The XOR Operator in Java
- Understanding the XOR Operator
- Using the XOR Operator for Simple Calculations
- Swapping Two Numbers Without a Temporary Variable
- Checking Even or Odd Numbers
- Conclusion
- FAQ
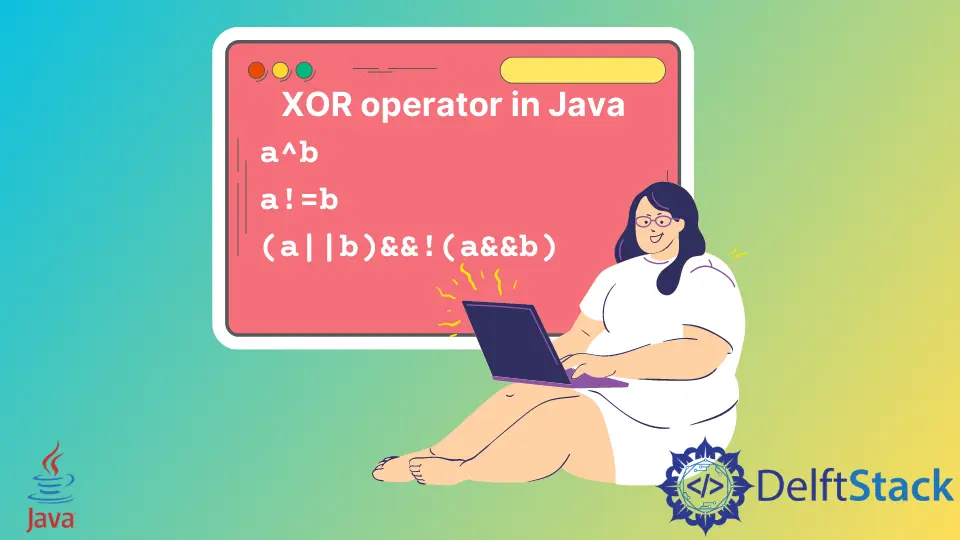
The XOR operator, often represented by the caret symbol (^), is a fundamental part of Java’s bitwise operations. It stands for “exclusive or” and is a powerful tool for performing operations on binary numbers. By understanding how to use the XOR operator in Java, developers can accomplish a variety of tasks, from simple logical comparisons to more complex algorithms.
This article will delve into the methods for using the XOR operator effectively in Java, providing clear examples and explanations to enhance your coding skills. Whether you’re a beginner or an experienced programmer, mastering the XOR operator can greatly improve your problem-solving abilities.
Understanding the XOR Operator
Before diving into the practical applications of the XOR operator, it’s essential to grasp how it works. The XOR operator compares two bits and returns true if the bits are different and false if they are the same. For instance:
- 0 XOR 0 = 0
- 0 XOR 1 = 1
- 1 XOR 0 = 1
- 1 XOR 1 = 0
This property makes the XOR operator particularly useful in various programming scenarios, such as toggling bits, checking for equality, and performing cryptographic operations. In Java, the XOR operator is used with integers, and it operates at the bit level, making it efficient for low-level data manipulation.
Using the XOR Operator for Simple Calculations
One of the most straightforward applications of the XOR operator in Java is to perform simple calculations. For example, you can use the XOR operator to find the unique number in an array where every other number appears twice. Here’s how you can achieve this:
public class UniqueNumberFinder {
public static void main(String[] args) {
int[] numbers = {2, 3, 5, 3, 2};
int unique = 0;
for (int number : numbers) {
unique ^= number;
}
System.out.println("The unique number is: " + unique);
}
}
Output:
The unique number is: 5
In this code, we initialize a variable unique
to 0. We then iterate through the numbers
array, applying the XOR operator to each element. The beauty of the XOR operation lies in its ability to cancel out duplicate numbers. Thus, after processing the entire array, the unique
variable holds the value of the number that appears only once. This method is not only efficient but also elegant, showcasing the power of the XOR operator.
Swapping Two Numbers Without a Temporary Variable
Another interesting use case for the XOR operator in Java is swapping two numbers without using a temporary variable. This technique is not only clever but also a great example of how bitwise operations can simplify your code. Here’s how it works:
public class SwapNumbers {
public static void main(String[] args) {
int a = 10;
int b = 20;
a = a ^ b;
b = a ^ b;
a = a ^ b;
System.out.println("After swapping: a = " + a + ", b = " + b);
}
}
Output:
After swapping: a = 20, b = 10
In this example, we start with two integers, a
and b
. The first line performs an XOR operation between a
and b
and stores the result in a
. The second line updates b
by XORing the new value of a
with b
. Finally, the last line updates a
again by XORing with the new value of b
. This series of operations effectively swaps the values of a
and b
without needing any extra storage. It’s a neat trick that highlights the power of the XOR operator.
Checking Even or Odd Numbers
The XOR operator can also be used to check whether a number is even or odd. This is done by examining the least significant bit (LSB) of the number. If the LSB is 0, the number is even; if it’s 1, the number is odd. Here’s how you can implement this in Java:
public class EvenOddChecker {
public static void main(String[] args) {
int number = 15;
if ((number & 1) == 1) {
System.out.println(number + " is odd.");
} else {
System.out.println(number + " is even.");
}
}
}
Output:
15 is odd.
In this code snippet, we use the bitwise AND operator (&) in conjunction with the XOR operator to determine if the number is even or odd. The expression (number & 1)
isolates the least significant bit. If the result is 1, the number is odd; if it’s 0, it’s even. This method is efficient and leverages the properties of binary numbers, making it a great example of how bitwise operations can simplify logic in your code.
Conclusion
The XOR operator in Java is a versatile tool that can simplify many programming challenges. From finding unique numbers in arrays to swapping variables and checking the parity of integers, the XOR operator offers elegant solutions to common problems. By mastering its use, you can enhance your coding efficiency and problem-solving skills. As you continue to explore Java, remember to experiment with the XOR operator in various scenarios to fully appreciate its capabilities.
FAQ
-
what does the XOR operator do in Java?
The XOR operator compares two bits and returns true if they are different and false if they are the same. -
can the XOR operator be used for swapping numbers?
Yes, the XOR operator can swap two numbers without needing a temporary variable. -
how can I find a unique number in an array using XOR?
You can iterate through the array and apply the XOR operator to each element. The result will be the unique number. -
is the XOR operator efficient in Java?
Yes, the XOR operator operates at the bit level, making it efficient for low-level data manipulation. -
can the XOR operator be used to check if a number is even or odd?
Yes, you can use the XOR operator in conjunction with the bitwise AND operator to check if a number is even or odd.