String Pool in Java
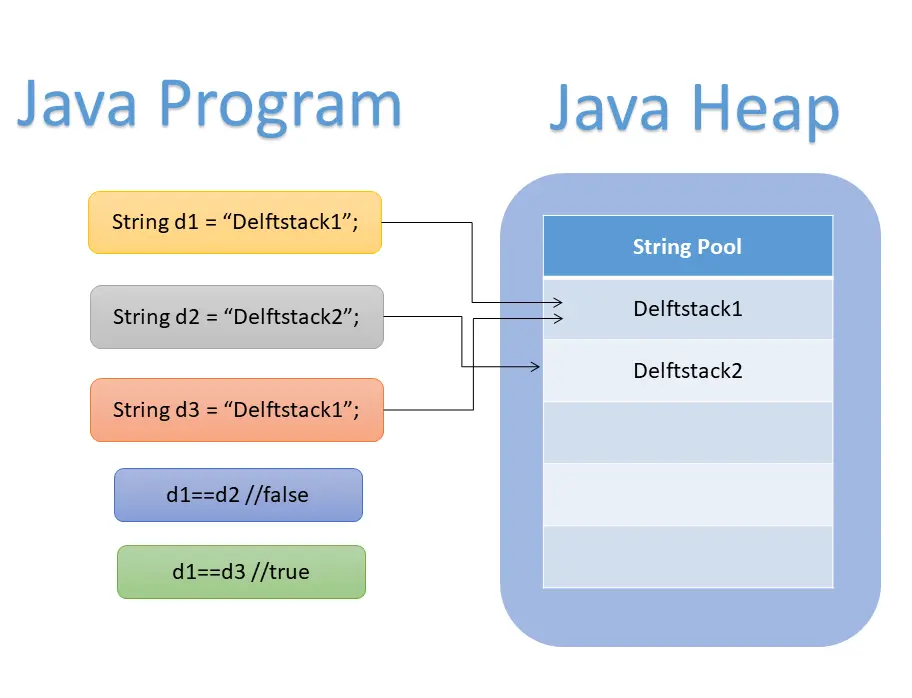
The String Pool is a storage area in the Java heap. It is a special memory region where the Java Virtual Machine stores strings.
This tutorial explains and demonstrates the string pool in Java.
String Pool in Java
The string pool is used to increase performance and decrease memory overhead.
String allocation is costly for time and memory, so the JVM performs some operations while initializing the strings to decrease both costs. These operations are performed using a string pool.
Whenever a string is initialized, the Java Virtual Machine will check the pool first, and if the initialized string already exists in the pool, it will return a reference to the pooled instance. And if the initialized string is not in the pool, a new string object will be created in the pool.
The picture below demonstrates the overview of the string pool in the Java heap.
Here is the step-by-step process of how a string pool works; let’s take the picture above as an example.
When the class is loaded, the Java Virtual Machine starts the work.
- The JVM will now look for all the string literals in the Java program.
- First, the JVM finds the string variable
d1
, which has the literalDelftstack1
; the JVM will create it in the Java heap memory. - The JVM places a reference for the literal
Delfstack1
in the string constant pool memory. - The JVM then looks for other variables; as shown in the picture above, it finds
d2
with the literalDelfstack2
andd3
with the same literal asd1
, theDelftstack1
. - Now, the strings
d1
andd3
have the same literal, so the JVM will refer them to the same object in the string pool, saving the memory for another literal.
Now let’s run the Java program, demonstrating the example described in the picture and process. See example:
package Delfstack;
public class String_Pool {
public static void main(String[] args) {
String d1 = "Delftstack1";
String d2 = "Delftstack2";
String d3 = "Delftstack1";
if (d1 == d2) {
System.out.println("Both Strings Refers to the same object in the String Pool");
} else {
System.out.println("Both Strings Refers to the different objects in the String Pool");
}
if (d1 == d3) {
System.out.println("Both Strings Refers to the same object in the String Pool");
} else {
System.out.println("Both Strings Refers to the different objects in the String Pool");
}
}
}