Unsigned and Signed Right Bit Shift Operator in Java
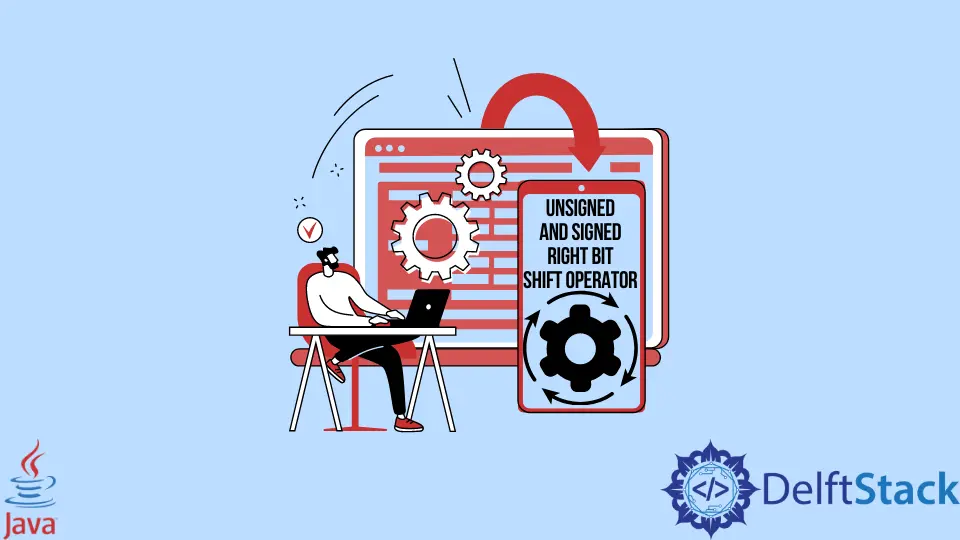
This tutorial educates about signed and unsigned right-bit shift operators in Java. It also demonstrates their use via code examples.
Unsigned and Signed Right Bit Shift Operator in Java
Unlike other programming languages, Java supports two right-bit shift operators.
- The Signed right bit-shift, represented by the symbol
>>
. - The Unsigned right bit-shift, represented by the symbol
>>>
.
Note that other programming languages like C, C++, etc. contain only the Signed right bit-shift operator (>>
), but Java allows you to use both signed and unsigned shift operations.
Difference Between Signed & Unsigned Right Bit Shift Operator
The signed right shift (>>
) shifts each bit of a number to the right and preserves the sign bit (leftmost bit). The sign bit is used to reserve the sign of the number. The number would be positive or negative if the sign bit is 0
or 1
, respectively.
On the other hand, the Unsigned right shift (>>>
) also does a similar operation as the signed right shift, but the difference is that the unsigned right shift always fills the leftmost position with the 0
as the value is not signed.
If you apply the unsigned right shift on a positive number, it will show you the same result as the signed right shift, but if you provide the negative number, the result will be positive as all the signed bit is replaced by 0
.
Example for Signed Right Bit Shift Operator (>>
):
Let's take a number -11, and we will shift 2 bits towards the right.
+11 in 8-bit form = 0000 1011
1's Complement = 1111 0100
2's Compelement = +1
-----------------------------
2's Complement of -11 = 1111 0101
n = -11 = 1111 0101
Shift 1st bit = 1111 1010
Shift 2nd bit = 1111 1101
1111 1101
-1
------------------------------
1111 1100 = 1's Complement
0000 0011 = Complement of each bit
So -11 >> 2 = -3 which is in binary 0000 0011.
Example for Unsigned Right Bit Shift Operator (>>>
):
n = 10 = 0000 1010 //the leftmost bit position is filled with '0' as 'n' is positive
Now Shift the '3' bits towards the right; you can do it directly, but let's go one-by-one
n = 0000 1010
Shift 1st bit = 0000 0101
Shift 2nd bit = 0000 0010
Shift 3rd bit = 0000 0001
So, n >>> 3 = 0000 0001 which would be 1 in decimals.
We can also use this formula:(Given decimal number/2^n)
Where 'n' is the required number of shifts, in our case, it is '3'.
So, n >>> 3 = 10/2^3 = 10/8 = 1
Remember, all shifted bits will be lost in both cases (signed and unsigned right shift).
Use of Signed Right Bit Shift (>>
) in Java
We performed a signed right bit-shift operation on a number in the example below.
Example Code:
public class SignedRightShift {
public static void main(String args[]) {
int a = -11;
System.out.println(a >> 2);
int b = 4;
System.out.println(b >> 1);
}
}
Output:
-3
2
Use of Unsigned Right Bit Shift (>>>
) in Java
The example below illustrates an unsigned right bit-shift operation on a number.
Example Code:
public class UnsignedRightShift {
public static void main(String args[]) {
// The binary representation of -1 is all "111..1".
int a = -1;
// The binary value of 'a >>> 29' is "00...0111"
System.out.println(a >>> 29);
// The binary value of 'a >>> 30' is "00...0011"
System.out.println(a >>> 30);
// The value of 'a >>> 31' is "00...0001"
System.out.println(a >>> 31);
}
}
Output:
7
3
1
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn