How to Format Number With Commas in JavaScript
- Use Regular Expressions to Format Number With Commas in JavaScript
-
Use
Intl.NumberFormat()
to Format Number With Commas in JavaScript -
Use
toLocaleString()
to Format Number With Commas in JavaScript - Conclusion
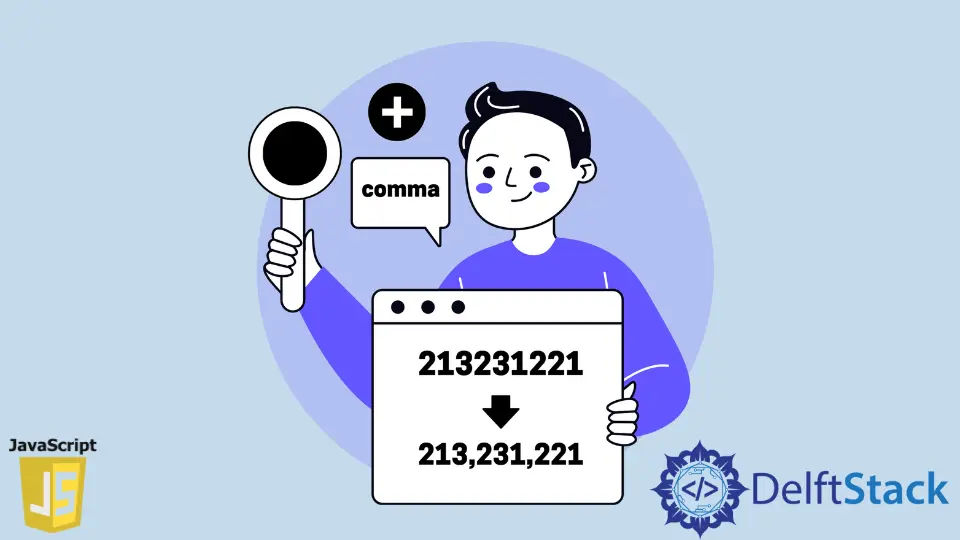
When working with numbers in JavaScript, it’s often helpful to format them with commas for better readability. Whether displaying large monetary values, statistical data, or any numerical information, adding commas to separate thousands, millions, and beyond can greatly enhance the user experience.
Methods for formatting numbers with commas include built-in JavaScript functions like the toLocaleString()
function, the Intl.NumberFormat()
object, and custom functions that leverage string manipulation and regular expressions. We will cover all three methods depending on our use case and the speed/efficiency required to process the given number.
This article will explore different approaches to formatting numbers with commas using JavaScript. Additionally, we will discuss scenarios such as formatting decimal numbers and handling negative values to ensure accurate and aesthetically pleasing number display.
Use Regular Expressions to Format Number With Commas in JavaScript
The regex uses two lookahead assertions:
- One positive lookahead assertion searches for a point in the string containing a group of 3 digits after it.
- One negative lookahead assertion that ensures that the given point has a group size of exactly 3 digits.
Then the replacement expression inserts a comma at that position.
It also takes care of the decimal places by splitting the string before applying the regex expression on the part before the decimal.
const numb = 213231221;
function separator(numb) {
var str = numb.toLocaleString().split('.');
str[0] = str[0].replace(/\B(?=(\d{3})+(?!\d))/g, ',');
return str.join('.');
}
console.log(separator(numb))
Output:
Use Intl.NumberFormat()
to Format Number With Commas in JavaScript
The Intl.NumberFormat()
method is used to represent numbers in language-sensitive formatting and represent currency according to the parameters provided. The parameter provided is called locale
and specifies the format of the number.
For example, The en-IN
locale takes the format of India and the English language. The first comma from the right separates thousands and the rest onwards in terms of hundreds.
We will use the format()
function attached to the object yielded by Intl.NumberFormat()
. This function takes in the number and returns a comma-separated string.
We can use the en-US
locale to achieve a string separated by a thousand.
const givenNumber = 123423134231233423;
internationalNumberFormat = new Intl.NumberFormat('en-US')
console.log(internationalNumberFormat.format(givenNumber))
Output:
Use toLocaleString()
to Format Number With Commas in JavaScript
The toLocaleString()
method returns a string with the language-sensitive representation of a number, and these strings are always separated with the help of commas. Initially, the US format is followed by default to display a number.
It also takes the locale parameters that specify the format of the number.
const givenNumber = 123423134231233423;
console.log(givenNumber.toLocaleString('en-US'))
Output:
Conclusion
Formatting numbers with commas in JavaScript is a valuable technique for enhancing the readability and user-friendliness of numerical data whether you choose to use the built-in toLocaleString()
function, the all-reliable Intl.NumberFormat()
object, or a custom function with regular expressions, both approaches allow you to achieve the desired formatting effect.
First, however, you can go for the easy-to-apply and direct function toLocaleString()
if you are a beginner, as it is easy to comprehend.
By incorporating these methods into your JavaScript code, you can ensure that large numbers are presented clearly and visually appealingly. Consider the specific requirements of your project, such as handling decimals or negative values, and select the appropriate method accordingly.
Formatting numbers with commas will improve your data presentation and create a more enjoyable user experience.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn