How to Sort a List Alphabetically in Java
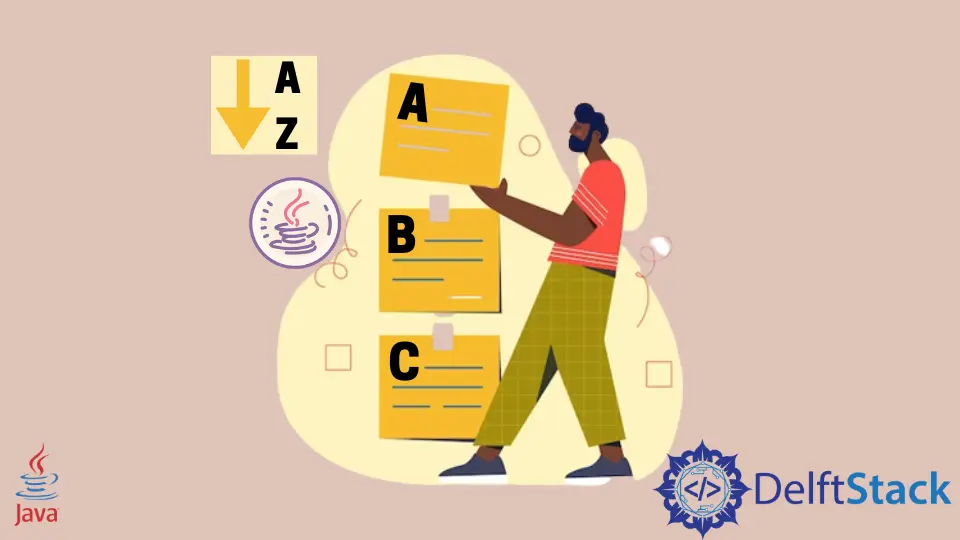
This article delves into the significance of sorting a list alphabetically in Java and explores various methods, including List.sort()
, custom comparators, Stream API, and TreeSet
, providing concise insights into their importance and application.
List
is a linear data type used to store elements of a similar type in Java. In Java, List
is an interface, and ArrayList
is its implementation class.
Importance of Sorting a List Alphabetically
Sorting a list in alphabetical order is crucial for enhanced data organization and accessibility. It allows quick and efficient retrieval of information, simplifies visual scanning, and improves overall user experience.
Alphabetically sorted lists facilitate systematic searching, making it easier for users to locate specific items or information. This organization is fundamental in various applications, from managing names in directories to presenting sorted options in dropdown menus.
Additionally, alphabetical sorting provides a standardized and predictable structure, contributing to better data readability and user understanding.
Methods of Sorting a List Alphabetically
Using the Collections.sort()
Method
Collections.sort()
simplifies alphabetical sorting in Java, offering a concise and versatile solution. It’s adaptable to diverse data types and allows customization through comparators.
The method streamlines the sorting process, enhancing code readability and maintainability compared to other methods.
Code Example:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class AlphabeticalSortExample {
public static void main(String[] args) {
// Creating a list of country names
List<String> countries = new ArrayList<>();
countries.add("India");
countries.add("China");
countries.add("Russia");
countries.add("Australia");
// Displaying the list before sorting
System.out.println("Before sorting: " + countries);
// Sorting the list alphabetically using Collections.sort()
Collections.sort(countries);
// Displaying the list after sorting
System.out.println("After sorting: " + countries);
}
}
In the code explanation, we first initialize a List<String>
called countries
using ArrayList
. Next, we add the country names to the list.
The initial order is displayed by printing the list. The crucial step involves sorting the list alphabetically using Collections.sort()
.
Finally, the sorted list is printed to observe the alphabetical order. This straightforward approach provides a clear understanding of the code’s flow, from list creation to sorting and display.
Output:
In this example, we’ve demonstrated how to use the Collections.sort()
method to alphabetically sort a list of strings in Java. Sorting is a fundamental operation that becomes crucial in scenarios where a sorted order enhances data readability and usability.
Understanding and implementing such methods is essential for any Java developer, and the Collections.sort()
method proves to be a reliable tool for achieving this task efficiently.
Using Collections.sort()
with a Custom Comparator
In scenarios where the default ordering may not suffice, a custom comparator can be employed to define a specific sorting criterion. Collections.sort()
with a custom comparator is vital for flexible alphabetical sorting in Java.
It allows tailored sorting logic, accommodating diverse data types and user-defined criteria. This method enhances code adaptability and readability, surpassing the limitations of default sorting methods.
Code Example:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
public class CustomComparatorSortExample {
public static void main(String[] args) {
// Creating a list of country names
List<String> countries = new ArrayList<>();
countries.add("India");
countries.add("China");
countries.add("Russia");
countries.add("Australia");
// Displaying the list before sorting
System.out.println("Before sorting: " + countries);
// Sorting the list alphabetically using Collections.sort() with a custom comparator
Collections.sort(countries, Comparator.naturalOrder());
// Displaying the list after sorting
System.out.println("After sorting: " + countries);
}
}
In this code explanation, we initialize a List<String>
named countries
using the ArrayList
implementation and add country names to it. The initial order is displayed by printing the list.
The crucial step involves sorting the list alphabetically using Collections.sort()
with a custom comparator, demonstrated here with Comparator.naturalOrder()
. Finally, the sorted list is printed to observe the alphabetical order.
This example emphasizes the flexibility of custom comparators, showcasing how they can be applied to achieve specific sorting criteria beyond the default ordering.
Output:
In this example, we’ve demonstrated how to use the Collections.sort()
method with a custom comparator to alphabetically sort a list of strings in Java. This approach provides flexibility when a specific ordering criterion is required.
Understanding how to utilize custom comparators enhances a developer’s ability to tailor sorting logic according to the unique requirements of their application. The output showcases the effectiveness of this method in achieving the desired alphabetical order.
Using the Stream API
Using the Stream API for sorting a list alphabetically in Java brings simplicity and conciseness to the code. It provides a functional and expressive approach, reducing the need for boilerplate code.
The streamlined syntax enhances readability and allows for easy integration with other stream operations. This method is particularly advantageous when working with complex data manipulations, offering a more elegant solution compared to traditional approaches.
Code Example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamApiSortExample {
public static void main(String[] args) {
// Creating a list of country names
List<String> countries = Arrays.asList("India", "China", "Russia", "Australia");
// Displaying the list before sorting
System.out.println("Before sorting: " + countries);
// Sorting the list alphabetically using Stream API
List<String> sortedCountries = countries.stream().sorted().collect(Collectors.toList());
// Displaying the list after sorting
System.out.println("After sorting: " + sortedCountries);
}
}
In this code explanation, we begin by initializing a List<String>
called countries
using Arrays.asList()
. The initial order is displayed by printing the list.
The core step involves sorting the list alphabetically using the Stream API. This is achieved by calling the stream()
method on the list, followed by the sorted()
operation.
The sorted elements are then collected back into a list using the collect()
method. The final step is to print the sorted list, providing a concise and expressive way to observe the alphabetical order.
Output:
In this example, we’ve demonstrated how to use the Stream API to alphabetically sort a list of strings in Java. The Stream API simplifies the code and makes it more expressive, particularly for developers familiar with functional programming concepts.
The output showcases the effectiveness of the Stream API in achieving the desired alphabetical order, providing a powerful and concise alternative to traditional sorting methods.
Using the List.sort()
Method
Using List.sort()
for alphabetical sorting in Java simplifies the sorting process, offering a direct and efficient method. This approach is concise, readable, and integrated directly into the List
interface, reducing the need for external utility classes.
It enhances code clarity, making it a preferred choice for straightforward alphabetical sorting tasks, contributing to better maintainability and ease of understanding in Java programs.
Code Example:
import java.util.ArrayList;
import java.util.List;
public class ListSortExample {
public static void main(String[] args) {
// Creating a list of country names
List<String> countries = new ArrayList<>();
countries.add("India");
countries.add("China");
countries.add("Russia");
countries.add("Australia");
// Displaying the list before sorting
System.out.println("Before sorting: " + countries);
// Sorting the list alphabetically using List.sort() method
countries.sort(String::compareToIgnoreCase);
// Displaying the list after sorting
System.out.println("After sorting: " + countries);
}
}
In the code explanation, we initiate a List<String>
named countries
using ArrayList
and add country names to it. The initial order is displayed to provide insight into the original sequence.
The crux of the process involves using the List.sort()
method directly on the list. This method facilitates alphabetical sorting, and a comparator is utilized for case-insensitive comparison.
Finally, the sorted list is printed, allowing easy observation of the new alphabetical order. This approach emphasizes simplicity and readability, making it a preferred method for straightforward alphabetical sorting in Java.
Output:
This example illustrates the simplicity and efficiency of using the List.sort()
method to alphabetically sort a list of strings in Java. The output demonstrates the effectiveness of this method in achieving the desired alphabetical order.
The compareToIgnoreCase
method used as a comparator ensures a case-insensitive comparison, making it versatile for a wide range of scenarios. Incorporating the List.sort()
method in your Java applications provides a concise and readable solution for sorting lists.
Using the TreeSet
Method
Using TreeSet
for alphabetical sorting in Java is crucial due to its automatic sorting and uniqueness features. It simplifies the sorting process, eliminating the need for explicit sorting code.
Additionally, TreeSet
ensures that elements are arranged in their natural order, providing a straightforward solution for tasks where alphabetical order and uniqueness are essential. This method enhances code simplicity, reducing the need for complex sorting logic and contributing to more efficient and readable Java programs.
Code Example:
import java.util.Set;
import java.util.TreeSet;
public class TreeSetSortExample {
public static void main(String[] args) {
// Creating a TreeSet to store country names
Set<String> countries = new TreeSet<>();
countries.add("India");
countries.add("China");
countries.add("Russia");
countries.add("Australia");
// Displaying the set before sorting
System.out.println("Before sorting: " + countries);
// TreeSet automatically maintains alphabetical order
// No explicit sorting code needed
// Displaying the set after sorting
System.out.println("After sorting: " + countries);
}
}
In the code explanation, we initialize a TreeSet<String>
called countries
for automatic sorting and uniqueness. Country names are added to the TreeSet
, and the initial order is displayed.
Unlike other sorting methods, TreeSet
requires no explicit sorting code; it automatically maintains alphabetical order. The set is then printed after sorting, showcasing the new alphabetical order with the automatic removal of duplicates.
This emphasizes the simplicity and effectiveness of using TreeSet
for tasks where both sorting and uniqueness are crucial in Java.
Output:
The use of TreeSet
provides an elegant and concise solution for sorting and ensuring uniqueness in a collection. In this example, the TreeSet
automatically maintains alphabetical order without requiring explicit sorting code.
The output demonstrates the effectiveness of using TreeSet
for sorting, making it a valuable choice when both sorting and uniqueness are essential. Incorporating TreeSet
into your Java applications simplifies the process of managing ordered and distinct collections.
Conclusion
Alphabetically sorting a list is essential for better organization and accessibility of data. This article has explored various methods to achieve alphabetical sorting in Java, each with its advantages.
From the straightforward List.sort()
method to the flexibility of custom comparators and the automatic ordering of TreeSet
, developers have versatile options to suit different needs. Whether using the concise Stream API or the simplicity of Collections.sort()
, the importance lies in creating readable, maintainable code that ensures data is presented in a clear and ordered fashion.