How to Check if Variable Exists in JavaScript
Kirill Ibrahim
Feb 02, 2024
JavaScript
JavaScript Variable
-
Use the
typeof
Operator to Check if Variable Exists in JavaScript: -
Use
if (varibale)
Statement to Check if Variable Exists in JavaScript:
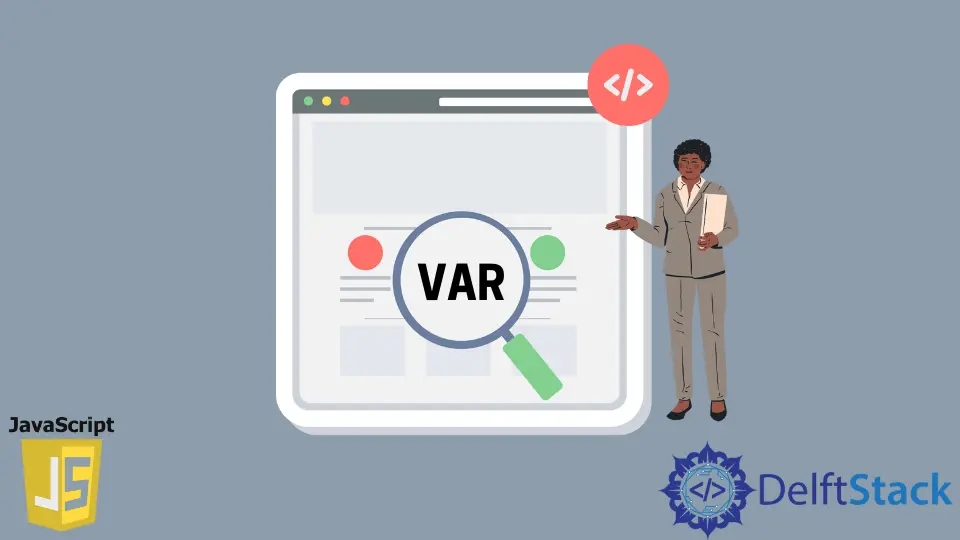
In this article, we will introduce multiple ways to check if a variable is defined/initialized. Every method below will have a code example, which you can run on your machine.
Use the typeof
Operator to Check if Variable Exists in JavaScript:
The typeof
operator checks if a variable is defined/null, but it does not throw a ReferenceError
if used with an undeclared variable.
Example:
<!DOCTYPE html>
<html>
<head>
<title>
How to check if variable exists in JavaScript?
</title>
</head>
<body style = "text-align:center;">
<h2 >
How to check if variable exists in JavaScript?
</h2>
<p>
variable-name : Vatiable1
</p>
<button onclick="checkVariable()">
Check Variable
</button>
<h4 id = "result" style="color:blue;"></h4>
<!-- Script to check existence of variable -->
<script>
const checkVariable = () => {
let Vatiable1;
let result = document.getElementById("result");
if (typeof Vatiable1 === 'undefined') {
result.innerHTML = "Variable is Undefined";
}
else {
result.innerHTML = "Variable is defined and"
+ " value is " + Vatiable1;
}
}
</script>
</body>
Example:
We will use the same html as above.
<script>
const checkVariable = () => {
let Vatiable1 = "variable 1";
let result = document.getElementById("result");
if (typeof Vatiable1 === 'undefined') {
result.innerHTML = "Variable is Undefined";
}
else {
result.innerHTML = "Variable is defined and"
+ " value is " + Vatiable1 ;
}
}
</script>
Example:
We will use the same html above, to check if the variable is null:
<script>
const checkVariable = () => {
let Vatiable1 = null;
let result = document.getElementById("result");
if (typeof Vatiable1 === 'undefined' ) {
result.innerHTML = "Variable is Undefined";
}
else if (Vatiable1 === null){
result.innerHTML = "Variable is null and not declared";
}
else {
result.innerHTML = "Variable is defined and"
+ " value is " + Vatiable1 ;
}
}
</script>
Use if (varibale)
Statement to Check if Variable Exists in JavaScript:
We can also use the if
statement to check if a variable exists because it covers and checks many cases like it checks whether the variable is undefined
, null
, ''
, 0
, Nan
, and false
. But the typeof
operator checks only undefined
or null
.
Example:
We will use the same html above:
<script>
const checkVariable = () => {
//let Vatiable1;
let Vatiable1 = null;
// let Vatiable1 = '';
let result = document.getElementById("result");
if(Vatiable1){
result.innerHTML = "Variable is defined and"
+ " value is " + Vatiable1 ;
}
else{
result.innerHTML = "Variable is Undefined"
}
}
</script>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript