Operator Overloading in Java
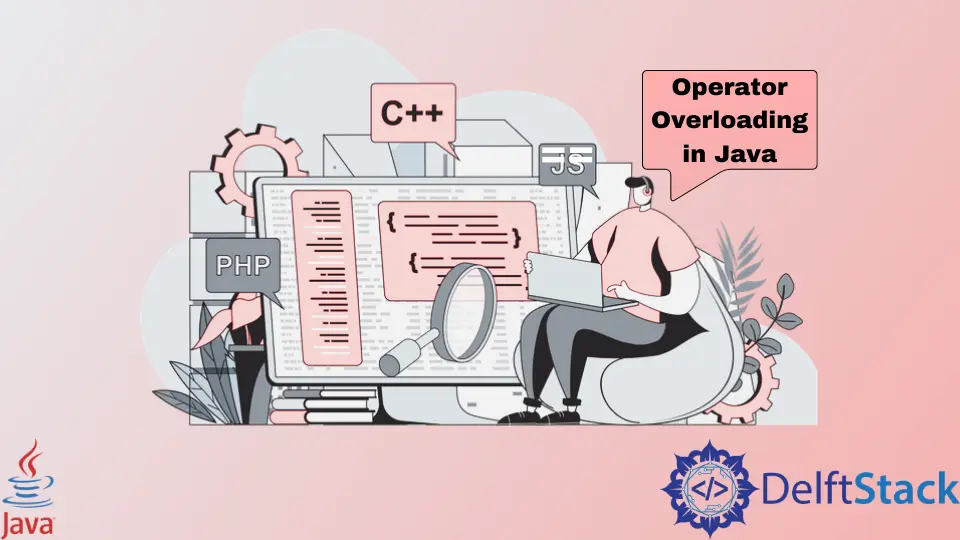
This article discusses whether Java supports user-defined operator overloading or not.
Operator Overloading in Java
Operator overloading is how operators can be implemented in user-defined types. It uses customized logic based on the type and number of arguments we pass.
Using operator overloading, we can specify user-defined operation implementation. Syntactic sugar is another term for this. The operators involved in operation can be of structure type or user-defined class.
For example, using a plus operator +
for adding two numbers and concatenating two strings is the simplest case of operator overloading.
However, Java does not support operator overloading except for one case, string concatenation using the plus operator.
Here is the complete example.
import java.util.Scanner;
public class OperatorOverloading {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Input the first string
System.out.println("Enter a string");
String s1 = sc.next();
// Input the second string
System.out.println("Enter another string: ");
String s2 = sc.next();
// Plus operator concatenates the two Strings
System.out.println(s1 + ' ' + s2);
// Input the first integer
System.out.println("Enter a number");
int x = sc.nextInt();
// Input the second integer
System.out.println("Enter another number");
int y = sc.nextInt();
// Plus operator adds the two numbers
System.out.println(x + y);
}
}
Output:
Enter a string
Hello
Enter another string:
World
Hello World
Enter a number
3
Enter another number
4
7
In this example, the plus operator adds the two integers and concatenates the two strings. It is the only operator overloading that Java supports.
Other than this, Java does not support user-defined operator overloading. The only part of Java close to operator overloading is the handling of +
for concatenating strings.
It results in the compile-time concatenation of constants or run-time concatenation. However, in Java, we cannot define our operators, which act like this.
Although we can concatenate strings in Java using the plus operator, Java has an in-built concat()
method that eliminates the need to perform operator overloading. The concat()
method appends another string at the end of a given string.
We should specify the strings to get the combined string in the order we desire.
Syntax:
String resultant = String1.concat(String2)
It will add the second string at the end of the first string. The example below uses the concat()
method to put together two strings.
import java.util.Scanner;
public class OperatorOverloading {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter a string:");
String s1 = sc.next();
System.out.println("Enter another string:");
String s2 = sc.next();
// Concatenate the two strings using concat() method
String res = s1.concat(s2);
System.out.println(res);
}
}
Output:
Enter a string:
Hello
Enter another string:
World
HelloWorld
Note that here, we are not using the plus operator for string concatenation, and we can still combine the two strings with the help of the concat()
method.
Reasons Why Java Doesn’t Support Operator Overloading
We can redefine most of the built-in operators in C++ but not in Java. It is due to the following reasons.
- Operator overloading increases code complexity. If we overload an operator in Java, the Java Virtual Machine (JVM) in Java needs to put an extra effort to find out the actual functionality of the operator used in any particular statement. It makes the processing quite complex.
- Operator overloading introduces errors in the program. Operator overloading creates confusion amongst programmers. Also, working with languages that support operator overloading has chances of error compared to other languages. Java has method overloading. Method Overloading serves the functionality of operator overloading and eliminates the scope for errors.
- Java supports method overloading. Method overloading is the concept of a class having more than a single method with the same name but different parameters. The identification of the method that is to be used is easier here. It depends on the number of arguments or the data type of the arguments.
Let us see an example.
import java.util.*;
// The class which has two methods
class DemoClass {
// This method takes two arguments
static int demo(int a, int b) {
return a + b;
}
// This method takes three arguments
static int demo(int a, int b, int c) {
return a * b * c;
}
}
class MethodOverloading {
public static void main(String[] args) {
// Passing two arguments
System.out.println(DemoClass.demo(10, 12));
// Passing three arguments
System.out.println(DemoClass.demo(11, 10, 10));
}
}
Output:
22
1100
The class DemoClass
has two methods with the same name, demo
, in the example above. But these two methods take different numbers of arguments.
When we pass two arguments, the first demo method comes into action, and when we pass three arguments, the second demo method comes into action. It is how method overloading works in Java.
To keep the language simple, the developers of Java did not provide the feature of operator overloading. Every operator has some meaning that we can change depending on the developer’s requirement.
It leads to confusion when multiple people are working on the same project. The Java designers avoided operator overloading in Java to avoid using operators. It makes Java an easy-to-implement language.
A few Java-like languages, also known as JVM-based languages, support operator overloading. These include Groovy and Kotlin.
Developed by Jetbrains, Kotlin is a high-level and statically typed language. It runs on the Java Virtual Machine or JVM and can even be compiled into Javascript source code.
One of the major advancements of Kotlin over Java is that Kotlin supports operator overloading, unlike Java.
On the other hand, Groovy supports both static and dynamic typing and compiles to Java Virtual Machine ByteCode. Groovy also supports operator overloading.
Conclusion
As an answer to whether Java supports operator overloading or not, Java does not support operator overloading. Only one part of Java resembles this concept: string concatenation using the plus operator.
Other than this, Java does not let you define your operators. However, a few other JVM-based languages, like Java, support operator overloading.
These include Groovy and Kotlin. But these are not the same as Java.