How to Check for an Empty String in JavaScript
- Using the Length Property
- Using the Trim Method
- Using the Logical NOT Operator
- Using the Equality Operator
- Conclusion
- FAQ
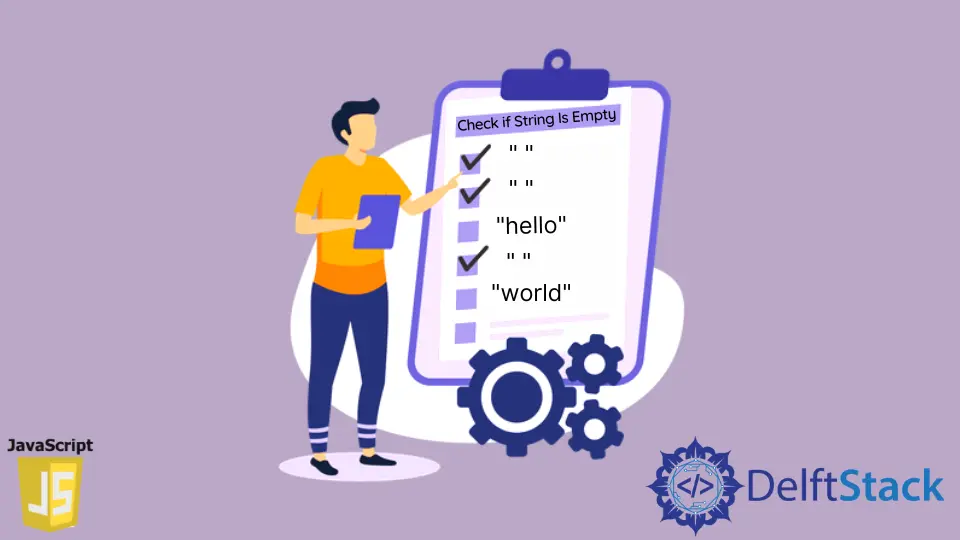
When working with JavaScript, one common task developers face is checking whether a string is empty or filled with content. An empty string can lead to unexpected behavior in your applications, especially when it comes to user input, API responses, and data validation. Understanding how to effectively check for empty strings is crucial for writing robust JavaScript code.
In this article, we will explore various methods to determine if a string is empty in JavaScript. From simple conditional checks to built-in methods, we will cover everything you need to know to handle strings like a pro.
Using the Length Property
One of the simplest ways to check if a string is empty in JavaScript is by using its length
property. This property returns the number of characters in the string. If the length is zero, then the string is considered empty. Here’s how you can implement this:
let str = "";
if (str.length === 0) {
console.log("The string is empty.");
} else {
console.log("The string is not empty.");
}
Output:
The string is empty.
In this code snippet, we declare a variable str
and assign it an empty string. The if
statement checks whether str.length
equals zero. If it does, the console logs that the string is empty. Otherwise, it indicates that the string contains characters. This method is straightforward and effective, making it a popular choice among developers.
Using the Trim Method
Sometimes strings may contain whitespace characters, which can lead to confusion when checking for emptiness. To handle this, you can use the trim()
method, which removes whitespace from both ends of a string. This way, you can accurately determine if a string is empty or just filled with spaces. Here’s how you can use it:
let str = " ";
if (str.trim().length === 0) {
console.log("The string is empty or whitespace.");
} else {
console.log("The string is not empty.");
}
Output:
The string is empty or whitespace.
In this example, the trim()
method is applied to str
, which consists solely of spaces. After trimming, its length is checked. If the length is zero, the string is considered empty or whitespace. This method is particularly useful when dealing with user inputs, as it ensures that only meaningful content is evaluated.
Using the Logical NOT Operator
Another elegant way to check for an empty string is by using the logical NOT operator (!
). In JavaScript, an empty string is considered a falsy value. Therefore, you can leverage this characteristic for a concise check. Here’s an example:
let str = "";
if (!str) {
console.log("The string is empty.");
} else {
console.log("The string is not empty.");
}
Output:
The string is empty.
In this case, the if
statement uses the !
operator to evaluate str
. If str
is an empty string, it evaluates to false
, and the !
operator converts it to true
, triggering the console log. This method is compact and efficient, making it a favorite among many developers for quick checks.
Using the Equality Operator
You can also use the equality operator (===
) to directly compare a string against an empty string. This method is straightforward and clear, making it easy to understand. Here’s how it works:
let str = "";
if (str === "") {
console.log("The string is empty.");
} else {
console.log("The string is not empty.");
}
Output:
The string is empty.
In this example, we directly compare str
with an empty string (""
). If they are equal, it confirms that str
is indeed empty. This method is simple and effective, ensuring clarity in your code. It’s particularly useful when you want to make your intentions obvious to anyone reading your code.
Conclusion
Checking for an empty string in JavaScript is a fundamental skill that every developer should master. Whether you choose to use the length property, trim method, logical NOT operator, or equality operator, each method has its own advantages. By understanding these techniques, you can write cleaner, more reliable code that handles user inputs and data validations effectively. Remember, a well-checked string can save you from unexpected bugs and improve your application’s overall performance.
FAQ
-
How do I check for an empty string in JavaScript?
You can check for an empty string using the length property, trim method, logical NOT operator, or by comparing it directly to an empty string. -
What is the difference between an empty string and a string with whitespace?
An empty string has no characters, while a string with whitespace contains spaces, tabs, or new lines. You can use the trim method to check for both. -
Is using the logical NOT operator a good practice for checking empty strings?
Yes, using the logical NOT operator is a concise and efficient way to check for empty strings, as it leverages JavaScript’s handling of falsy values. -
Can I use regular expressions to check for empty strings?
Yes, you can use regular expressions to check for empty strings or strings that contain only whitespace, but it may be more complex than necessary for simple checks. -
What happens if I forget to check for empty strings in my code?
Not checking for empty strings can lead to unexpected behavior, bugs, or errors in your application, especially when dealing with user inputs or API responses.