Java の符号なしおよび符号付き右ビット シフト演算子
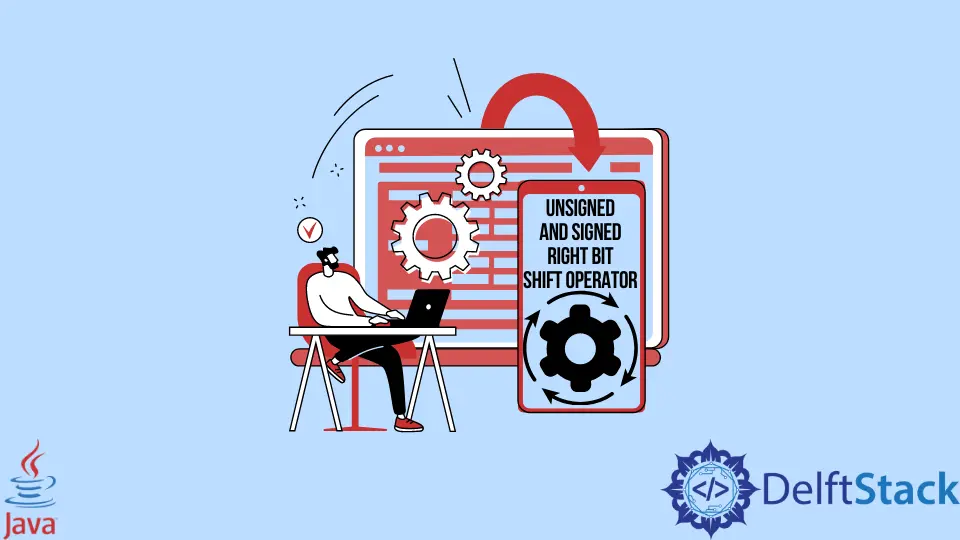
このチュートリアルでは、Java の符号付きおよび符号なしの右ビット シフト演算子について説明します。 また、コード例を介してその使用法を示します。
Java の符号なしおよび符号付き右ビット シフト演算子
他のプログラミング言語とは異なり、Java は 2つの右ビット シフト演算子をサポートしています。
- シンボル
>>
で表される符号付き右ビット シフト。 - 記号
>>>
で表される符号なし右ビット シフト。
C、C++ などの他のプログラミング言語には符号付き右ビット シフト演算子 (>>
) しか含まれていませんが、Java では符号付きシフト操作と符号なしシフト操作の両方を使用できます。
符号付きと符号なしの右ビット シフト演算子の違い
符号付き右シフト (>>
) は、数値の各ビットを右にシフトし、符号ビット (左端のビット) を保持します。 符号ビットは、数値の符号を予約するために使用されます。 符号ビットが 0
または 1
の場合、数値はそれぞれ正または負になります。
一方、符号なし右シフト (>>>
) も符号付き右シフトと同様の操作を行いますが、違いは、符号なし右シフトでは値が常に 0
で左端の位置が埋められることです。 署名されていません。
正の数に符号なし右シフトを適用すると、符号付き右シフトと同じ結果が表示されますが、負の数を指定すると、すべての符号付きビットが 0
に置き換えられるため、結果は正になります。
符号付き右ビット シフト演算子 (>>
) の例:
Let's take a number -11, and we will shift 2 bits towards the right.
+11 in 8-bit form = 0000 1011
1's Complement = 1111 0100
2's Compelement = +1
-----------------------------
2's Complement of -11 = 1111 0101
n = -11 = 1111 0101
Shift 1st bit = 1111 1010
Shift 2nd bit = 1111 1101
1111 1101
-1
------------------------------
1111 1100 = 1's Complement
0000 0011 = Complement of each bit
So -11 >> 2 = -3 which is in binary 0000 0011.
符号なし右ビット シフト演算子 (>>>
) の例:
n = 10 = 0000 1010 //the leftmost bit position is filled with '0' as 'n' is positive
Now Shift the '3' bits towards the right; you can do it directly, but let's go one-by-one
n = 0000 1010
Shift 1st bit = 0000 0101
Shift 2nd bit = 0000 0010
Shift 3rd bit = 0000 0001
So, n >>> 3 = 0000 0001 which would be 1 in decimals.
We can also use this formula:(Given decimal number/2^n)
Where 'n' is the required number of shifts, in our case, it is '3'.
So, n >>> 3 = 10/2^3 = 10/8 = 1
どちらの場合も、シフトされたすべてのビットが失われることに注意してください (符号付きおよび符号なしの右シフト)。
Java での符号付き右ビット シフト (>>
) の使用
以下の例では、数値に対して符号付き右ビット シフト操作を実行しました。
コード例:
public class SignedRightShift {
public static void main(String args[]) {
int a = -11;
System.out.println(a >> 2);
int b = 4;
System.out.println(b >> 1);
}
}
出力:
-3
2
Java での符号なし右ビット シフト (>>>
) の使用
次の例は、数値の符号なし右ビット シフト演算を示しています。
コード例:
public class UnsignedRightShift {
public static void main(String args[]) {
// The binary representation of -1 is all "111..1".
int a = -1;
// The binary value of 'a >>> 29' is "00...0111"
System.out.println(a >>> 29);
// The binary value of 'a >>> 30' is "00...0011"
System.out.println(a >>> 30);
// The value of 'a >>> 31' is "00...0001"
System.out.println(a >>> 31);
}
}
出力:
7
3
1
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn