How to Format a Number as a Currency String in JavaScript
-
Use the
Intl.NumberFormat()
Method to Format a Number as Currency String in JavaScript -
Use the
toLocaleString()
Method to Format a Number as a Currency String in JavaScript -
Use the
Numeral.js
Library to Format a Number as a Currency String in JavaScript
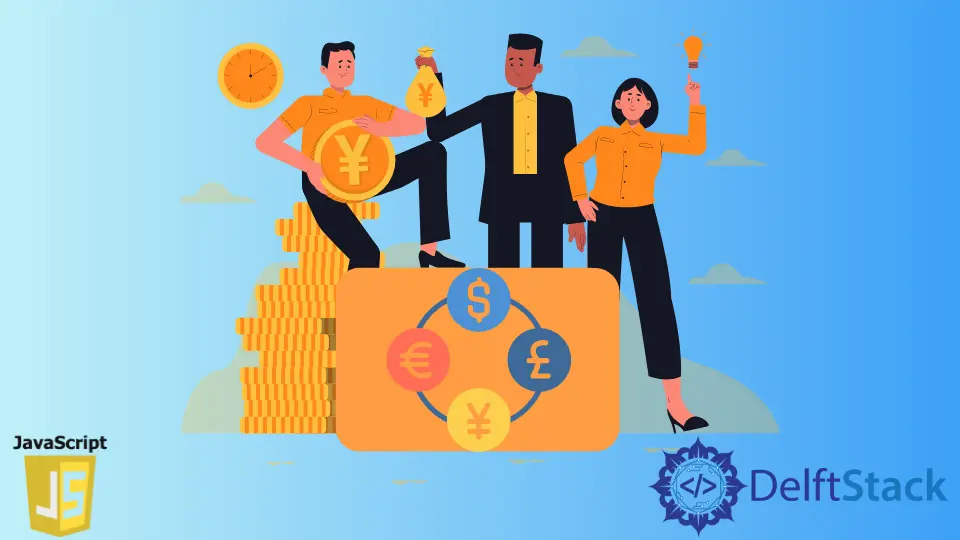
A number is represented in many ways, date/time, currency, number, and much more. When it is represented as a monetary value, its impact increases and becomes much more readable. For example, A monetary value written as 10000
is much less impactful than a number represented as $ 10,000.00
. It tells us so much more about the number, the different currency symbols, and the different comma separations. This tutorial teaches how to format a number as a currency string in JavaScript.
Use the Intl.NumberFormat()
Method to Format a Number as Currency String in JavaScript
This method is used to represent numbers in language-sensitive formatting and represent currency according to the parameters provided. It takes two parameters as input: locales
and options
.
locales
: It specifies the language and the region settings. It is a small string made up of a combination of language and region separated by a dash. For example, Theen-IN
locale takes the format of India and the English language. The first comma from right separates thousands and rest onwards in terms of hundreds.options
: It is an object consisting of tons of properties, or we can say options. when formatting a number as a currency string, there are 3 major options required.style
: It is simply the style for our number formatting. It can have3
different values,decimal
,currency
andpercent
, depending on the use-case. In our case, we will set the style ascurrency
.currency
: It specifies the symbol’s currency type to be included before the formatting number string, for example, US Dollar and Euro.minimumFractionDigits
: It specifies the minimum digits displayed after the decimal in the formatted string.
const money = 10000;
const currency = function(number) {
return new Intl
.NumberFormat(
'en-IN',
{style: 'currency', currency: 'INR', minimumFractionDigits: 2})
.format(number);
};
console.log(currency(money));
The above code helps to convert a given number into an Indian currency string.
Use the toLocaleString()
Method to Format a Number as a Currency String in JavaScript
This method is essentially the same as Intl.NumberFormat()
but is not usually used because of its slow speed with multiple items. For single items speed of both the methods is similar. It has the same parameters as Intl.NumberFormat()
. It just differs in speed due to the different internal implementation of both methods.
const number = 2000;
number.toLocaleString(
'en-IN', {style: 'currency', currency: 'INR', minimumFractionDigits: 2})
console.log(number);
The above code helps to convert a given number into an Indian currency string.
Use the Numeral.js
Library to Format a Number as a Currency String in JavaScript
Numeral.js
is one of the most widely used libraries for formatting numbers. To format numbers as currency string using this library, we first create an instance of a numeral. It is the standard data type used by this library that converts numbers or strings into a number before performing the formatting. After, this we can specify the currency format by using the format()
function.
<script src="//cdnjs.cloudflare.com/ajax/libs/numeral.js/2.0.6/numeral.min.js"></script>
The above code is used to include the Numeral.js
library in the browser.
var number = 1000;
var myNumeral = numeral(number);
var currencyString = myNumeral.format('$0,0.00');
We created a numeral and then called the format function to format the numeral as USD with 2 decimal points. The above code provides a much cleaner way of specifying the currency format.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn