Object Inside an Object in JavaScript
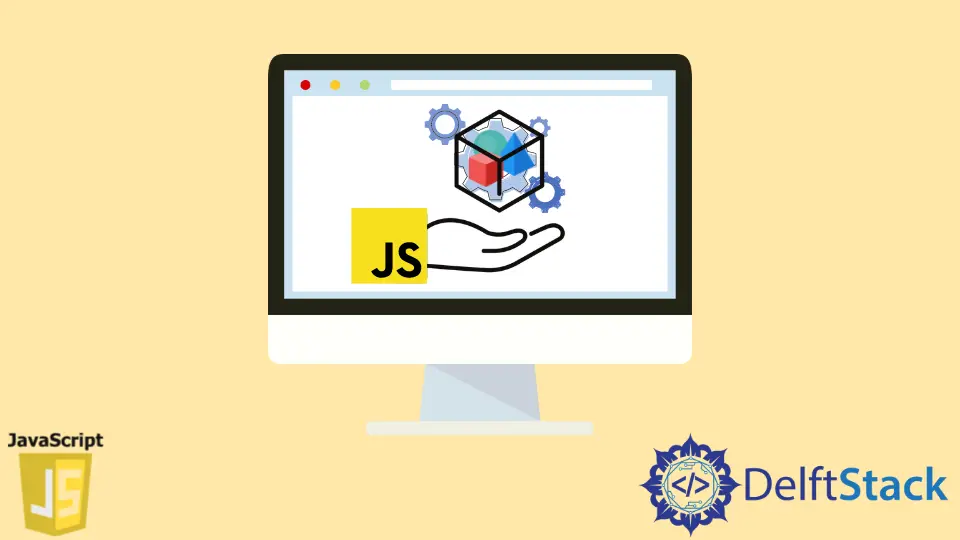
This tutorial article will explain how we can create multiple objects inside another object in JavaScript.
A JavaScript Object is a collection of Key-Value pairs, and nested objects are objects that have other objects inside them as their property. Nesting is a widely used practice in programming as it provides the code with more enhanced details.
Each object has its own properties or keys, which are used to store the details of the object. A comma separates each property or key.
Let’s have a look at how we create an object and properties in Javascript.
const layer0 = {
layer1Item1: 'Layer 1 Item 1 Text',
layer1Item2: 'Layer 1 Item 2 text',
layer1Item3: 'Layer 1 Item 3 Text',
layer1Item4: 'Layer 1 Item 4 text'
}
Here, if you can see, const layer0
is the name of the object. This is how we create an object in Javascript. Now within the object, as you can see, layer1Item1
, layer1Item2
, layer1Item3
and layer1Item4
are the properties of the object. These properties must be unique and the differentiating factors that distinguish an object from another.
Now, if you want to create an object within another object, the inner object is created as a property of the outer one, and this inner object can only be accessed using the outer object.
Create Nested Objects in JavaScript
const layer0 = {
layer1Item1: 'Layer 1 Item 1 Text',
layer1Item2: {
layer2Item1: 'Layer 2 Item 2 Text',
layer2Item2: false
}
};
In this example, layer1Item2
is a new object inside of another object. However, layer1Item1
is a property of the object, and layer1Item2
is an object. Both look similar because the newly created object is also created as a property of the outer object layer0
.
Now, if you want to access the inner object, you will write the name of the outer object, and after a dot, you will write the name of the inner object.
layer0.layer1Item2
And if you want to access some property within the inner object, the code will be:
layer0.layer1Item2.layer2Item1
Multiple Nesting in Javascript
There is no limit of nesting in Javascript. You can make n number of hierarchies. The method of accessing the objects would be the same for accessing the inner ones, as discussed in the previous example.
const layer0 = {
layer1Item1: 'Layer 1 Item 1 Text',
layer1Item2: {
layer2Item1: 'Layer 2 Item 2 Text',
layer2Item2: false,
layer2Item3: {layer3Item1: 'Layer 3 Item 2 Text'}
}
};
In this example, there are 3 objects created, layer0
, layer1
, and layer2
. The layer2
object is inside layer1
, and layer1
is inside layer0
. There are 3 layers or hierarchies in this example. Now, layer2
is written as a property of layer1
.
To access the properties of the layer2
, we will write the following code.
layer0.layer1Item2.layer2Item1.layer3Item1
Now, to add a new property to an object in the following code:
let layer0 = {
layer1Item1: 'Layer 1 Item 1 Text',
layer1Item2: 'Layer 1 Item 2 text',
layer1Item3: 'Layer 1 Item 3 Text',
layer1Item4: 'Layer 1 Item 4 text'
}
we will simply attach the object name with the object property with a dot and assign it a value like below:
layer0.layer1Item5 = 'New Item created';
Now the resultant object will look like the following:
{
layer1Item1: 'Layer 1 Item 1 Text', layer1Item2: 'Layer 1 Item 2 text',
layer1Item3: 'Layer 1 Item 3 Text', layer1Item4: 'Layer 1 Item 4 text',
layer1Item5: 'New Item Created'
}
If you want to delete any property from the object, it is straightforward to understand. We use the delete
keyword alongside the object name attached with the object property, separated with a dot you want to delete. Look at the following example:
Consider we have the following object.
let layer0 = {
layer1Item1: 'Layer 1 Item 1 Text',
layer1Item2: 'Layer 1 Item 2 text',
layer1Item3: 'Layer 1 Item 3 Text',
layer1Item4: 'Layer 1 Item 4 text'
}
And we want to delete the layer1Item4 property; we will write the following code.
delete layer0.layer1Item4;
Now the object will look like as below.
{
layer1Item1: 'Layer 1 Item 1 Text', layer1Item2: 'Layer 1 Item 2 text',
layer1Item3: 'Layer 1 Item 3 Text',
}