How to Append Values to Object in JavaScript
-
Use the
object.assign()
Method to Append Elements to Objects in JavaScript -
Use the
push()
Method to Append Elements to Objects in JavaScript - Use the Spread Operator to Append to Objects in JavaScript
- Conclusion
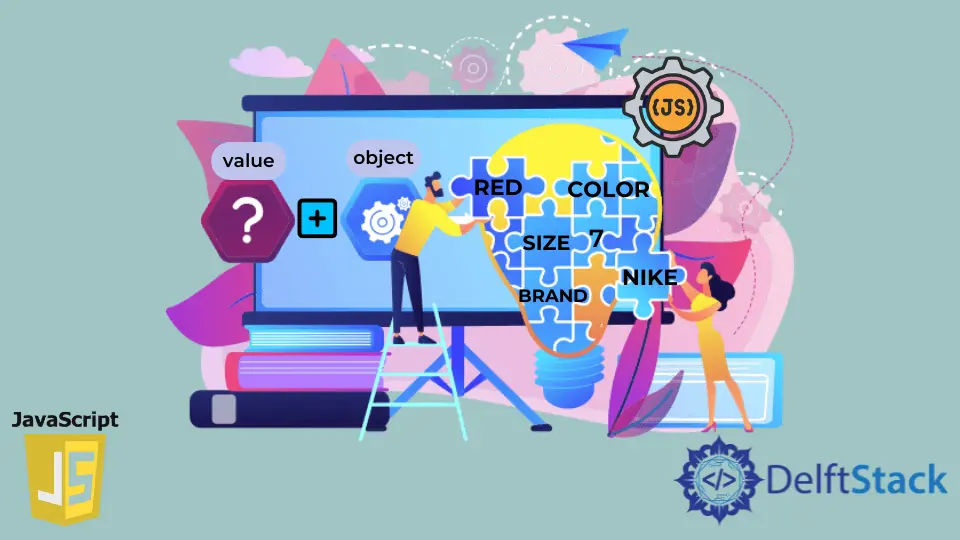
One fundamental operation frequently encountered in programming is appending values to JavaScript objects. This guide focuses on the key ins and outs of JavaScript append to object and will equip you with the knowledge to confidently handle object manipulation in JavaScript.
This tutorial demonstrates how to append values to JavaScript object.
Use the object.assign()
Method to Append Elements to Objects in JavaScript
The Object.assign()
method in JavaScript is used to copy the all the values of enumerable properties from one or more source objects to a target object. It returns the target object after copying the properties from existing objects.
Syntax
Object.assign(target, source1, source2, ...);
target
: The target object to which properties will be copied.
source1, source2
: One or more source objects from which properties will be copied.
Example
let book = {
title: 'JavaScript Essentials'
};
let additionalDetails = {
author: 'Alex Doe',
year: 2023
};
Object.assign(book, additionalDetails);
// Displaying the result
console.log('Book Details:', book);
We define a book
object with an initial property: title
.
We create an additionalDetails
object with more properties: author
and year
.
By using Object.assign()
, we merge additionalDetails
into the book
. The book
is the target and first argument, and additionalDetails
is the source and the second argument.
console.log()
is the function used to display the updated book
object in console.
Output:
Use the push()
Method to Append Elements to Objects in JavaScript
The push()
function in JavaScript is primarily used to add new elements to the end of an array, modifying its length. Its utility shines in object manipulation when accessing an object contains an element of an array as one of its properties.
Syntax
array.push(element1, element2, ..., elementN);
array
: The array to which new elements are being added.
element1, element2, ..., elementN
: These are the elements that will be added to the end of the array. You can add as many elements as you need.
Example
let users = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 }
];
let newUser = { name: 'Charlie', age: 35 };
users.push(newUser);
// Displaying the result
console.log('Updated Users List:', users);
We start with creating users
array containing two objects, each representing once instance of a user.
We define a newUser
object that we intend to assign and to add to the users
array.
The push()
method is then called on the users
array, passing newUser
as the argument. This method adds the value newUser
to the end of the users
array.
Finally, console.log()
prints the updated array, demonstrating the addition of the new user.
Output:
Use the Spread Operator to Append to Objects in JavaScript
The spread operator is used to merge or clone objects in a JavaScript object. It can be used when all elements in an object need to be included in some list.
Syntax
let newObject = { ...originalObject, ...additionalProperties };
originalObject
: The JavaScript object whose properties are to be copied.
additionalProperties
: Additional properties or another object whose properties are to be appended to property above.
Example
let originalObject = {
name: 'John Doe',
age: 28
};
let newInfo = {
occupation: 'Developer',
country: 'USA'
};
let updatedObject = { ...originalObject, ...newInfo };
// Displaying the result
console.log('Updated Object:', updatedObject);
We define a variable originalObject
with properties name
and age
.
Another object, newInfo
, holds additional properties: occupation
and country
.
The updatedObject
is created using the spread operator. It takes all properties from originalObject
and newInfo
and combines them into and creates a new object.
The console.log()
function then outputs the updatedObject
.
Output:
Conclusion
In wrapping up our comprehensive guide on appending values to objects in JavaScript, we’ve explored three key pivotal methods: Object.assign()
, the spread operator
, and the push()
method. Each technique serves a specific purpose in the realm of object and array manipulation.
Object.assign()
is crucial for merging and cloning objects, the spread syntax operator
excels in appending properties while preserving object immutability, and the push()
method is essential for adding elements to arrays.
For developers seeking to expand their JavaScript skills, practicing these methods in various scenarios is key. This could range from basic data handling to complex state management in applications.