How to Create Syllable Counter in Java
- Use a User-Defined Function to Create Syllable Counter in Java
- Use Regular Expressions to Create a Syllable Counter in Java
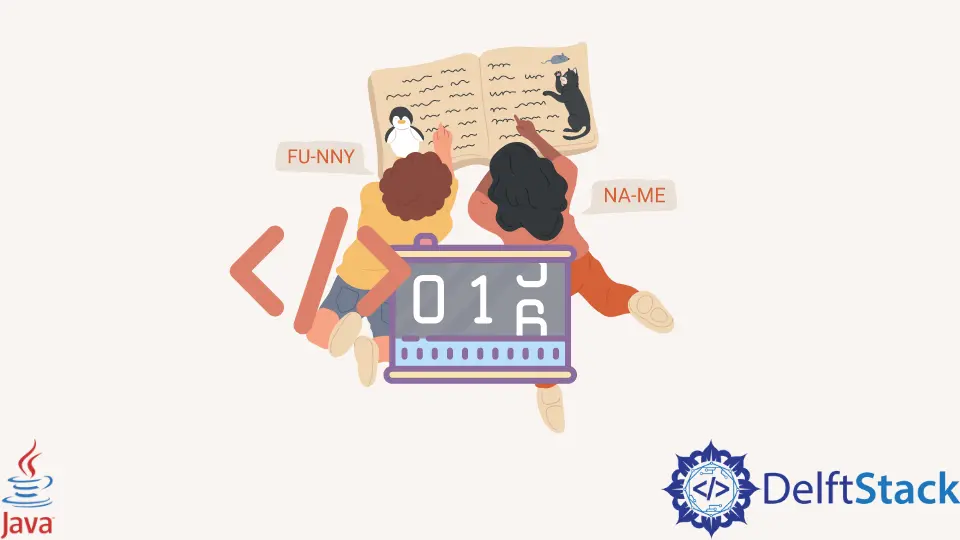
A syllable is a fundamental unit of pronunciation of any word which has a vowel sound. Specification for a syllable in this tutorial will be that each adjacent vowel counts as one syllable.
For example, in the word real
, ea
contributes to one syllable. But for the word regal
, there would be two syllables: e
and a
. However, an e
at the end of the word will not be counted as a syllable. Also, every word has at least one syllable regardless of the previously mentioned rules.
This tutorial will discuss how to create a syllable counter with the specifications mentioned above using Java.
Use a User-Defined Function to Create Syllable Counter in Java
We can create our own method SyllableCount()
that, counts the syllable based on the provided specifications. First, we use the toLowerCase()
function and convert the required string to lower case. We traverse through the string and check every character individually, whether it is a vowel or not, and the previous character.
We implement this in the following code.
import java.util.*;
public class Main {
static public int SyllableCount(String s) {
int count = 0;
s = s.toLowerCase();
for (int i = 0; i < s.length(); i++) { // traversing till length of string
if (s.charAt(i) == '\"' || s.charAt(i) == '\'' || s.charAt(i) == '-' || s.charAt(i) == ','
|| s.charAt(i) == ')' || s.charAt(i) == '(') {
// if at any point, we encounter any such expression, we substring the string from start
// till that point and further.
s = s.substring(0, i) + s.substring(i + 1, s.length());
}
}
boolean isPrevVowel = false;
for (int j = 0; j < s.length(); j++) {
if (s.contains("a") || s.contains("e") || s.contains("i") || s.contains("o")
|| s.contains("u")) {
// checking if character is a vowel and if the last letter of the word is 'e' or not
if (isVowel(s.charAt(j)) && !((s.charAt(j) == 'e') && (j == s.length() - 1))) {
if (isPrevVowel == false) {
count++;
isPrevVowel = true;
}
} else {
isPrevVowel = false;
}
} else {
count++;
break;
}
}
return count;
}
static public boolean isVowel(char c) {
if (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u') {
return true;
} else {
return false;
}
}
public static void main(String[] args) {
String ans = "Regal";
String ans1 = "Real";
System.out.println("syllables for string " + ans + " is " + SyllableCount(ans));
System.out.println("syllables for string " + ans1 + " is " + SyllableCount(ans1));
}
}
Output:
syllables for string Regal is 2
syllables for string Real is 1
In the above method, we divide up the problem, read these lines, split them into words, and then count the syllables for each word. Afterwards, we count for each line.
Use Regular Expressions to Create a Syllable Counter in Java
We can also use regular expressions. We could use the Matcher.find()
function to find the syllables using a pattern for the given string.
Remember to import the java.util.regex
package to use regular expressions.
See the code below.
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Main {
static public int countSyllables(String s) {
int counter = 0;
s = s.toLowerCase(); // converting all string to lowercase
if (s.contains("the ")) {
counter++;
}
String[] split = s.split("e!$|e[?]$|e,|e |e[),]|e$");
ArrayList<String> al = new ArrayList<String>();
Pattern tokSplitter = Pattern.compile("[aeiouy]+");
for (int i = 0; i < split.length; i++) {
String s1 = split[i];
Matcher m = tokSplitter.matcher(s1);
while (m.find()) {
al.add(m.group());
}
}
counter += al.size();
return counter;
}
public static void main(String[] args) {
String ans = "Regal";
System.out.println("syllables for string " + ans + " is " + countSyllables(ans));
}
}
Output:
syllables for string Regal is 2