How to Format a Decimal in JavaScript
-
Use the
toString()
Method to Format a Decimal in JavaScript -
Use the
toFixed()
Method to Format a Decimal in JavaScript -
Use the
toLocaleString()
Method to Format a Decimal in JavaScript
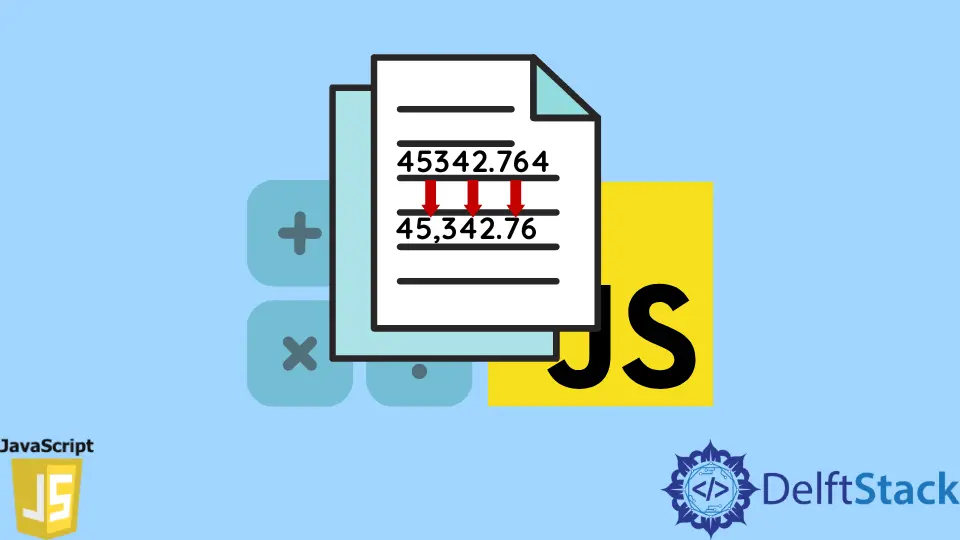
This article will look into how to format the decimal number places using JavaScript.
To format a number with commas and decimal places in JavaScript, we may use Math.round()
and split()
to a specified number. We can use the toFixed()
, toString()
, and toLocaleString()
methods to format the decimal number.
Use the toString()
Method to Format a Decimal in JavaScript
JavaScript’s toString()
method converts numbers to strings and returns a string representing the specified Number
object.
<!DOCTYPE html>
<html>
<body>
<script>
var numb = 45342.764;
var valu = Math.round(Number(numb) * 100) / 100;
var pts = valu.toString().split(".");
var num =
pts[0].replace(/\B(?=(\d{3})+(?!\d))/g, ",") +
(pts[1] ? "." + pts[1] : "");
console.log(num);
</script>
</body>
</html>
The .split()
function makes fragments of the string at specific spots into array components, which are then merged back into a series separated by commas.
Output:
"45,342.76"
You can even use the toString()
method by making a function.
<script>
var numb = 45342.76;
function numbCommas(x) {
return x.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
}
console.log(numbCommas(numb));
</script>
Output:
"45,342.76"
Use the toFixed()
Method to Format a Decimal in JavaScript
The toFixed()
method in JavaScript formats a number with two decimal places. The toFixed()
method formats the number with the specified number of digits to the right of the decimal point.
It outputs a string representation of a number with the correct number of decimal places but does not use scientific notation.
<script>
var number1 = 45342.764; document.write("number1 is : " + number1.toFixed(2));
document.write("
<br /> ");
</script>
Output:
number1 is: 45342.76
Use the toLocaleString()
Method to Format a Decimal in JavaScript
Likewise, we can use the toLocaleString()
method to separate decimal numbers with commas, which are different for different localities. Like en-US
separates numbers with commas at every 3 positions, whereas hi-IN
separates at the first 3 positions and then at every 2 places.
let decNum = 4534289.764;
console.log(decNum.toLocaleString('en-US'));
console.log(decNum.toLocaleString('hi-IN'));
Output:
"4,534,289.764"
"45,34,289.764"
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn