How to Declare Empty Array in JavaScript
- Method 1: Using Array Literal Syntax
- Method 2: Using the Array Constructor
- Method 3: Using Array.of()
- Method 4: Using Array.from()
- Conclusion
- FAQ
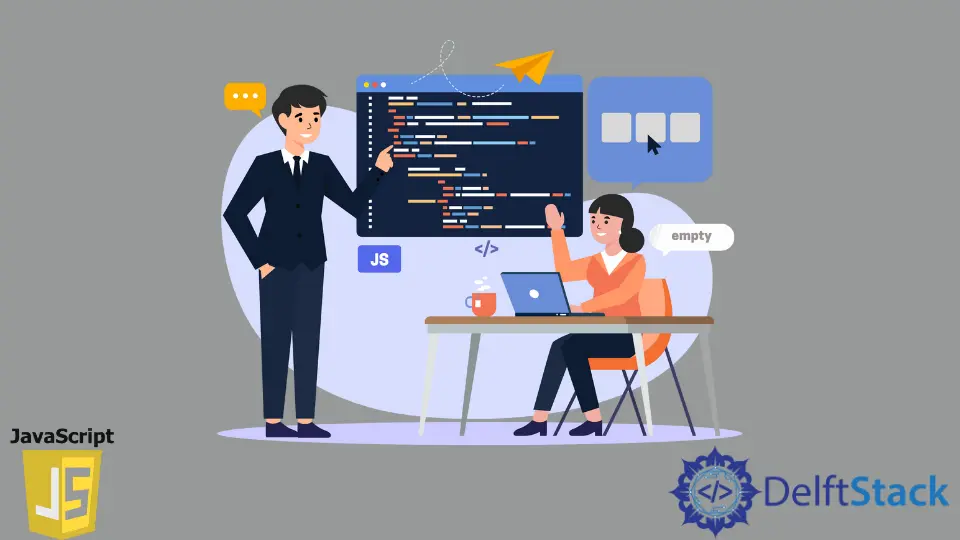
Declaring an empty array in JavaScript is a fundamental skill for any developer. Arrays are versatile data structures that allow you to store multiple values in a single variable. Whether you’re managing lists of items, processing data, or handling user inputs, knowing how to create an empty array is essential.
In this article, we’ll explore different methods to declare an empty array in JavaScript, providing you with clear examples and explanations. By the end, you’ll be equipped with the knowledge to efficiently utilize arrays in your coding projects. Let’s dive in!
Method 1: Using Array Literal Syntax
One of the most common ways to declare an empty array in JavaScript is by using the array literal syntax. This method is straightforward and easy to understand.
let emptyArray = [];
Output:
[]
In this example, we create a variable named emptyArray
and assign it an empty array using square brackets. This method is preferred for its simplicity and clarity. It’s also the most concise way to initialize an array, making your code cleaner and easier to read.
You can later add elements to this array using methods like .push()
or .unshift()
. For instance, if you wanted to add a number to your empty array, you could do so as follows:
emptyArray.push(10);
Output:
[10]
Using the array literal syntax is not only the most common method but also the most efficient in terms of performance. It directly communicates your intent to create an array, making it a favorite among JavaScript developers.
Method 2: Using the Array Constructor
Another way to declare an empty array is by using the Array
constructor. This method is slightly less common but still valid.
let emptyArray = new Array();
Output:
[]
Here, we use the new
keyword along with the Array
constructor to create an empty array. While this approach works perfectly, it’s generally less preferred than the array literal syntax due to its verbosity.
The Array
constructor can also take an argument that specifies the initial length of the array. For example:
let arrayWithLength = new Array(5);
Output:
[empty × 5]
This creates an array with a length of 5, but all the elements are empty slots. Despite this flexibility, using the Array
constructor can lead to confusion, especially for new developers. Therefore, it’s best to stick with the array literal syntax unless you have a specific reason to use the constructor.
Method 3: Using Array.of()
The Array.of()
method is another modern way to create an empty array in JavaScript. This method was introduced in ECMAScript 2015 (ES6) and provides a more intuitive way to create arrays.
let emptyArray = Array.of();
Output:
[]
In this case, Array.of()
is called without any arguments, resulting in an empty array. This method is particularly useful when you want to create arrays from a set of arguments, but it can also be used to declare an empty array.
One of the benefits of using Array.of()
is that it avoids some of the pitfalls associated with the Array
constructor. For instance, if you pass a single numeric argument to the Array
constructor, it creates an array of that length. However, Array.of()
always creates an array with the exact elements you provide.
This method is a great choice for developers who want to ensure clarity and avoid confusion in their code. It’s worth noting that Array.of()
is widely supported in modern browsers, making it a safe choice for most applications.
Method 4: Using Array.from()
The Array.from()
method is another way to create an empty array in JavaScript. This method is particularly useful when you want to create an array from an array-like or iterable object.
let emptyArray = Array.from([]);
Output:
[]
In this example, we pass an empty array to Array.from()
, resulting in another empty array. This method is particularly beneficial when you want to convert other data types into an array. For instance, you can use it to convert a string or a NodeList into an array.
Using Array.from()
allows for more flexibility in your code, especially when working with different types of data. It’s a powerful tool to have in your JavaScript toolkit, especially when dealing with complex data structures.
Conclusion
Declaring an empty array in JavaScript is a simple yet crucial skill for any developer. Whether you choose to use the array literal syntax, the Array
constructor, Array.of()
, or Array.from()
, each method has its unique advantages. By understanding these different approaches, you can write cleaner, more efficient code and better manage your data structures. Remember, the choice of method can depend on your specific use case and coding style. So, experiment with these techniques and find what works best for you!
FAQ
-
What is the most common way to declare an empty array in JavaScript?
The most common way is using array literal syntax:let emptyArray = [];
. -
Can I declare an empty array using the Array constructor?
Yes, you can uselet emptyArray = new Array();
to declare an empty array. -
What is the difference between Array.of() and Array.from()?
Array.of()
creates an array from a set of arguments, whileArray.from()
converts an array-like or iterable object into an array. -
Are there performance differences between these methods?
Generally, the array literal syntax is the most efficient and preferred method for declaring empty arrays. -
Can I add elements to an empty array after declaring it?
Yes, you can add elements using methods like.push()
,.unshift()
, or by directly assigning values to specific indices.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript