How to Modify URL in JavaScript
-
Use the
replaceState()
Method to Modify URL With JavaScript -
Use the
pushState()
Method to Modify URL With JavaScript
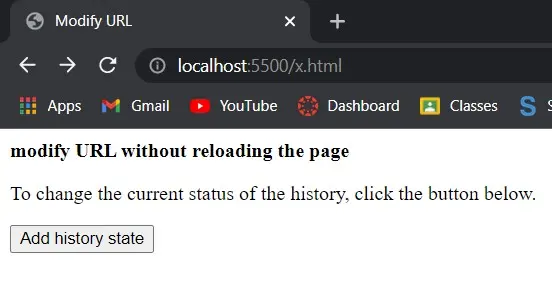
The browser’s history interface controls the browser’s session history. It contains the last page visited in the tab or frame in which the current page is displayed.
Manipulation of this state allows you to alter the browser’s URL without reloading the page. In this article, we’ll tackle modifying URLs using different methods in JavaScript.
Use the replaceState()
Method to Modify URL With JavaScript
The replaceState()
method replaces the current state in history by replacing the state’s properties with those from the provided parameters.
Syntax:
history.replaceState(data, title, url)
The data represents the current state of the browser’s history, the title refers to the new page’s title, and the URL refers to the URL of the new titled page.
Example:
<!DOCTYPE html>
<html>
<head>
<title>
modify URL
</title>
</head>
<body>
<b>
Modify URL without reloading the page
</b>
<p>
To change the current status of the history, click the button below.
</p>
<button onclick="modifyState()">
Modify history state
</button>
<script>
function modifyState() {
let stateObj = { id: "100" };
window.history.replaceState(stateObj, "x 2", "/x2.html");
}
</script>
</body>
</html>
Instead of producing a new history item, this approach updates the present one. When we wish to update the URL of the current history record, we utilize this method.
The URL can be altered by giving the required URL as a string to this method. This will modify the page’s URL without having to reload it.
Output:
Before clicking the button:
After clicking the button:
Use the pushState()
Method to Modify URL With JavaScript
The properties given as inputs to the pushState()
method create a new history entry. Without restarting the page, this will update the current URL to the new state specified.
Syntax:
history.pushState(state object, title, url)
Example:
<!DOCTYPE html>
<html>
<head>
<title>
Modify URL
</title>
</head>
<body>
<b>
modify URL without reloading
the page
</b>
<p>
To change the current status of the history, click the button below.
</p>
<button onclick="addState()">
Add history state
</button>
<script>
function addState() {
let stateObj = { id: "100" };
window.history.pushState(stateObj,
"x 2", "/x2.html");
}
</script>
</body>
</html>
The URL can be altered by giving the required URL as a string to this method. This will modify the page’s URL without having to reload it.
Output:
Before clicking the button:
After clicking the button:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn