Integer Division in JavaScript
-
Perform Integer Division in JavaScript Using the
math.floor()
Function -
Perform Integer Division in JavaScript Using the
math.trunc()
Function - Perform Integer Division in JavaScript Using the Bitwise Operators
-
Perform Integer Division in JavaScript Using the
parseInt()
Function - Conclusion
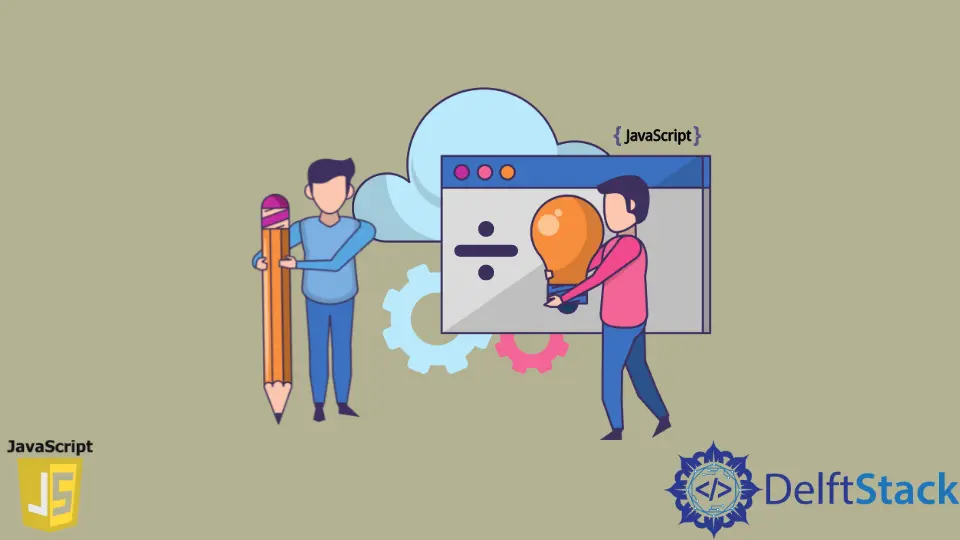
In JavaScript, performing integer division involves calculating the quotient and remainder when dividing one integer by another. Understanding how integer division works in JavaScript is crucial for accurately manipulating numbers and implementing various mathematical operations.
When dividing two integers, JavaScript offers different ways to handle the resulting quotient and remainder. Understanding the differences between these approaches is important to ensure accurate calculations.
This article will explore different approaches to performing integer division in JavaScript.
Perform Integer Division in JavaScript Using the math.floor()
Function
In JavaScript, we can divide two variables easily, resulting in floating-point numbers, but if we want to get the quotient and remainder of the division, we can use the Math
library functions. One such function from the math
library is the math.floor()
function.
The Math.floor()
function rounds a number downwards to the nearest integer. By applying this function to the result of standard division, we can obtain the integer quotient.
For example, let’s find the quotient and the remainder of 13 divided by 5. See the code below.
var a = 13;
var b = 5;
var quo = Math.floor(a / b);
var rem = a % b;
console.log('Quotient = ', quo, 'Remainder = ', rem);
Output:
Note that the
Math
library will fail if the numbers are too large or we are dealing with negative numbers.
Perform Integer Division in JavaScript Using the math.trunc()
Function
You would know now that the math.floor()
function rounds down to the closest integer. The math.trunc()
function, on the other hand, returns the integer part of the given number while getting rid of any fractional digits that might be present.
The advantage that this method holds is that you can use the
Math.trunc()
function in cases when you have to handle large numbers as compared to themath.floor()
function.
Consider the following code.
var a = 13;
var b = 5;
var quo = Math.trunc(a / b);
var rem = a % b;
console.log('Quotient = ', quo, 'Remainder = ', rem)
Output:
The
Math.floor()
function will fail in the case of negative numbers, butMath.trunc()
won’t fail in the case of negative numbers.
Perform Integer Division in JavaScript Using the Bitwise Operators
In JavaScript, we can find the remainder and the quotient of a division with the help of the bitwise operators. For example, the bitwise NOT
~
or bitwise OR
|
can be utilized to get the quotient of a division, which works by converting the floating-point number to an integer.
Moreover, we can use the %
character to get the remainder. For example, let’s find the quotient and the remainder of 13 divided by 5. See the code below.
var a = 13;
var b = 5;
var quo = ~~(a / b);
var rem = a % b;
console.log('Quotient = ', quo, 'Remainder = ', rem);
Output:
As you can see, the output is the same as the above method. The performance of bitwise operators is greater than the Math
library functions, but the capacity to handle large numbers is less.
So, if you have small numbers, you can use the bitwise operators; otherwise, use the Math
library functions like the math.floor
function and the math.trunc
function.
The bitwise operators can also handle negative numbers. You can also use the parseInt()
function to convert a floating-point number to an integer.
If you don’t want the hassle of using any functions, you can utilize a straightforward formula with the remainder operator %
, as shown below.
var a = 13;
var b = 5;
var rem = a % b;
var quo = (a - rem) / b;
console.log('Quotient = ', quo, 'Remainder = ', rem);
Output:
Perform Integer Division in JavaScript Using the parseInt()
Function
The parseInt()
function parses a string and returns an integer. The integer quotient can be extracted straightforwardly by converting the result of the standard division to a string and then parsing it using the parseInt()
function.
Consider the following example code.
var a = 13;
var b = 5;
var quo = parseInt(a / b);
var rem = a % b;
console.log('Quotient = ', quo, 'Remainder = ', rem)
Output:
Conclusion
JavaScript provides a flexible range of options for integer division, allowing you to perform precise calculations in your JavaScript projects. In this article, we explored different approaches to perform integer division, including using the Math.floor()
function, the math.trunc()
function, the Bitwise Operators, and the parseInt()
function.
By employing these methods, you can accurately calculate the quotient without the remainder, essential for various mathematical operations and algorithm implementations. Remember to choose the appropriate method based on your needs and the desired outcome.