How to Convert Array to JSON in JavaScript
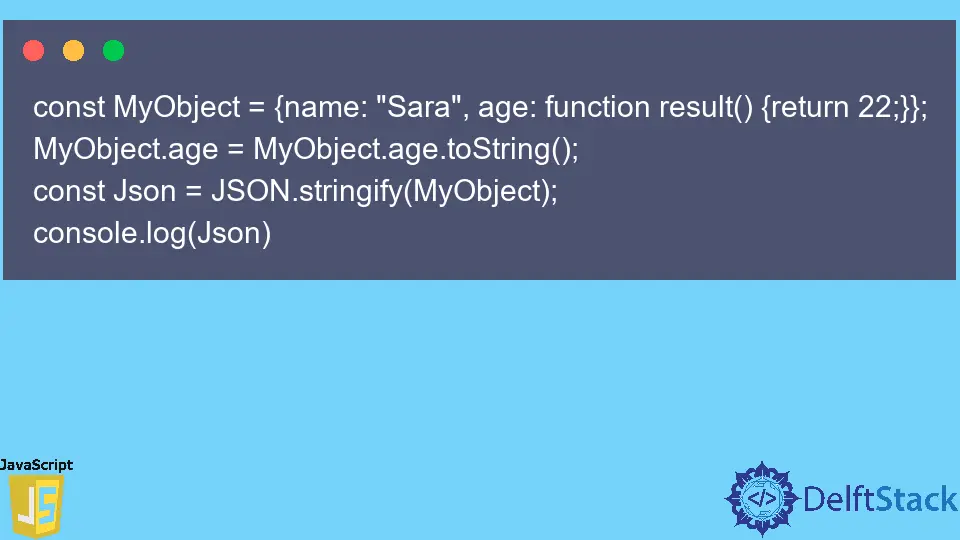
This tutorial will discuss converting an array to JSON using the JSON.stringify()
function in JavaScript.
Convert an Array to JSON Using the JSON.stringify()
Function in JavaScript
We use JSON to send and receive data from a server, and the data should be in a string format. We can convert a JavaScript array to JSON using the JSON.stringify()
function. For example, let’s create a string array and convert it to JSON using the JSON.stringify()
function. See the code below.
const MyArray = ['Banana', 'Apple'];
const JsonArray = JSON.stringify(MyArray);
console.log(JsonArray)
Output:
["Banana","Apple"]
After converting the array to JSON, you can send it to the server without any issues. You can also convert an object containing multiple properties to JSON using the JSON.stringify()
function in JavaScript. For example, let’s create an object with various properties and convert it into JSON using the JSON.stringify()
function in JavaScript. See the code below.
const MyObject = {
name: 'Sara',
age: 22
};
const Json = JSON.stringify(MyObject);
console.log(Json)
Output:
{"name":"Sara","age":22}
If you want to send an object which contains a date function to a server, you can use the JSON.stringify()
function, but it will also convert it into a string. So you have to convert that string into a date object at the receiver. For example, let’s pass a date object into the JSON.stringify()
function and see the result. See the code below.
const MyObject = {
name: 'Sara',
date: new Date()
};
const Json = JSON.stringify(MyObject);
console.log(Json)
Output:
{"name":"Sara","date":"2021-07-17T02:50:10.568Z"}
As you can see in the output, the date has also been converted into a string. If you want to convert an object or array which contains a function, the JSON.stringify()
function will remove the function. So you have to convert the function into a string before converting the object or array into JSON, and at the receiver, you can restore it to a function. For example, let’s convert an object containing a function into JSON using the JSON.stingify()
function. See the code below.
const MyObject = {
name: 'Sara',
age: function result() {
return 22;
}
};
MyObject.age = MyObject.age.toString();
const Json = JSON.stringify(MyObject);
console.log(Json)
Output:
{"name":"Sara","age":"function result() {return 22;}"}
In the above code, we used the toString()
function to convert the function into a string, and then we used the JSON.stringify()
function to convert the object to JSON. We can use the eval()
function to restore the function.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript