How to Empty an Array in JavaScript
- Set Array to New Array of Size Zero
-
Use the
length
Property to Empty an Array in JavaScript -
Use the
splice()
Method to Empty an Array in JavaScript -
Use the
pop()
Method to Empty an Array in JavaScript - Modify native methods to empty an array in JavaScript
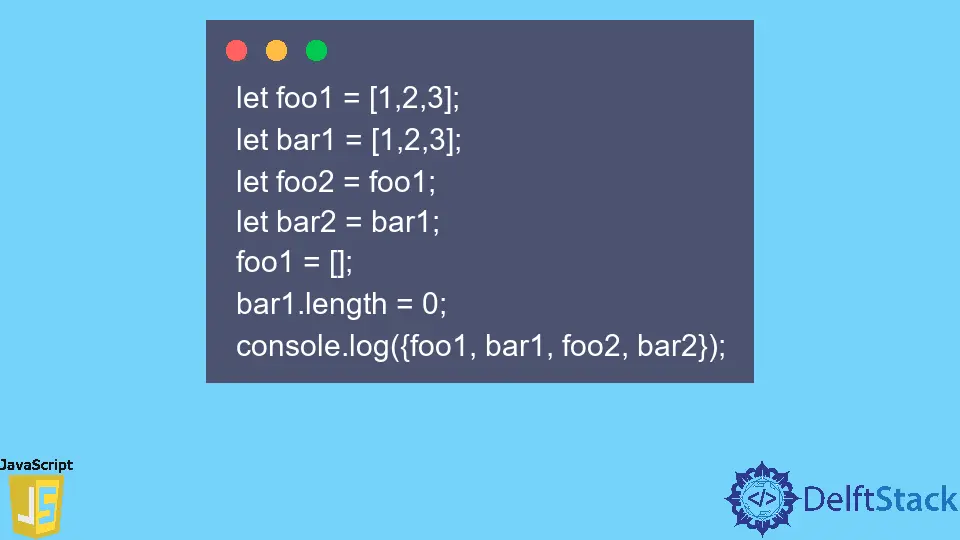
The array is a collection of items stored in a convenient, indexed set. What if we want to empty an array that contains multiply items?
This tutorial introduces different methods of how to empty an array in JavaScript.
Set Array to New Array of Size Zero
It is the fastest way. This will set the array to a new empty array.
let Arr = [1, 2, 3, 4];
Arr = [];
It is straightforward if you don’t have any reference from other places to the original array. These references won’t be updated if you do so, and those places will continue to use the old array. It means that references to the previous array contents are still kept in memory, leading to memory leaks.
Only use this if you reference the array by its original variable Arr
.
Below code example shows the issue you can encounter when using this method:
let Arr1 = [1, 2, 3, 4, 5, 6];
let Arr2 = Arr1;
Arr1 = [];
console.log({Arr1, Arr2});
Output:
{
Arr1: [],
Arr2: [1, 2, 3, 4, 5, 6]
}
Use the length
Property to Empty an Array in JavaScript
It clears the existing array by setting its length to 0. The length property of an array is a read/write property, so it also works when using the strict mode in ECMAScript 5.
Arr1.length = 0
This way has an advantage because it deletes everything in the array, which does impact other references. If we have two references to the same array, we delete the array’s contents using Arr1.length = 0
, both references will now point to the same empty array.
Example:
let foo1 = [1, 2, 3];
let bar1 = [1, 2, 3];
let foo2 = foo1;
let bar2 = bar1;
foo1 = [];
bar1.length = 0;
console.log({foo1, bar1, foo2, bar2});
Output:
{
bar1: [],
bar2: [circular object Array],
foo1: [],
foo2: [1, 2, 3]
}
Use the splice()
Method to Empty an Array in JavaScript
The array.splice()
method is a built-in method in JavaScript, which is used to add/remove items to/from an array and returns the removed items.
let Arr1 = ['Tomato', 'Letcuce', 'Spinash', 'Cucumber'];
Arr1.splice(2, 0, 'Lemon', 'Kiwi')
console.log(Arr1);
Output:
["Tomato", "Letcuce", "Lemon", "Kiwi", "Spinash", "Cucumber"]
To empty the array:
Arr1.splice(0, Arr1.length);
console.log(Arr1);
Output:
[]
It removes all elements from the array and will clean the original array.
Use the pop()
Method to Empty an Array in JavaScript
The pop()
method removes the last element from an array and returns that element. And it will change the length of the array.
let vegetables = ['broccoli', 'cauliflower', 'cabbage', 'kale', 'tomato'];
console.log(vegetables.pop());
console.log(vegetables);
Output:
tomato
(4) ["broccoli", "cauliflower", "cabbage", "kale"]
We loop on the array, pop the array element on every loop, and finally get the empty array.
let Arr1 = [1, 2, 3, 4, 5];
for (let i = Arr1.length; i > 0; i--) {
Arr1.pop();
}
console.log(Arr1);
Output:
[]
This solution is not very concise, and it is also the slowest solution.
Modify native methods to empty an array in JavaScript
The last technique in this list can be used with all the methods above; JavaScript allows us to modify and use native methods to extend their capabilities. We can use the Array.proptotype.remove
function to modify the process.
In the example below, we use the splice()
method, which we utilized in the previous example. Now, we can use the process by calling it like the arr.remove()
function.
var arr = [1, 2, 3, 4, 5, 6];
Array.prototype.remove = Array.prototype.remove || function() {
this.splice(0, this.length);
};
arr.remove();
console.log(arr);
Output:
[]
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript