Diamond Operator in Java
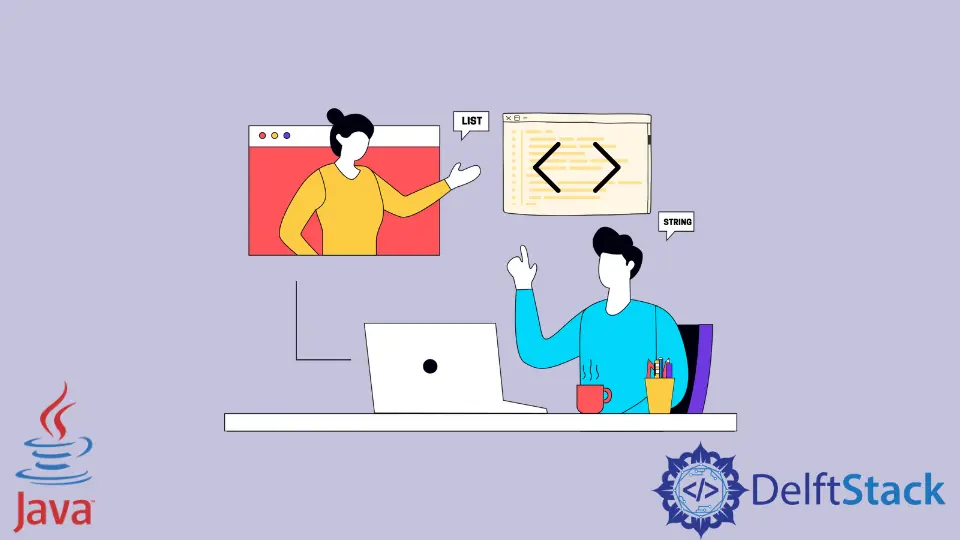
If you are looking for a more simplified use of Generics, you should look at the diamond operator of Java. The diamond operator looks like this <>
, mainly used for the Anonymous Inner Class.
This special operator in Java will help you to simplify the use of Generics when you are going to create an object. It will increase the program’s readability and allow you to avoid unchecked warnings in a program.
In this article, we will see the use of the diamond operator <>
, and we will discuss the topic with the help of examples and explanations to make the topic easier.
Diamond Operator in Java
The most interesting thing about the diamond operator is that we can create an object of the Generics without mentioning the type of the Generics. The general syntax shared below is for creating objects with the diamond operator:
ClassName<TYPE> MyObj = new ClassName<>();
Please note that you can’t use the diamond operator when running the lower version of JDK like JDK 7. So, to run the codes from this article, you must upgrade your JDK.
An Example With the Diamond Operator in Java
Our example below will demonstrate how we can use the diamond operator in Java. Let’s follow the below example code,
class UsingDiamond {
// Declaring a generic method
static <T> void DisplayMsg(T data) {
System.out.println("The input string is: " + data);
}
// Our controlling method ( Main method )
public static void main(String[] args) {
// Call a generic method with a String argument
DisplayMsg("This is a string");
}
}
We already commanded the purpose of each line. After running the example code shared above, you will get an output like the one below in your console.
The input string is: This is a string
Let’s see another example to make the topic clear to us,
In our example below, we will create an abstract class and link it with the main class using the diamond operator. Take a look at the below example:
abstract class AbstractClass<T> { // Declaring a abstract class
abstract T Sum(T num1, T num2); // Declaring a abstract method
}
// Our main class
public class UsingDiamond {
public static void main(String[] args) {
AbstractClass<Integer> obj =
new AbstractClass<>() { // Creating an object for the abstract class
Integer Sum(Integer n1, Integer n2) { // Defining the abstract method
return (n1 + n2);
}
};
Integer result = obj.Sum(30, 20); // Calling the abstract method.
System.out.println("The result is: " + result);
}
}
We already commanded the purpose of each code line. After running the example code shared above, you will get an output like the one below in your console.
The result is: 50
Please note that the code examples shared here are in Java. You must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn