Java typeof Operator
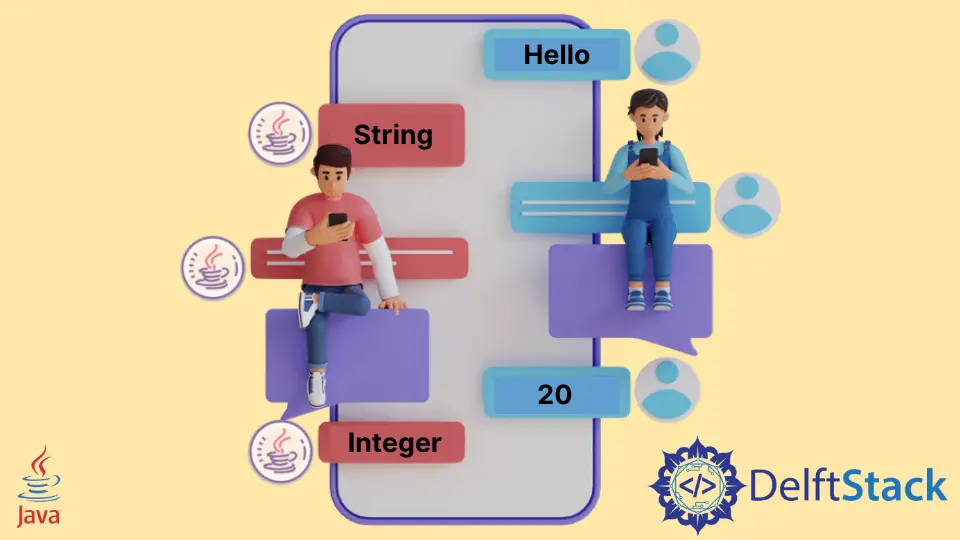
This tutorial introduces how to get the data type of a variable or value in Java and lists some example codes to understand the topic.
In Java, to get type of a variable or a value, we can use getClass()
method of Object
class. This is the only way to do this, unlike JavaScript with the typeof()
method to check type.
Since we used the getClass()
method of Object class, it works with objects only, not primitives. If you want to get the type of primitives, then first convert them using the wrapper class. Let’s understand with some examples.
Get the Type of a Variable/Value in Java
In this example, we used getClass()
to check the type of a variable. Since this variable is a string type, then we can directly call the method. See the example below.
Notice that the getClass()
method returns a fully qualified class name, including a package name such as java.lang.String
in our case.
public class SimpleTesting {
public static void main(String[] args) {
String msg = "Hello";
System.out.println(msg);
System.out.println("Type is: " + msg.getClass());
}
}
Output:
Hello
Type is: class java.lang.String
Get the Type of Any Variable/Value in Java
In the above example, we used a string variable and got its type similarly; we can also use another type of variable, and the method returns the desired result. See the example below.
In this example, we created two more variables, integer and character, apart from string and used the getClass()
method.
package javaexample;
public class SimpleTesting {
public static void main(String[] args) {
String msg = "Hello";
System.out.println(msg);
System.out.println("Type is: " + msg.getClass());
// Integer
Integer val = 20;
System.out.println(val);
System.out.println("Type is: " + val.getClass());
// Character
Character ch = 'G';
System.out.println(ch);
System.out.println("Type is: " + ch.getClass());
}
}
Output:
Hello
Type is: class java.lang.String
20
Type is: class java.lang.Integer
G
Type is: class java.lang.Character
The getClass()
method returns a complete qualified name of the class, including the package name. If you wish to get only the type name, you can use the getSimpleName()
method that returns a single string. See the example below.
package javaexample;
public class SimpleTesting {
public static void main(String[] args) {
String msg = "Hello";
System.out.println(msg);
System.out.println("Type is: " + msg.getClass().getSimpleName());
// Integer
Integer val = 20;
System.out.println(val);
System.out.println("Type is: " + val.getClass().getSimpleName());
// Character
Character ch = 'G';
System.out.println(ch);
System.out.println("Type is: " + ch.getClass().getSimpleName());
}
}
Output:
Hello
Type is: String
20
Type is: Integer
G
Type is: Character