Unhandled Exception in Java
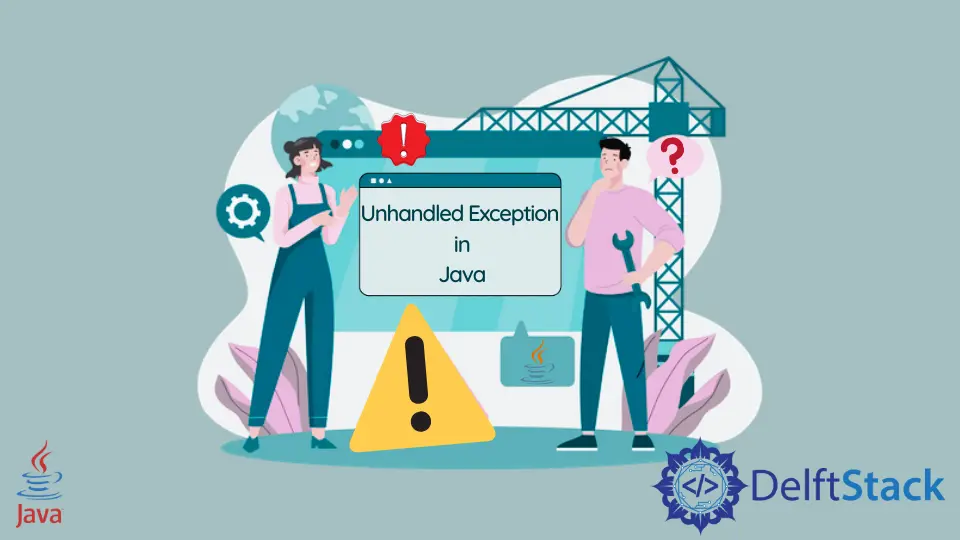
This tutorial introduces what an unhandled exception is and how to handle it in Java.
An exception is a condition that stops a code execution abnormally and leads to the termination of code.
In Java, exceptions can occur either by bad code or system failure, such as memory issues. Java provides complete support to handle the exceptions so that code can run smoothly without termination and provide the desired result.
An exception that is not handled is called an unhandled exception and leads to terminating the code before its execution.
In this article, we will discuss what an unhandled exception is and how to handle it. Let’s understand with some examples.
Unhandled Exception in Java
We will take two integer values in this example from a user and divide them. This code works fine without any error or exception, but Java does not execute the code and throws an exception if the divisor is zero.
Since we did not use any exception handler here, the code stops execution and terminates abnormally. We can see the console to understand the reason for the exception.
This exception is unhandled, and we can see how dangerous it can be if it did not handle properly.
import java.util.Scanner;
public class SimpleTesting {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter any two values");
int val1 = sc.nextInt();
int val2 = sc.nextInt();
double result = val1 / val2;
System.out.println("result " + result); // this statement does not execute
}
}
Output:
Enter any two values
30
0
Exception in thread "main" java.lang.ArithmeticException: / by zero
at myproject.SimpleTesting.main(SimpleTesting.java:13)
Handled Exception in Java
Here, we are using a handler (try-catch block) to handle the exception. This code is similar to the above code, except it has the try-catch
block and lets the code execute even after the exception.
Notice the last print statement of the code. In the above code, this statement does not execute because of an exception.
Still, it executes even after getting an exception in the below code because now the code does not terminate. Besides, it skips the code and starts executing by printing the result to the console.
See the example below.
import java.util.Scanner;
public class SimpleTesting {
public static void main(String[] args) {
double result = 0.0;
try {
Scanner sc = new Scanner(System.in);
System.out.println("Enter any two values");
int val1 = sc.nextInt();
int val2 = sc.nextInt();
result = val1 / val2;
} catch (Exception e) {
System.out.println("Exception occured " + e);
}
System.out.println("result " + result);
}
}
Output:
Enter any two values
30
0
Exception occured java.lang.ArithmeticException: / by zero
result 0.0