How to Check if Key Exists in Object in JavaScript
- Use the Element Direct Access Method to Check if the Object Key Exists in JavaScript
-
Use the
in
Operator to Check if the Object Key Exists or Not in JavaScript -
Use the
hasOwnProperty
Method to Check if the Object Key Exists or Not in JavaScript -
Use the
underscore
Library to Check if the Object Key Exists or Not in JavaScript
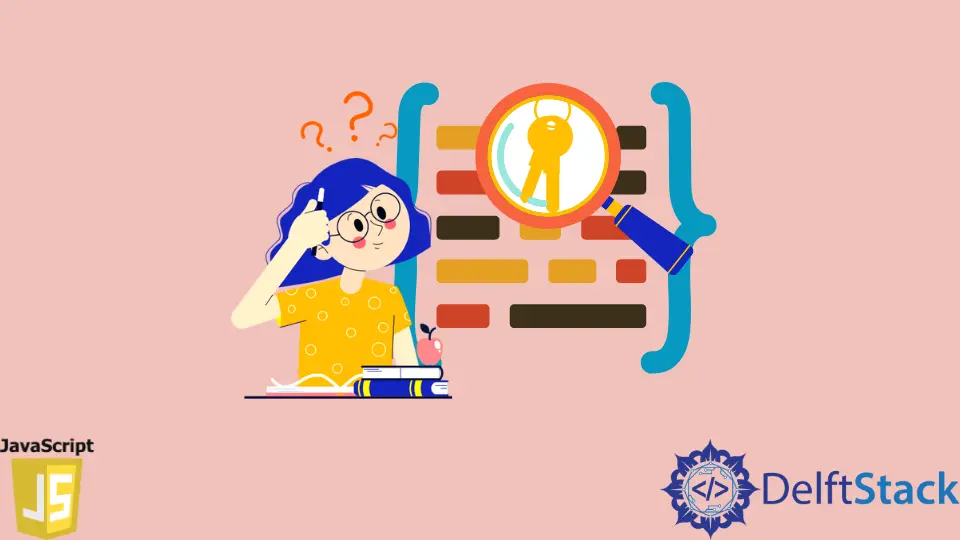
In this tutorial, we will learn how to check if the key exists in JavaScript using multiple ways.
Use the Element Direct Access Method to Check if the Object Key Exists in JavaScript
If a key exists, it should not return undefined
. To check if it returns undefined
or not, we have direct access to the keys, and it can be done in two styles, the object
style, and the brackets access style.
In the following example, we will show how to check if the key exists by direct access to the key using the brackets style.
let myObject = {'mykey1': 'My Value 1', 'mykey2': 'My Value 2'};
function isKeyExists(obj, key) {
if (obj[key] == undefined) {
return false;
} else {
return true;
}
}
let result0 = isKeyExists(myObject, 'mykey0')
console.log('Check for the non-existing key, is key exists > ' + result0)
let result1 = isKeyExists(myObject, 'mykey1')
console.log('Check for the existing key, is key exists > ' + result1)
Output:
Check for the non-existing key, is key exists > false
Check for the existing key, is key exists > true
The Other style to check for the key by direct access is using the object style. In the following example, we can see how to check for that key existence in JavaScript.
let myObject = {mykey1: 'My Value 1', mykey2: 'My Value 2'};
let result = myObject.mykey0 != undefined
console.log('Is myKey0 exists ? ' + result)
let result1 = myObject.mykey1 != undefined
console.log('Is myKey1 exists ? ' + result1)
Output:
Is myKey0 exists ? false
Is myKey1 exists ? true
One of the drawbacks of checking for the undefined
using direct key access is that the key’s value can be equal to undefined
. Let’s see the following example to show the issue with that method.
let myObject = {mykey0: undefined, mykey1: 'My Value 1'};
let result = myObject.mykey0 != undefined
console.log('Is myKey0 exists? ' + result)
let result1 = myObject.mykey1 != undefined
console.log('Is myKey1 exists? ' + result1)
Output:
Is myKey0 exists? false
Is myKey1 exists? true
The above case’s solution is never to assign undefined
to a property when you want to initialize it with an undefined value, but initialize it with null
instead.
If we insist on using the undefined
value to initialize the keys, we should use the in
operator, the hasOwnProperty
method, or the underscore
library _.has
method.
Use the in
Operator to Check if the Object Key Exists or Not in JavaScript
The in
operator is simply used to return false
if the key was not found in the target object and returns true
if found.
let myObject =
{favoriteDish: 'Spaghetti', language: 'English'}
function isKeyExists(obj, key) {
return key in obj;
}
console.log(
'Does language key exists? ' + isKeyExists(myObject, 'language'))
console.log(
'Does nationality key exists? ' + isKeyExists(myObject, 'nationality'))
Output:
Does language key exists? true
Does nationality key exists? false
Use the hasOwnProperty
Method to Check if the Object Key Exists or Not in JavaScript
Another way to check if the object contains a specific property key or not is to use the hasOwnProperty
method. In the following example, we will show how we can use the hasOwnProperty
method.
let myObject =
{favoriteDish: 'Spaghetti', language: 'English'}
function isKeyExists(obj, key) {
return obj.hasOwnProperty(key);
}
console.log(
'Does the object have language key? ' +
isKeyExists(myObject, 'language'))
console.log(
'Does the object have nationality key? ' +
isKeyExists(myObject, 'nationality'))
Output:
Does the object have language key? true
Does the object have nationality key? false
Use the underscore
Library to Check if the Object Key Exists or Not in JavaScript
If we are already using any of the underscore
library methods, we can use the _.has()
method, as it returns true
if that object has the provided key and returns false
if not.
let myObject =
{favoriteDish: 'Spaghetti', language: 'English'}
console.log(
'Check using underscore library if the object has language key? ' +
_.has(myObject, 'language'))
console.log(
'Check using underscore library if the object has nationality key? ' +
_.has(myObject, 'nationality'))
Output:
Check using underscore library if the object has language key? true
Check using underscore library if the object has nationality key? false
We can import the library from here.