How to Capitalize the First Letter of a String in JavaScript
-
Use
toUpperCase()
andslice()
Methods to Capitalize the First Letter in JavaScript -
Use
toUpperCase()
WithcharAt()
to Capitalize the First Letter in JavaScript -
Use
toUpperCase()
andreplace()
to Capitalize the First Letter in JavaScript
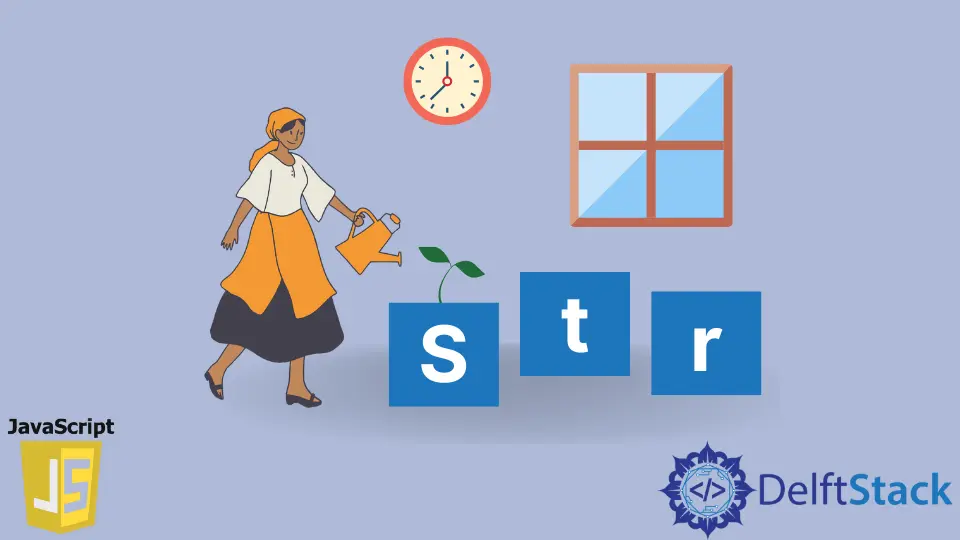
There is a number of ways to capitalize the first letter of the string in JavaScript.
For example:
"this is an example"
->"This is an example"
"the Atlantic Ocean"
->"The Atlantic Ocean"
Use toUpperCase()
and slice()
Methods to Capitalize the First Letter in JavaScript
The toUpperCase()
method transforms all letters in a string to uppercase; we will use it in combination with other JavaScript functions to reach our desired goal.
The slice(start,end)
method extracts a section of a string and returns it as a new string.
start
is the required parameter. It is the position where to begin slice string.
end
is optional. It is the position where to end slice string. If omitted, slice()
finishes at the end of the string.
Example:
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body style = "text-align:center;">
<input id = "input" type="text" name="input"/>
<button onclick="capitalizeString()">
Capitalize
</button>
<h3 id = "modified-string" >
</h3>
<script>
function capitalizeString() {
let input = document.getElementById("input");
let headingElement = document.getElementById("modified-string");
let string = input.value;
headingElement.innerHTML = string[0].toUpperCase() +
string.slice(1);
}
</script>
</body>
</html>
Output:
Another Example:
// shortened version
function capitalize(sentence) {
return sentence && sentence[0].toUpperCase() + sentence.slice(1);
}
Use toUpperCase()
With charAt()
to Capitalize the First Letter in JavaScript
The charAt()
method returns the character from the specified index in a string. The index starts at 0.
Example:
// We will use the same html above
function capitalizeString() {
let input = document.getElementById('input');
let headingElement = document.getElementById('modified-string');
let string = input.value;
headingElement.innerHTML = string.charAt(0).toUpperCase() + string.slice(1);
;
}
Use toUpperCase()
and replace()
to Capitalize the First Letter in JavaScript
The replace()
is an inbuilt method in JavaScript which searches the given string for a specified value or a regular expression pattern, replaces it, and returns a new string.
JavaScript replace()
Syntax:
string.replace(paramA, paramB)
Here, the paramA
is a value or regular expression, and paramB
is a value to replace paramA
with.
Quick Example on replace()
Method:
function replaceFunction() {
let str = 'Google Event';
let res = str.replace('Google', 'Microsoft');
console.log(res)
}
replaceFunction();
function replaceAllFunction() {
let str = 'Google Event will be in Headquarters of Google';
let res = str.replace(/Google/g, 'Microsoft');
console.log(res)
}
replaceFunction();
replace()
method only replaces the first instance by default. We should use the regular expression global (g
) modifier to replace all occurrences.Example:
// We will use the same html above
function capitalizeString() {
let input = document.getElementById('input');
let headingElement = document.getElementById('modified-string');
let string = input.value;
headingElement.innerHTML = string.replace(/^./, string[0].toUpperCase());
}
/^./
represents the first letter of the string. Read more about regular expressions in the RegExp Tutorial and the RegExp Object Reference.