How to Create and Parse a 3D Array in JavaScript
- Introduction to Parsing in JavaScript
- Create an Array in JavaScript
- Parse an Array in JavaScript
- Different Types of Arrays in JavaScript
- Create and Parse a 3D Array in JavaScript
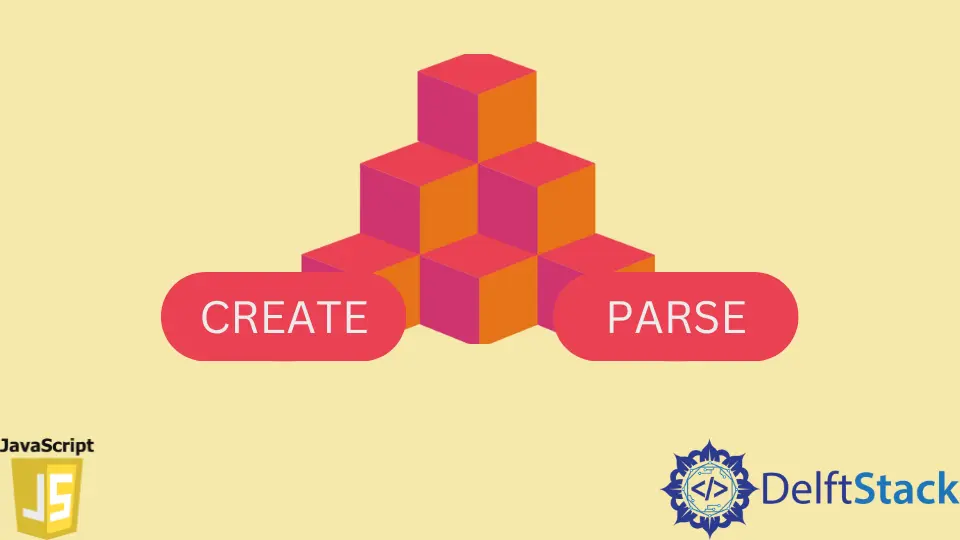
This article addresses the following.
- Introduction to parsing
- How to create an array in JavaScript?
- How to do parsing on an array in JavaScript?
- Different types of arrays
- Creating and parsing a 3D array in JavaScript
Introduction to Parsing in JavaScript
Parsing evaluates and changes a program into an internal format that a runtime environment can execute, such as the JavaScript engine found in browsers. A parser analyses our code line by line and checks for mistakes, stopping execution if any are found.
The parser generates an abstract syntax tree converted to machine code and output if the code doesn’t have syntax errors. The execution context gives the environment where our code executes and is defined as one of the following.
- Global: Default environment where code is executed the first time.
- Local/Function: When the code within the function gets executed.
Eval
: when the text within theeval
gets performed
JavaScript is parsed at compilation time or when the parser is called, such as during a method call. The code below tells you how do you parse an object in JavaScript.
var person = 'XYZ';
const func1 = function() {
let address = 'Pakistan';
func2();
console.log(`${person} ${address}`);
} const func2 = function() {
let designation = 'Software Engineer';
let address = 'America'
console.log(`${person} ${designation} ${address}`);
} func1();
console.log(window.person === person)
The above code is an example of the global context of the environment. Let’s look at the preceding example again.
The variable person
is declared in the global context, and the global context is used to run code that isn’t part of any function. Whenever a function is called, a new execution context is produced and put on top of the existing context, forming the execution stack.
You can access this code through this link.
Create an Array in JavaScript
Let’s first see what an array is. An array can contain numerous values under a single name, and the items can be accessed by referring to an index number.
Creating an array is shown below.
<html>
<body>
<p id="data"></p>
</body>
</html>
JavaScript code for the above HTML file is below.
const cars = ['Saab', 'Volvo', 'BMW'];
document.getElementById('data').innerHTML = cars;
Execution of this code is given in this link.
Parse an Array in JavaScript
var arr = ['car', 'bike', 'truck'];
arr = arr.map(Vehicles => parseInt(Vehicles));
console.log(arr);
The above code gives parseint
array JavaScript. But parsing in JavaScript is usually done by using JSON.parse()
in JavaScript. JSON
is a format standard.
Parsing JSON entails translating the data into whichever language you’re working with at the time. When JSON
is parsed, a text is transformed into a JSON
object according to the specification, which can be utilized in any way you want.
An example of JavaScript parse JSON
is below.
var jsonPerson = '{"first_name":"XYZ", "age":18}';
var personObject = JSON.parse(jsonPerson);
Here parse JSON
string is converted into a JavaScript object. The following example can show the JavaScript object.
const json = '{ "fruit": "pineapple", "Pineapple": 10 }';
const obj = JSON.parse(json);
console.log(obj.fruit, obj.Pineapple);
Different Types of Arrays in JavaScript
There exist two types of arrays in JavaScript.
- Multi-dimensional arrays
- One-dimensional arrays
Multi-Dimensional Array in JavaScript
In JavaScript, multi-dimensional arrays are not immediately available. Therefore, a multi-dimensional array must be generated by employing another one-dimensional array if you wish to utilize anything that serves as a multi-dimensional array.
As a result, multi-dimensional arrays in JavaScript are called arrays inside arrays. You must nest certain arrays within an array for the entire thing to function as a multi-dimensional array.
The array into which the other arrays will be inserted is used as a multi-dimensional array in our code. A multi-dimensional array is defined similarly as a one-dimensional array is defined.
One-Dimensional Array in JavaScript
A linear array is also known as a one-dimensional JavaScript array. There is only one row or column in it.
var arr = []; // Empty One-Dimensional array
var arr1 =
['A', 'B', 'C', 'D'] // One-Dimensional array containing some alphabets
var arr1 = [1, 2, 3, 4, 5] // One-Dimensional array containing some digits
Now coming back towards the multi-dimensional array.
let arr = [['ABC', 18], ['XYZ', 19], ['JKL', 20]];
console.log(arr[0]);
console.log(arr[0][0]);
console.log(arr[2][1]);
Think of a multi-dimensional array as a table with three rows and two columns. When (arr[0] [0])
is called in console.log
it will give ABC
.
When (arr[1] [0])
is called in console.log
, it will give JKL
and so on. Link for the above code to run and play with.
Column 1 | Column 2 | |
---|---|---|
Row 1 | ABC, (arr [0] [0]) |
18 (arr [0] [1]) |
Row 2 | XYZ, (arr[1] [0]) |
19 (arr [1] [1]) |
Row 3 | JKL, (arr[2] [0]) |
20 (arr [2] [1]) |
Create and Parse a 3D Array in JavaScript
A 3D array is more sophisticated, and you should think twice about using it on the web because it has a restricted reach. With 1D or 2D arrays, 99 percent of issues can be addressed.
A 3D array, on the other hand, would be like below.
var myArr = new Array();
myArr[0] = new Array();
myArr[0][0] = new Array()
myArr[0][0][0] = 'Howdy';
myArr[0][0][1] = 'pardner';
alert(myArr[0][0][1]);
alert(myArr[0][0][0]);
Link is attached here for the above code.
In this article, a little walkthrough of concepts of parsing and arrays in JavaScript is discussed. Then different types of arrays and how to create and parse them in JavaScript are explained.
In the end, concepts of multi-dimensional arrays and 3D arrays are elaborated, and then creating and parsing of these arrays is explained with code segments.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript