Logical Operators and Short Circuit in Java
- Use of the Logical Operators in Java
- Use of the Not Operator (!) in Java
- Use of a Short Circuit in Java
- Advantages of Using Short Circuit in Java
- Disadvantages of Using Short Circuit in Java
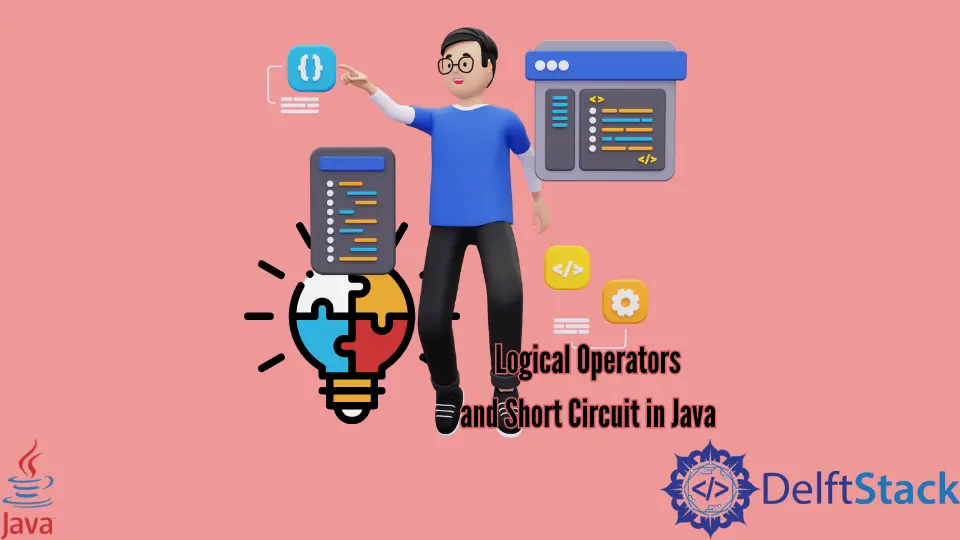
This article provides a deep look at the short circuit in java, but before short-circuiting in java, you must know what logical operators are and how to use them with various expressions.
Use of the Logical Operators in Java
Logical operators are used to checking the result of 2 or more expressions that return a Boolean value.
Logical operators are also called Boolean operators, and the multiple expressions they evaluate are known as compound expressions. These are logical AND (&&)
, logical OR (||)
, and Logical NOT (!)
.
Use of the Not Operator (!) in Java
The Not (!)
operator is the only unary logical operator. It checks a condition and returns the opposite result, meaning the operator will return true
if the condition is false
.
import java.io.*;
public class NotExample {
public static void main(String args[]) {
int big = 25, small = 10;
System.out.println("!(big < small) = " + !(big < small));
}
}
Output:
!(big < small) = true
Here, the (big < small)
returns false
. Since the Not (!)
operator is present before the condition, the answer becomes true
.
Use of a Short Circuit in Java
A short circuit in Java is the skipping action performed after evaluating logical expressions because the final result is obtained before the whole expression is evaluated.
Short-circuiting in Java can happen with:
-
Logical
AND (&&)
Operator - There are two scenarios. If the first expression returnstrue
, we will check the second one to see if it also givestrue
, returningtrue
as the final answer.However, short-circuiting occurs if the first expression returns
false
after its computation, and the second expression is not computed because the condition has already failed.
import java.io.*;
public class ANDExample {
public static void main(String arg[]) {
if (false && true && true) {
//
} else {
System.out.println("First false, condition failed, Short Circuiting!");
}
if (true && true && true) {
System.out.println("All true, no short circuiting!");
}
}
}
Output:
First false, condition failed, Short Circuiting!
All true, no short circuiting!
-
Logical OR (||) Operator - The
OR (||)
operator means that if the first or second expression returnstrue
, the condition will be met, and the code will run.However, if our first condition returns
true
, then short-circuiting occurs, and the second expression will be skipped because our condition to get true has already been satisfied.
public class ORExample {
public static void main(String arg[]) {
if (true || false || false) {
System.out.println("True found, condition met, short circuit!");
} else {
//
}
if (false || true) {
System.out.println("True found at end, condition met, no short circuit.");
}
}
}
Output:
True found, condition met, short circuit!
True found at end, condition met, no short circuit.
Short-circuiting does not occur while working with bitwise AND (&)
and Bitwise OR (|)
operators in Java.
And (&)
Operator - Checks the result of all expressions and returns true
if and only if all expressions are true
.
Or (|)
Operator - Compares the results of all expressions. If even one of the expressions is true
, the output will return ’true'.
if (false | true) {
// Both conditions evaluated
}
if (false || true) {
// Only one condition evaluated
}
Both conditions will be evaluated in the first, if statement
, but in the second if statement
, only the first one is evaluated, and the second is skipped.
Short-circuiting is only performed while working with Logical AND (&&)
and Logical OR (||)
.
Advantages of Using Short Circuit in Java
Short-circuiting in java helps avoid specific time complex tasks if the predetermined conditions are already met or failed, reducing computation time and complexity.
For instance, if you check conditions for N number of expressions, you need them to return true
. If an expression returning false
is computed at any position, say F where F«N, it can significantly reduce the execution time.
Instead of evaluating the total number of expressions, the compiler skips N-F terms.
Disadvantages of Using Short Circuit in Java
-
Bypassed Logics: In some circumstances, short-circuiting can ignore logic. Suppose we are working with the logical
OR
with two conditions. The first condition returnstrue
orfalse
, and the second condition calls another method to complete the processing of a specific part of the program.If the first condition is satisfied and short-circuiting occurs, the other function crucial to processing is not called, creating problems bypassing logic.
-
Less Efficient Execution: Short-circuiting can reduce code execution time and increase it in some cases. Some compilers involve different check cycles to verify the short circuit evaluation, adding extra computations.