Case Insensitive String Comparison in JavaScript
-
Use the
toUpperCase()
/toLowerCase()
String Methods to Do Case Insenstive Comparsion in JavaScript -
Use the
localeCompare()
String Method to Do Case Insensitive Comparison in JavaScript -
Use a Regular Expression Method
RegExp
to Do Case Insensitive Comparison in JavaScript
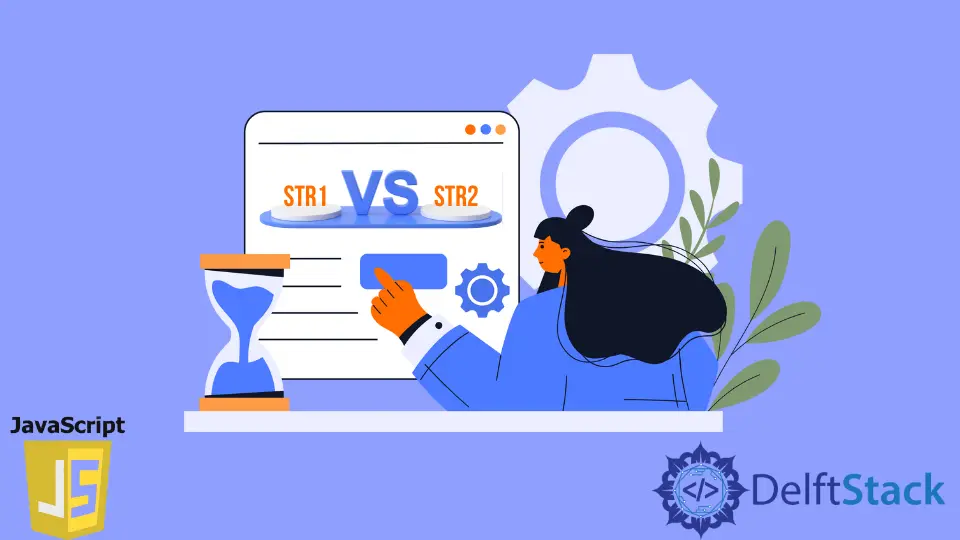
This article explains how to perform case-insensitive string comparison in JavaScript.
Use the toUpperCase()
/toLowerCase()
String Methods to Do Case Insenstive Comparsion in JavaScript
In the toUpperCase()
method, the strings are first converted to uppercase or lowercase and then compared with the triple equals operator ===
.
Here’s an example.
var stringA = 'This is a JavaScript tutorial';
var stringB = 'THIS is A javascript TUTORIAL';
if (stringA.toUpperCase() === stringB.toUpperCase()) {
alert('The strings are equal.')
} else {
alert('The strings are NOT equal.')
}
Output:
The strings are equal.
Alternatively, you can also convert the strings to lowercase using the toLowercase()
string method as in the following example.
var stringA = 'This is a JavaScript tutorial';
var stringB = 'THESE are javascript TUTORIALS';
if (stringA.toLowerCase() === stringB.toLowerCase()) {
alert('The strings are equal.')
} else {
alert('The strings are NOT equal.')
}
Output:
The strings are NOT equal
It is worth noting, however, that the uppercase method is preferred. It is because some characters in the alphabet cannot make a round trip when converted to lowercase. This essentially means that they may be unable to be converted from one locale to another in a way that represents that data characters accurately.
Use the localeCompare()
String Method to Do Case Insensitive Comparison in JavaScript
While the method above gives an easy answer, it may not work well where the strings include Unicode characters. The localeCompare
string method can take care of this. It is used with the sensitivity: 'base'
option to capture all Unicode characters. For example, we can this in an if()
statement to get results when the strings in question are equal and when they are not:
if ('javascript'.localeCompare('JavaScrpt', undefined, {sensitivity: 'base'}) ==
0) {
alert('The strings are equal')
} else {
alert('The strings are different.')
}
Output:
The strings are different.
Use a Regular Expression Method RegExp
to Do Case Insensitive Comparison in JavaScript
In this method, we use the RegExp
pattern with the test()
method to do a comparison between case insensitive strings. For instance,
const strA = 'This is a case sensitive comparison';
const strB = 'This is a CASE SENSITIVE comparison';
const regex = new RegExp(strA, 'gi');
const comparison = regex.test(strB)
if (comparison) {
alert('Similar strings');
}
else {
alert('Different strings');
}
Output:
Similar strings