How to Compare Two Dates in JavaScript
- JavaScript Compare Two Dates With Comparison Operators
-
JavaScript Compare Two Dates With the
getTime()
Method -
JavaScript Compare Two Dates With the
valueOf()
Method -
JavaScript Compare Two Dates With the
Number()
Function -
JavaScript Compare Two Dates With the Plus Unary Operator
+
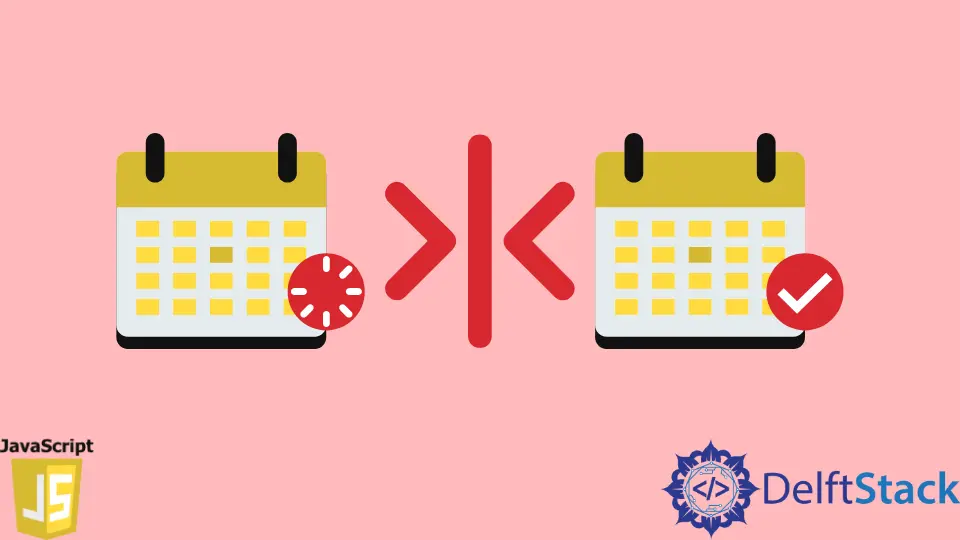
JavaScript has the built-in comparison system for dates, which makes it so easy to make the comparison.
This tutorial introduces different methods of how to compare two dates in JavaScript. Every method will have an example of codes, which you can run on your own machine.
JavaScript Compare Two Dates With Comparison Operators
We can directly compare two dates in JavaScript with comparison operators like <
, <=
, >
and >=
.
var date1 = new Date('2020-10-23');
var date2 = new Date('2020-10-22');
console.log(date1 > date2);
console.log(date1 >= date2);
console.log(date1 < date2);
console.log(date1 <= date2);
Output:
true
true
false
false
Date
objects directly in JavaScript. Because two different objects in JavaScript are never equal both in strict and abstract level. See the example below.let date1 = new Date();
let date2 = new Date(date1);
console.log(date1 == date2);
console.log(date1 === date2);
console.log(date1 != date2);
console.log(date1 !== date2);
Output:
false
false
true
true
We can check the equality of two dates with the following method.
JavaScript Compare Two Dates With the getTime()
Method
We convert two dates into numeric values corresponding to their time using the getTime()
method, and then we can compare two of them directly.
let date1 = new Date(2019, 08, 07, 11, 45, 55);
let date2 = new Date(2019, 08, 03, 11, 45, 55);
if (date1.getTime() < date2.getTime())
document.write('date1 is lesser than date2');
else if (date1.getTime() > date2.getTime())
document.write('date1 is greater than date2');
else
document.write('both are equal');
Output:
date1 is lesser than date2
The getTime()
method could check the equality of two dates in JavaScript.
let date1 = new Date();
let date2 = new Date(date1);
if (date1.getTime() == date2.getTime()) document.write('Two dates are equal.');
if (date1.getTime() === date2.getTime()) document.write('Two dates are equal.');
Output:
Two dates are equal.Two dates are equal.
JavaScript Compare Two Dates With the valueOf()
Method
The valueOf()
method of the Date
object works similar to the getTime()
method. It converts the Date
object into numeric value.
let date1 = new Date(2019, 08, 07, 11, 45, 55);
let date2 = new Date(2019, 08, 07, 11, 45, 55);
if (date1.valueOf() < date2.valueOf())
document.write('date1 is lesser than date2');
else if (date1.valueOf() > date2.valueOf())
document.write('date1 is greater than date2');
else if (date1.valueOf() === date2.valueOf())
document.write('both are equal');
console.log(date1.valueOf() === date2.valueOf())
Both getTime()
and valueOf()
return the number of milliseconds since January 1, 1970, 00:00 UTC.
JavaScript Compare Two Dates With the Number()
Function
The Number()
function converts the Date
object to a number representing the object’s value in Java. It returns NaN if the object can not be converted to a legal number.
let date1 = new Date(2019, 08, 07, 11, 45, 55);
let date2 = new Date(2019, 08, 07, 11, 45, 55);
console.log(Number(date1) === Number(date2))
console.log(Number(date1) == Number(date2))
console.log(Number(date1) < Number(date2))
console.log(Number(date1) > Number(date2))
JavaScript Compare Two Dates With the Plus Unary Operator +
A unary operator takes a single argument and operates on this argument or operand. JavaScript has many unary operators. Here we use the unary plus +
operator, which tries to convert the argument to a number.
let date1 = new Date(2019, 08, 07, 11, 45, 55);
let date2 = new Date(2019, 08, 07, 11, 45, 55);
console.log(+date1 === +date2)
console.log(+date1 == +date2)
console.log(+date1 < +date2)
console.log(+date1 > +date2)
Both Number
function and unary +
operator call the valueOf()
methods behind the scenes.