objects
When working with arrays of objects, the filter()
method becomes even more powerful. Suppose you have an array of user objects, each containing properties like name
, age
, and city
. You can easily filter this array to find users who match specific criteria.
Let’s say we want to filter users who are over 18 years old. Here’s how you can achieve this:
const users = [
{ name: "Alice", age: 25, city: "New York" },
{ name: "Bob", age: 17, city: "Chicago" },
{ name: "Charlie", age: 30, city: "San Francisco" }
];
const adults = users.filter(user => user.age > 18);
console.log(adults);
Output:
[
{ name: "Alice", age: 25, city: "New York" },
{ name: "Charlie", age: 30, city: "San Francisco" }
]
In this example, we have an array of user objects. The filter()
method checks each user’s age and returns a new array containing only those who are over 18. This is particularly useful when you need to display or process data based on specific conditions.
Filtering with Multiple Criteria
You can also filter arrays based on multiple criteria. For instance, if you want to find users who are over 18 and live in New York, you can modify the callback function accordingly. Here’s how:
const users = [
{ name: "Alice", age: 25, city: "New York" },
{ name: "Bob", age: 17, city: "Chicago" },
{ name: "Charlie", age: 30, city: "San Francisco" },
{ name: "David", age: 22, city: "New York" }
];
const newYorkAdults = users.filter(user => user.age > 18 && user.city === "New York");
console.log(newYorkAdults);
Output:
[
{ name: "Alice", age: 25, city: "New York" },
{ name: "David", age: 22, city: "New York" }
]
In this code snippet, the filter()
method is used to find users who are both over 18 and reside in New York. By combining conditions with the logical AND operator (&&
), you can refine your results even further. This capability is particularly useful in applications where you need to filter data based on user input or other dynamic criteria.
Using the filter()
Method with Complex Objects
Sometimes, the objects in your array might contain nested structures. The filter()
method can still be used effectively in these scenarios. For instance, consider an array of products, where each product has a category and a price. You might want to filter products based on their category and price range.
Here’s an example:
const products = [
{ name: "Laptop", category: "Electronics", price: 999 },
{ name: "Shoes", category: "Fashion", price: 59 },
{ name: "Smartphone", category: "Electronics", price: 699 },
{ name: "Jacket", category: "Fashion", price: 120 }
];
const affordableElectronics = products.filter(product =>
product.category === "Electronics" && product.price < 800
);
console.log(affordableElectronics);
Output:
[
{ name: "Smartphone", category: "Electronics", price: 699 }
]
In this example, we filtered the products
array to find affordable electronics under 800. The filter()
method allows you to dive deep into the properties of each object, making it a versatile tool for data manipulation. This flexibility is crucial when dealing with complex data structures in modern web applications.
Conclusion
Mastering the filter()
method in JavaScript is essential for any developer looking to manipulate arrays of objects efficiently. Whether you’re filtering based on simple criteria or navigating complex data structures, this method provides the tools you need to create clean, readable, and effective code. With practice, you’ll find that filtering data becomes second nature, allowing you to focus on building robust applications.
FAQ
- What is the
filter()
method in JavaScript?
Thefilter()
method creates a new array with elements that pass a test defined by a provided function.
-
Can I use filter() on arrays of objects?
Yes, thefilter()
method can be used on arrays of objects to return elements that meet specific criteria. -
Does filter() modify the original array?
No, thefilter()
method does not change the original array; it returns a new array. -
Can I filter based on multiple conditions?
Yes, you can combine multiple conditions using logical operators like AND (&&
) and OR (||
) in the callback function. -
What happens if no elements pass the filter?
If no elements pass the filter, it returns an empty array.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript