Java Bitwise Operators
- Java Bitwise Operators
-
Bitwise
OR
Operator in Java -
Bitwise
AND
Operator in Java -
Bitwise
XOR
Operator in Java - Bitwise Complement Operator in Java
- Bitwise Shift Operator in Java
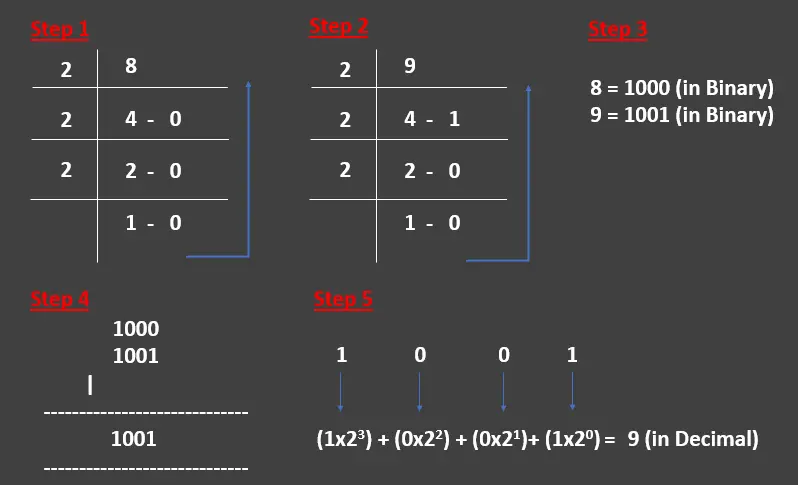
This tutorial educates about the bitwise operators in Java. We will learn about bitwise OR
, AND
, XOR
, complement, and shift operators via code examples and mathematical explanations.
Java Bitwise Operators
Considering Java programming, an operator is used to do a specified operation. Bit operators work on bits and operate individually (bit-by-bit).
The bitwise operators can be used with int
, short
, and char
. We can use bitwise operators when we perform an update or want to query operators of a binary indexed tree.
The different types of bitwise operators are listed below.
- Bitwise
OR
, symbolically represented as|
. - Bitwise
AND
, symbolically represented as&
. - Bitwise
XOR
, represented in the code as^
. - Bitwise Complement, represented with
~
. - Bitwise Shift operators:
5.1 Signed Right Shift, represented as>>
.
5.2 Signed Left Shift, represented as<<
.
5.3 Unsigned Right Shift, denoted with>>>
.
5.4 Unsigned Left Shift denoted with<<<
.
Let’s learn them one by one.
Bitwise OR
Operator in Java
The OR
is a binary operator denoted with the pipe sign |
. It is also known as inclusive OR
.
The OR
operator returns 1 if it finds at least one of the operands is 1; otherwise, 0. Following is the truth table for two operands, X
and Y
, that we can use to understand the bitwise OR
operator.
X | Y | X | Y |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
After understanding the truth table, we can easily convert it into a Java program given below.
public class BitwiseOr {
public static void main(String[] args) {
int x = 8, y = 9;
System.out.println("x | y = " + (x | y));
}
}
Output:
x | y = 9
What is happening behind this program? Here, the numbers 8 and 9 are converted to bits first, 1000 and 1001, respectively.
Their OR
is equal to 1001, which is 9. How? See the following.
Bitwise AND
Operator in Java
This binary operator is denoted by &
and returns 1 if both bits are 1; otherwise, it returns 0. The following truth table demonstrates the AND
operator where X
and Y
are two operands and accept binary values only (1 or 0).
X | Y | X & Y |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Now, we can transform this truth table into Java code.
public class BitwiseAnd {
public static void main(String[] args) {
int x = 8, y = 9;
System.out.println("x & y = " + (x & y));
}
}
Output:
x & y = 8
Following is the visual explanation of each step being performed behind the Java program given above.
Bitwise XOR
Operator in Java
The binary XOR
operator (also known as exclusive OR
) is denoted with the symbol ^
returns 1 if both bits are different. We get 1 if the operand X
is 1, and Y
is 0, or Y
is 1, and X
is 0.
We get the result as 0 if both are either 1 or 0. Don’t be confused; see the following truth table to understand it.
X | Y | X ^ Y |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
We can translate it into the Java program as follows.
public class BitwiseXor {
public static void main(String[] args) {
int x = 8, y = 9;
System.out.println("x ^ y = " + (x ^ y));
}
}
Output:
x ^ y = 1
Let’s understand how it works in the following step-by-step screenshot.
Bitwise Complement Operator in Java
This binary operator is a unary operator denoted with ~
and pronounced as a tilde. The complement operator returns the inverse by flipping every bit from 0 to 1 and 1 to 0.
We can also say that it returns the one’s complement because it makes every bit from 1 to 0 and 0 to 1. Check the following truth table.
X | ~X |
---|---|
0 | 1 |
1 | 0 |
The Java program for the upper truth table is below.
public class BitwiseComplement {
public static void main(String[] args) {
int x = 8;
System.out.println("~x= " + (~x));
}
}
Output:
~x= -9
Remember, the complement of N
is always equal to -(N+1)
. Here N
is the input value which is 8 here.
So, the complement would be -(8+1) = -9
. Now, let’s understand it via mathematical work to confirm whether we can get the correct result or not.
See, we are not able to find out the expected result. Why? Because we are not allowed to convert the answer into decimals directly.
Remember, the 2’s complement of (-9) is equivalent to the 1’s complement of 8. Hence, we can say that ~N = - (N+1)
.
Let’s prove it together in the following mathematical explanation.
Bitwise Shift Operator in Java
We use the bitwise shift operators in Java to move the bits to the right or left by a given number. Let’s practice each of them.
Signed Right Shift Operator in Java
The signed right shift is also called the bitwise right shift operator, denoted with >>
and shifts a bits’ pattern towards the right by a particular number of bits. The rightmost bits are discarded, and the leftmost position is filled with the sign bit.
This shifting makes some vacant position(s) at the leftmost filled with zeros (because we don’t have any sign bit in this example that represents it as a positive number). The mathematical explanation of this example is given below.
Let’s compare it with the output we will get by executing the following program and the formula below.
Example Code:
public class BitwiseSignedRightShift {
public static void main(String[] args) {
int x = 10;
System.out.println("10 >> 1 = " + (x >> 1));
}
}
Output:
10 >> 1 = 5
See, we are getting the output 5 in all ways, which means we are doing right.
Signed Left Shift Operator in Java
It is also known as the bitwise left shift operator, denoted by <<
, which moves a particular pattern of bits towards the left with a given number of bits and fills the vacant positions with 0.
Let’s understand how it works using 8-bit
representation before moving on to the code example.
As you can see in the explanation above, we converted the number 10 into the binary form using the 8-bit
representation. Then, discard the one left most bit, shift all the bits to the left by 1 position that makes the rightmost bit vacant, which is filled with 0.
Remember, we are not filling the vacant positions with discarded bits. We can also use the formula given below to find the answer quickly.
Let’s do it with the code example given below, and you can also confirm the output by using the formula given above.
public class BitwiseSignedLeftShift {
public static void main(String[] args) {
int x = 10;
System.out.println("10 << 1 = " + (x << 1));
}
}
Output:
10 << 1 = 20
Unsigned Right Shift Operator in Java
It shifts the bits towards the right by a given number of bits but does not take a sign bit for stuffing the trailing positions. It is denoted with the >>>
and pack the trailing positions with 0.
See the following code example.
public class BitwiseUnsignedRightShift {
public static void main(String[] args) {
int x = 40;
System.out.println("40 >>> 2 = " + (x >>> 2));
}
}
Output:
40 >>> 2 = 10
Unsigned Left Shift Operator in Java
The unsigned left shift operator (denoted by <<<
) is not supported in Java programming because the <<
and <<<
are identical operations.